Degradation Modelling
This rover shows how degradation modelling can be performed to model the resilience of an engineered system over its entire lifecycle.
[1]:
import fmdtools.analyze as an
import fmdtools.sim.propagate as prop
import numpy as np
import matplotlib.pyplot as plt
import multiprocessing as mp
[2]:
from examples.rover.rover_degradation import DriveDegradation, PSFDegradationLong, PSFDegradationShort
Degradation models are defined independently of the fault model, but have attributes (e.g., functions) which may correspond to it directly.
Because degradation may only occur in specific functions/flows (and may not have inter-functional dependencies), it is not necessary for the degradation model to have the same
[3]:
deg_mdl = DriveDegradation()
deg_mdl
[3]:
drivedegradation DriveDegradation
- DriveDegradationStates(wear=0.0, corrosion=0.0, friction=0.0, drift=0.0)
[4]:
deg_mdl_hum_long = PSFDegradationLong()
deg_mdl_hum_long
[4]:
psfdegradationlong PSFDegradationLong
- PSFDegradationLongStates(experience=0.0)
[5]:
deg_mdl_hum_short = PSFDegradationShort()
deg_mdl_hum_short
[5]:
psfdegradationshort PSFDegradationShort
- PSFDegradationShortStates(fatigue=0.0, stress=0.0, experience=1.0)
[6]:
from examples.rover.rover_model import Rover, plot_map
fault_mdl = Rover(p={'ground':{'linetype': 'turn'}})
graph = fault_mdl.as_modelgraph()
fig, ax = graph.draw()
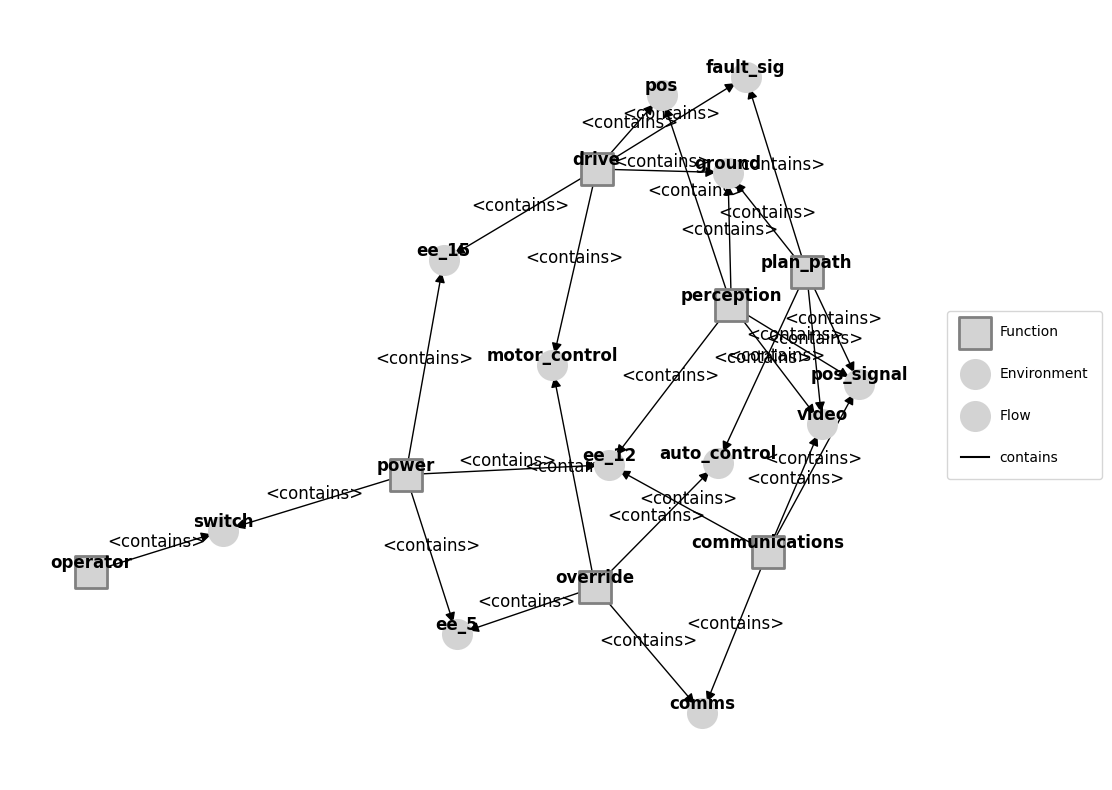
[7]:
fig.savefig("func_model.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[8]:
endresults, mdlhist = prop.nominal(fault_mdl)
fig, ax = plot_map(fault_mdl, mdlhist)
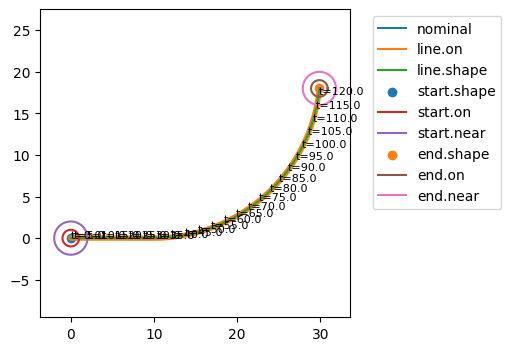
[9]:
fig.savefig("sine_rover_environment.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
As shown, there are two degradation models here: - one which focusses solely on faults in the drive system, and - one which focusses on the human degradation of fatigue Below we simulate these to model to the degradation behaviors being modelled in this drive system.
Drive Degradation
[10]:
deg_mdl = DriveDegradation()
endresults, mdlhist = prop.nominal(deg_mdl)
fig, ax = mdlhist.plot_line('s.wear', 's.corrosion', 's.friction', 's.drift', 'r.s.corrode_rate', 'r.s.wear_rate', 'r.s.yaw_load')
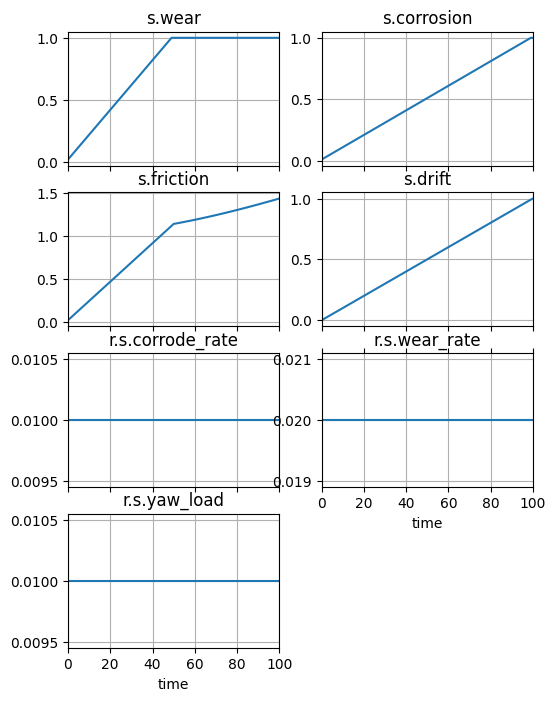
The major behaviors are: - wear - corrosion - friction - drift
These behaviors result from the accumulation of the following rates over each time-step: - yaw_load - corrode_rate - wear_rate
These degradation behaviors have additionally been defined to simulate stochastically if desired:
[11]:
deg_mdl = DriveDegradation()
endresults, mdlhist = prop.nominal(deg_mdl, run_stochastic=True)
fig, ax = mdlhist.plot_line('s.wear', 's.corrosion', 's.friction', 's.drift', 'r.s.corrode_rate', 'r.s.wear_rate', 'r.s.yaw_load')
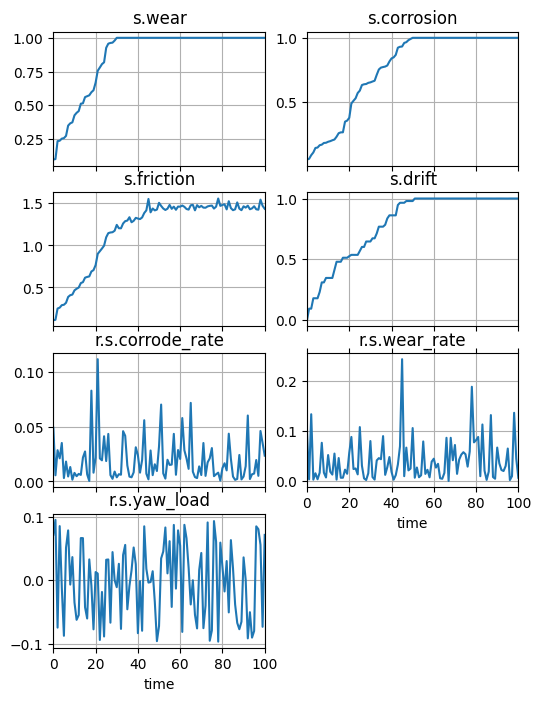
To get averages/percentages over a number of scenarios, we can view these behaviors over a given number of random seeds:
[12]:
from fmdtools.sim.sample import ParameterSample
ps = ParameterSample()
ps.add_variable_replicates([], replicates=100, seed_comb='independent')
endclasses_deg, mdlhists_deg = prop.parameter_sample(deg_mdl, ps, run_stochastic=True)
SCENARIOS COMPLETE: 100%|██████████| 100/100 [00:01<00:00, 84.54it/s]
[13]:
fig, ax = mdlhists_deg.plot_line('s.wear', 's.corrosion', 's.friction', 's.drift',
'r.s.corrode_rate', 'r.s.wear_rate', 'r.s.yaw_load',
title="", xlabel='lifecycle time (months)', aggregation = 'mean_bound')
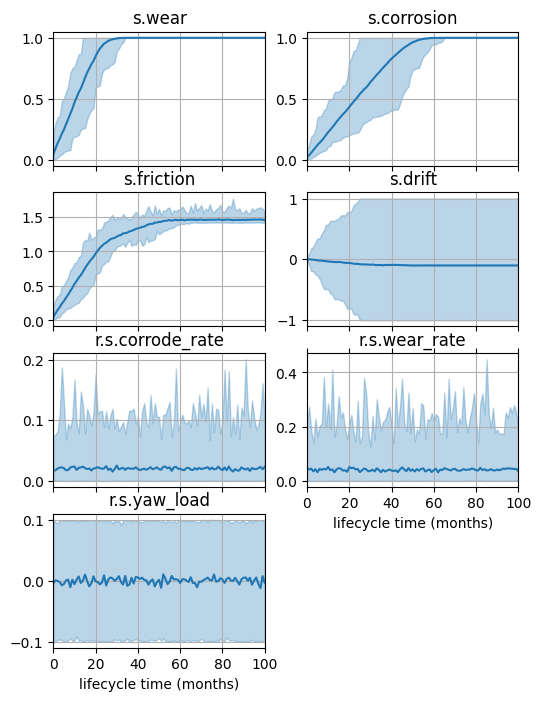
[14]:
fig.savefig("drive_degradations.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
As shown, while wear and friction proceed monotonically, drift can go one way or another, meaning that whether the rover drifts left or right is basically up to chance. We can further look at slices of these distributions:
[15]:
fig, axs = mdlhists_deg.plot_metric_dist([1, 10, 20, 25], 's.wear', 's.corrosion', 's.friction', 's.drift', bins=10, alpha=0.5)
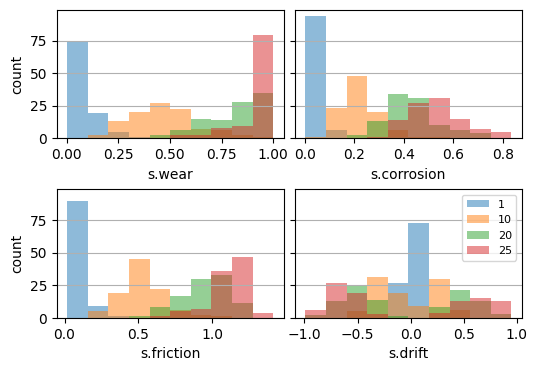
[16]:
from IPython.display import HTML
ani = mdlhists_deg.animate('plot_metric_dist_from',
plot_values=( 's.wear', 's.corrosion', 's.friction', 's.drift'),
bins=10, alpha=0.5)
HTML(ani.to_jshtml())
[16]:
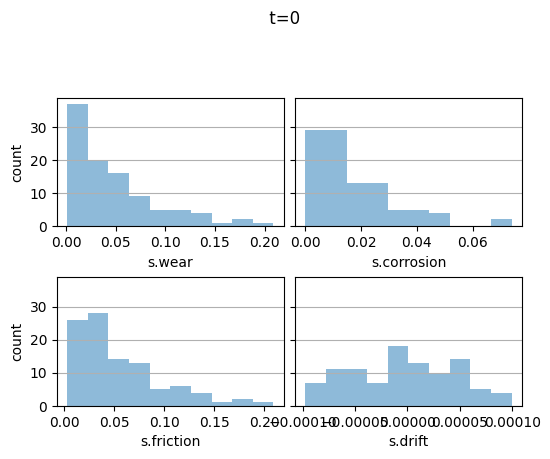
Given the parameter information (friction and drift) that the degradation model produced, we can now simulate the model with this information over time in the nominal scenarios.
[17]:
from fmdtools.sim.sample import ParameterDomain, ParameterHistSample
from examples.rover.rover_model import RoverParam
We can do this using a ParameterHistSample
to sample the histories of the various scenarios at different times.
First, by defining a ParameterDomain
:
[18]:
rpd = ParameterDomain(RoverParam)
rpd.add_variables('degradation.friction', 'degradation.drift')
rpd.add_constant('drive_modes', {"mode_args": "degradation"})
rpd(1, 10).degradation
[18]:
DegParam(friction=1.0, drift=10.0)
And then by defining the class:
[19]:
phs = ParameterHistSample(mdlhists_deg, 's.friction', 's.drift', paramdomain=rpd)
phs._get_repname('default', 1)
phs.add_hist_groups(reps= 10, ts = [1, 2, 5, 10])
len(phs.scenarios())
[19]:
40
Simulating the nominal scenario for these parameters:
[20]:
mdl=Rover(p={'drive_modes': {'mode_args': "degradation"}})
behave_endclasses, behave_mdlhists = prop.parameter_sample(fault_mdl, phs)
SCENARIOS COMPLETE: 100%|██████████| 40/40 [00:07<00:00, 5.03it/s]
[21]:
fig, ax = plot_map(fault_mdl, behave_mdlhists)
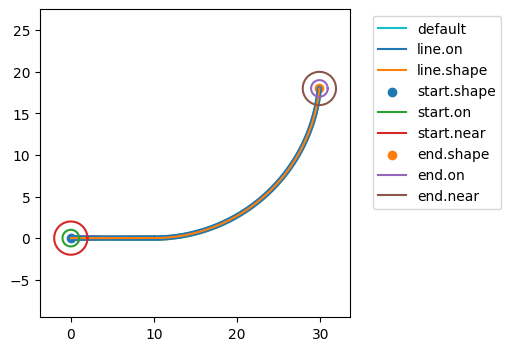
[22]:
behave_endclasses.state_probabilities('prob')
[22]:
{'nominal mission': 13.0, 'incomplete mission': 27.0}
[23]:
from fmdtools.analyze.tabulate import NominalEnvelope
[24]:
ne = NominalEnvelope(phs, behave_endclasses, 'classification',
'p.degradation.friction', 'p.degradation.drift',
func = lambda x: x == 'nominal mission')
ne.plot_scatter()
[24]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='p.degradation.friction', ylabel='p.degradation.drift'>)
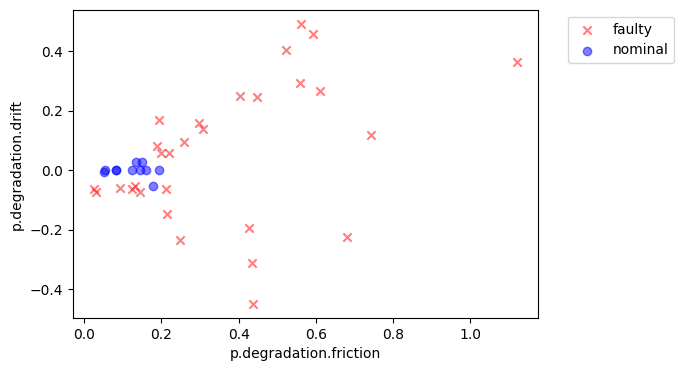
[25]:
ne = NominalEnvelope(phs, behave_endclasses, 'classification',
'inputparams.t', 'inputparams.rep',
func = lambda x: x == 'nominal mission')
ne.plot_scatter()
[25]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='inputparams.t', ylabel='inputparams.rep'>)
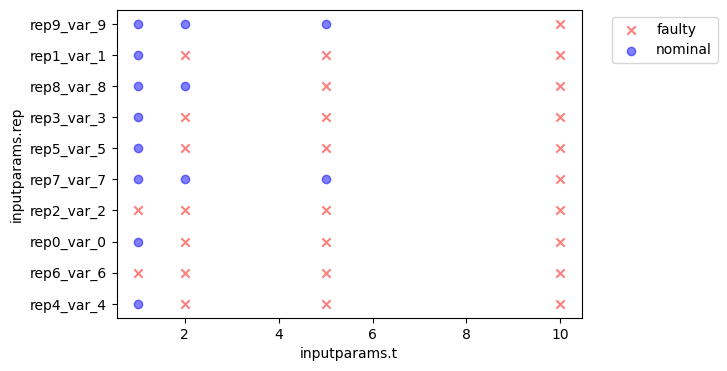
[26]:
fig.savefig("drive_deg_envelope.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
As shown, as the time (and thus degradation) increases, the rover becomes more likely to be unable to complete the mission. In this case, it results in the rover not completing the mission in time.
[27]:
from fmdtools.sim.sample import SampleApproach
sa = SampleApproach(mdl)
sa.add_faultdomain('drive_faults', 'all_fxnclass_modes', 'Drive')
sa.add_faultsample('drive_faults', 'fault_phases', 'drive_faults',"start")
# sa.scenarios()
[28]:
sa.scenarios()
[28]:
[SingleFaultScenario(sequence={15.0: Injection(faults={'drive': ['elec_open']}, disturbances={})}, times=(15.0,), function='drive', fault='elec_open', rate=0.25, name='drive_elec_open_t15p0', time=15.0, phase='start'),
SingleFaultScenario(sequence={15.0: Injection(faults={'drive': ['stuck']}, disturbances={})}, times=(15.0,), function='drive', fault='stuck', rate=0.25, name='drive_stuck_t15p0', time=15.0, phase='start'),
SingleFaultScenario(sequence={15.0: Injection(faults={'drive': ['stuck_right']}, disturbances={})}, times=(15.0,), function='drive', fault='stuck_right', rate=0.25, name='drive_stuck_right_t15p0', time=15.0, phase='start'),
SingleFaultScenario(sequence={15.0: Injection(faults={'drive': ['stuck_left']}, disturbances={})}, times=(15.0,), function='drive', fault='stuck_left', rate=0.25, name='drive_stuck_left_t15p0', time=15.0, phase='start')]
[29]:
ec_nest, hist_nest, app_nest = prop.nested_sample(mdl, phs, faultdomains={'drive_faults': (('all_fxnclass_modes', 'Drive'), {})},
faultsamples={'drive_faults': (('fault_phases', 'drive_faults', "start"), {})},
pool=mp.Pool(5))
NESTED SCENARIOS COMPLETE: 100%|██████████| 40/40 [00:17<00:00, 2.30it/s]
Finally, we can also visualize simulate and then view the effect of the degradation on average resilience…
[30]:
from fmdtools.analyze.tabulate import NestedComparison
nc = NestedComparison(ec_nest, phs, ['inputparams.t'], app_nest, ['fault'], metrics=['tot_deviation', 'end_dist'], default_stat=np.mean, ci_metrics=['end_dist', 'tot_deviation'])
[31]:
nc
[31]:
{'tot_deviation': {(1, 'stuck_right'): 0.21282445796258412, (1, 'elec_open'): 0.18784367224900939, (1, 'stuck'): 0.18784367224900939, (1, 'stuck_left'): 0.23240966184702705, (2, 'stuck_right'): 0.2178962518646644, (2, 'elec_open'): 0.20182101031500732, (2, 'stuck'): 0.20182101031500732, (2, 'stuck_left'): 0.2195858138306958, (10, 'stuck_right'): 0.23473611298070812, (10, 'elec_open'): 0.23473611298070812, (10, 'stuck'): 0.23473611298070812, (10, 'stuck_left'): 0.23473611298070812, (5, 'stuck_right'): 0.22501408859633654, (5, 'elec_open'): 0.221795401606049, (5, 'stuck'): 0.221795401606049, (5, 'stuck_left'): 0.22838035686339012}, 'end_dist': {(1, 'stuck_right'): 25.77330569442551, (1, 'elec_open'): 26.758057732490364, (1, 'stuck'): 26.706699830473305, (1, 'stuck_left'): 26.071342750930278, (2, 'stuck_right'): 26.22205412866822, (2, 'elec_open'): 27.392598112073596, (2, 'stuck'): 27.361026105890183, (2, 'stuck_left'): 27.052549312019742, (10, 'stuck_right'): 28.42947939465619, (10, 'elec_open'): 28.42947939465619, (10, 'stuck'): 28.42947939465619, (10, 'stuck_left'): 28.42947939465619, (5, 'stuck_right'): 27.636415495981502, (5, 'elec_open'): 28.03402882330746, (5, 'stuck'): 28.023304669774205, (5, 'stuck_left'): 27.921641032638348}, 'end_dist_lb': {(1, 'stuck_right'): 24.223298541855037, (1, 'elec_open'): 26.251004802354146, (1, 'stuck'): 26.16668324661208, (1, 'stuck_left'): 25.145073242706243, (2, 'stuck_right'): 24.2639423414734, (2, 'elec_open'): 26.711282624745337, (2, 'stuck'): 26.618894786278666, (2, 'stuck_left'): 25.991083189852453, (10, 'stuck_right'): 28.34021489117843, (10, 'elec_open'): 28.343215438740483, (10, 'stuck'): 28.3421127243937, (10, 'stuck_left'): 28.343028886017855, (5, 'stuck_right'): 25.1218330815329, (5, 'elec_open'): 27.253859756181217, (5, 'stuck'): 27.133239953020087, (5, 'stuck_left'): 26.613370299465085}, 'tot_deviation_lb': {(1, 'stuck_right'): 0.20814798213083172, (1, 'elec_open'): 0.15981498046706125, (1, 'stuck'): 0.15974792647226083, (1, 'stuck_left'): 0.21919554631257807, (2, 'stuck_right'): 0.21051528998312558, (2, 'elec_open'): 0.18045310724820787, (2, 'stuck'): 0.18025921003297296, (2, 'stuck_left'): 0.2105667553218262, (10, 'stuck_right'): 0.22429988906470905, (10, 'elec_open'): 0.22456142961848038, (10, 'stuck'): 0.22440723772916843, (10, 'stuck_left'): 0.22448895175223837, (5, 'stuck_right'): 0.21656122028541314, (5, 'elec_open'): 0.2107521155954482, (5, 'stuck'): 0.21034441740792031, (5, 'stuck_left'): 0.2183240163696882}, 'end_dist_ub': {(1, 'stuck_right'): 26.969249461818514, (1, 'elec_open'): 27.50250814607757, (1, 'stuck'): 27.50093587326163, (1, 'stuck_left'): 27.13444703039939, (2, 'stuck_right'): 27.589525923538428, (2, 'elec_open'): 27.976271604653753, (2, 'stuck'): 27.97487092582764, (2, 'stuck_left'): 27.88009019332042, (10, 'stuck_right'): 28.50775743731945, (10, 'elec_open'): 28.508753166606855, (10, 'stuck'): 28.507566388687156, (10, 'stuck_left'): 28.50827578676641, (5, 'stuck_right'): 28.345416063494287, (5, 'elec_open'): 28.34799476702721, (5, 'stuck'): 28.345632558608763, (5, 'stuck_left'): 28.343439358661996}, 'tot_deviation_ub': {(1, 'stuck_right'): 0.21696514655599564, (1, 'elec_open'): 0.20348031106166423, (1, 'stuck'): 0.2033036840363754, (1, 'stuck_left'): 0.24917277184211453, (2, 'stuck_right'): 0.2251786767185803, (2, 'elec_open'): 0.21441145084345895, (2, 'stuck'): 0.21421406209231245, (2, 'stuck_left'): 0.22956921296485552, (10, 'stuck_right'): 0.2511766633654025, (10, 'elec_open'): 0.25184030273731123, (10, 'stuck'): 0.2510341414486199, (10, 'stuck_left'): 0.25106713541199266, (5, 'stuck_right'): 0.23320395047163242, (5, 'elec_open'): 0.23156396248136826, (5, 'stuck'): 0.23163301902836095, (5, 'stuck_left'): 0.23840938076236962}}
[32]:
nc.sort_by_factor("fault")
nc.sort_by_factor("inputparams.t")
fig, ax = nc.as_plots('end_dist', 'tot_deviation', figsize=(8,4))
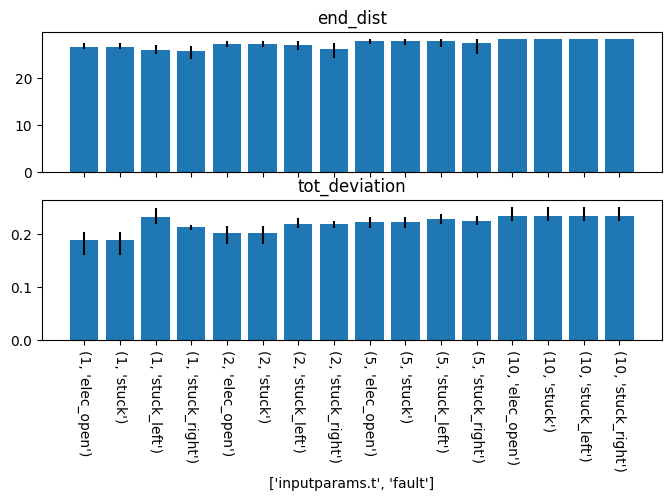
[33]:
nc
[33]:
{'tot_deviation': {(1, 'elec_open'): 0.18784367224900939, (1, 'stuck'): 0.18784367224900939, (1, 'stuck_left'): 0.23240966184702705, (1, 'stuck_right'): 0.21282445796258412, (2, 'elec_open'): 0.20182101031500732, (2, 'stuck'): 0.20182101031500732, (2, 'stuck_left'): 0.2195858138306958, (2, 'stuck_right'): 0.2178962518646644, (5, 'elec_open'): 0.221795401606049, (5, 'stuck'): 0.221795401606049, (5, 'stuck_left'): 0.22838035686339012, (5, 'stuck_right'): 0.22501408859633654, (10, 'elec_open'): 0.23473611298070812, (10, 'stuck'): 0.23473611298070812, (10, 'stuck_left'): 0.23473611298070812, (10, 'stuck_right'): 0.23473611298070812}, 'end_dist': {(1, 'elec_open'): 26.758057732490364, (1, 'stuck'): 26.706699830473305, (1, 'stuck_left'): 26.071342750930278, (1, 'stuck_right'): 25.77330569442551, (2, 'elec_open'): 27.392598112073596, (2, 'stuck'): 27.361026105890183, (2, 'stuck_left'): 27.052549312019742, (2, 'stuck_right'): 26.22205412866822, (5, 'elec_open'): 28.03402882330746, (5, 'stuck'): 28.023304669774205, (5, 'stuck_left'): 27.921641032638348, (5, 'stuck_right'): 27.636415495981502, (10, 'elec_open'): 28.42947939465619, (10, 'stuck'): 28.42947939465619, (10, 'stuck_left'): 28.42947939465619, (10, 'stuck_right'): 28.42947939465619}, 'end_dist_lb': {(1, 'elec_open'): 26.251004802354146, (1, 'stuck'): 26.16668324661208, (1, 'stuck_left'): 25.145073242706243, (1, 'stuck_right'): 24.223298541855037, (2, 'elec_open'): 26.711282624745337, (2, 'stuck'): 26.618894786278666, (2, 'stuck_left'): 25.991083189852453, (2, 'stuck_right'): 24.2639423414734, (5, 'elec_open'): 27.253859756181217, (5, 'stuck'): 27.133239953020087, (5, 'stuck_left'): 26.613370299465085, (5, 'stuck_right'): 25.1218330815329, (10, 'elec_open'): 28.343215438740483, (10, 'stuck'): 28.3421127243937, (10, 'stuck_left'): 28.343028886017855, (10, 'stuck_right'): 28.34021489117843}, 'tot_deviation_lb': {(1, 'elec_open'): 0.15981498046706125, (1, 'stuck'): 0.15974792647226083, (1, 'stuck_left'): 0.21919554631257807, (1, 'stuck_right'): 0.20814798213083172, (2, 'elec_open'): 0.18045310724820787, (2, 'stuck'): 0.18025921003297296, (2, 'stuck_left'): 0.2105667553218262, (2, 'stuck_right'): 0.21051528998312558, (5, 'elec_open'): 0.2107521155954482, (5, 'stuck'): 0.21034441740792031, (5, 'stuck_left'): 0.2183240163696882, (5, 'stuck_right'): 0.21656122028541314, (10, 'elec_open'): 0.22456142961848038, (10, 'stuck'): 0.22440723772916843, (10, 'stuck_left'): 0.22448895175223837, (10, 'stuck_right'): 0.22429988906470905}, 'end_dist_ub': {(1, 'elec_open'): 27.50250814607757, (1, 'stuck'): 27.50093587326163, (1, 'stuck_left'): 27.13444703039939, (1, 'stuck_right'): 26.969249461818514, (2, 'elec_open'): 27.976271604653753, (2, 'stuck'): 27.97487092582764, (2, 'stuck_left'): 27.88009019332042, (2, 'stuck_right'): 27.589525923538428, (5, 'elec_open'): 28.34799476702721, (5, 'stuck'): 28.345632558608763, (5, 'stuck_left'): 28.343439358661996, (5, 'stuck_right'): 28.345416063494287, (10, 'elec_open'): 28.508753166606855, (10, 'stuck'): 28.507566388687156, (10, 'stuck_left'): 28.50827578676641, (10, 'stuck_right'): 28.50775743731945}, 'tot_deviation_ub': {(1, 'elec_open'): 0.20348031106166423, (1, 'stuck'): 0.2033036840363754, (1, 'stuck_left'): 0.24917277184211453, (1, 'stuck_right'): 0.21696514655599564, (2, 'elec_open'): 0.21441145084345895, (2, 'stuck'): 0.21421406209231245, (2, 'stuck_left'): 0.22956921296485552, (2, 'stuck_right'): 0.2251786767185803, (5, 'elec_open'): 0.23156396248136826, (5, 'stuck'): 0.23163301902836095, (5, 'stuck_left'): 0.23840938076236962, (5, 'stuck_right'): 0.23320395047163242, (10, 'elec_open'): 0.25184030273731123, (10, 'stuck'): 0.2510341414486199, (10, 'stuck_left'): 0.25106713541199266, (10, 'stuck_right'): 0.2511766633654025}}
[34]:
# fig.savefig("drive_resilience_degradation.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
As shown, while there is some resilience early in the lifecycle (resulting in a small proportion of faults being recovered), this resilience goes away with degradation.
Human Degradation
We can also perform this assessment for the human error model, which is split up into two parts: - long term “degradation” of experience over months - short term “degradation” of stress and fatigue over a day
[35]:
psf_long = PSFDegradationLong()
endresults, hist_psf_long = prop.nominal(psf_long)
[36]:
hist_psf_long.plot_line('s.experience')
[36]:
(<Figure size 300x200 with 1 Axes>,
[<Axes: title={'center': 's.experience'}, xlabel='time'>])
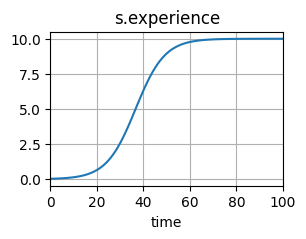
[37]:
hist_psf_long.plot_line('s.experience')
[37]:
(<Figure size 300x200 with 1 Axes>,
[<Axes: title={'center': 's.experience'}, xlabel='time'>])
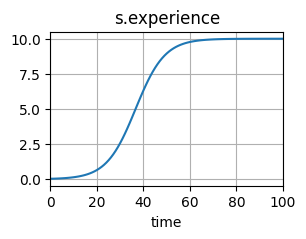
[38]:
from examples.rover.rover_degradation import LongParams
pd_hl = ParameterDomain(LongParams)
pd_hl.add_variable("experience_param", var_lim=())
pd_hl
[38]:
ParameterDomain with:
- variables: {'experience_param': ()}
- constants: {}
- parameter_initializer: LongParams
[39]:
pd_hl(10)
[39]:
LongParams(experience_param=10.0, training_frequency=8.0, experience_scale_max=10.0)
[40]:
ps_hl = ParameterSample(pd_hl)
xs = np.random.default_rng(seed=101).gamma(1,1.9,101)
# round so that dist is 0-10
xs = [min(x, 9.9) for x in xs]
weight = 1/len(xs)
for x in xs:
ps_hl.add_variable_scenario(x, weight=weight)
ps_hl
[40]:
ParameterSample of scenarios:
- var_0
- var_1
- var_2
- var_3
- var_4
- var_5
- var_6
- var_7
- var_8
- var_9
- ... (101 total)
[41]:
ec_psf_long, hist_psf_long= prop.parameter_sample(psf_long, ps_hl, run_stochastic=True)
SCENARIOS COMPLETE: 100%|██████████| 101/101 [00:00<00:00, 158.14it/s]
[42]:
hist_psf_long.plot_line('s.experience')
[42]:
(<Figure size 300x200 with 1 Axes>,
[<Axes: title={'center': 's.experience'}, xlabel='time'>])
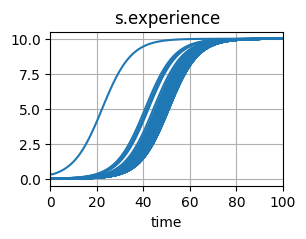
[43]:
fig, axs = hist_psf_long.plot_metric_dist([0, 40, 50, 60, 100], 's.experience', bins=20, alpha=0.5, figsize=(8,4))
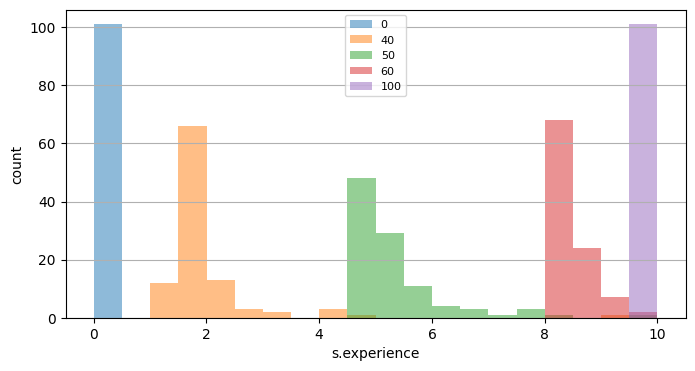
[44]:
# fig.savefig("experience_degradation.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Short-term degradation
[45]:
psf_short = PSFDegradationShort()
er, hist_short = prop.nominal(psf_short)
fig, axs = hist_short.plot_line('s.fatigue', 's.stress')
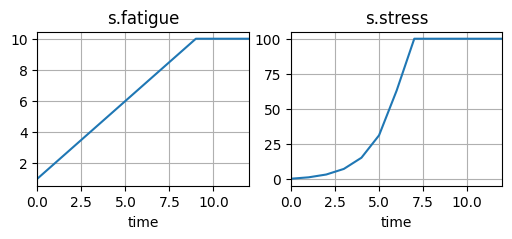
short-term degradation (over no external params)
[46]:
ps_psf_short = ParameterSample()
ps_psf_short.add_variable_replicates([], replicates=25)
ps_psf_short.scenarios()
[46]:
[ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1625865845}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep0_var_0'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 300566473}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep1_var_1'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 415938280}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep2_var_2'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 4129870959}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep3_var_3'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1207504356}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep4_var_4'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1698822850}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep5_var_5'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1804734720}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep6_var_6'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1107079035}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep7_var_7'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 2240059161}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep8_var_8'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1415794816}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep9_var_9'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 4032559193}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep10_var_10'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1622638704}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep11_var_11'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 2953570838}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep12_var_12'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 493440426}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep13_var_13'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1097593625}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep14_var_14'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 227296395}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep15_var_15'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 2133182648}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep16_var_16'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1414128234}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep17_var_17'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 2390675356}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep18_var_18'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1840706033}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep19_var_19'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 3356906507}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep20_var_20'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 3487406174}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep21_var_21'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 288020344}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep22_var_22'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 1755734049}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep23_var_23'),
ParameterScenario(sequence={}, times=(), p={}, r={'seed': 482271523}, sp={}, prob=0.04, inputparams={}, rangeid='', name='rep24_var_24')]
[47]:
ec_psf_short, hist_psf_short = prop.parameter_sample(psf_short, ps_psf_short, run_stochastic=True)
fig, axs = hist_psf_short.plot_line('s.fatigue', 's.stress', 'r.s.fatigue_param', aggregation="percentile")
SCENARIOS COMPLETE: 100%|██████████| 25/25 [00:00<00:00, 470.82it/s]
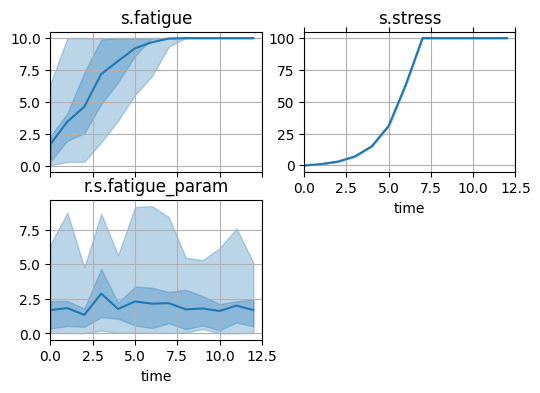
short-term degradation over long-term params
[48]:
from examples.rover.rover_degradation import PSFShortParams
pd_short_long = ParameterDomain(PSFShortParams)
pd_short_long.add_variable("experience")
ps_short_long = ParameterHistSample(hist_psf_long, 's.experience', paramdomain=pd_short_long)
ps_short_long.add_hist_groups(reps= 10, ts = [0, 40, 50, 60, 100])
# note - need to add a way to combine replicates (seeds) over replicates (input times/groups/)
[49]:
pd_short_long(10)
[49]:
PSFShortParams(experience=10.0, stress_param=0.0, fatigue_param=1.0)
[50]:
ps_short_long.scenarios()
[50]:
[ParameterScenario(sequence={}, times=(), p={'experience': 0.0147852093576427}, r={'seed': 3880807170}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_0', 't': 0}, rangeid='', name='hist_0'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.355521540650882}, r={'seed': 3880807170}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_0', 't': 40}, rangeid='', name='hist_1'),
ParameterScenario(sequence={}, times=(), p={'experience': 7.926159730949486}, r={'seed': 3880807170}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_0', 't': 50}, rangeid='', name='hist_2'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.498252713597697}, r={'seed': 3880807170}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_0', 't': 60}, rangeid='', name='hist_3'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.999122355752638}, r={'seed': 3880807170}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_0', 't': 100}, rangeid='', name='hist_4'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.003707397123126116}, r={'seed': 2555286384}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_1', 't': 0}, rangeid='', name='hist_5'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.6197044104299785}, r={'seed': 2555286384}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_1', 't': 40}, rangeid='', name='hist_6'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.890918517769909}, r={'seed': 2555286384}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_1', 't': 50}, rangeid='', name='hist_7'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.258304854924205}, r={'seed': 2555286384}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_1', 't': 60}, rangeid='', name='hist_8'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996496965739947}, r={'seed': 2555286384}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_1', 't': 100}, rangeid='', name='hist_9'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.0040328347821078905}, r={'seed': 3064130492}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_2', 't': 0}, rangeid='', name='hist_10'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.7372307652324728}, r={'seed': 3064130492}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_2', 't': 40}, rangeid='', name='hist_11'),
ParameterScenario(sequence={}, times=(), p={'experience': 5.1013174827709795}, r={'seed': 3064130492}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_2', 't': 50}, rangeid='', name='hist_12'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.376073873906922}, r={'seed': 3064130492}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_2', 't': 60}, rangeid='', name='hist_13'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996779663860996}, r={'seed': 3064130492}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_2', 't': 100}, rangeid='', name='hist_14'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.004105645810848513}, r={'seed': 1522442806}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_3', 't': 0}, rangeid='', name='hist_15'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.7630764252943243}, r={'seed': 1522442806}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_3', 't': 40}, rangeid='', name='hist_16'),
ParameterScenario(sequence={}, times=(), p={'experience': 5.1460417740129625}, r={'seed': 1522442806}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_3', 't': 50}, rangeid='', name='hist_17'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.40027584188355}, r={'seed': 1522442806}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_3', 't': 60}, rangeid='', name='hist_18'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996836779451714}, r={'seed': 1522442806}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_3', 't': 100}, rangeid='', name='hist_19'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.0037569908838189204}, r={'seed': 2192846486}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_4', 't': 0}, rangeid='', name='hist_20'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.6378293230060559}, r={'seed': 2192846486}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_4', 't': 40}, rangeid='', name='hist_21'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.924140166096661}, r={'seed': 2192846486}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_4', 't': 50}, rangeid='', name='hist_22'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.277342402550868}, r={'seed': 2192846486}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_4', 't': 60}, rangeid='', name='hist_23'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996543208332358}, r={'seed': 2192846486}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_4', 't': 100}, rangeid='', name='hist_24'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.0035576861892378416}, r={'seed': 2523984446}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_5', 't': 0}, rangeid='', name='hist_25'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.5645112885019485}, r={'seed': 2523984446}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_5', 't': 40}, rangeid='', name='hist_26'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.7879419002260555}, r={'seed': 2523984446}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_5', 't': 50}, rangeid='', name='hist_27'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.198196150411718}, r={'seed': 2523984446}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_5', 't': 60}, rangeid='', name='hist_28'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996349553773157}, r={'seed': 2523984446}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_5', 't': 100}, rangeid='', name='hist_29'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.006841983111062025}, r={'seed': 2737859614}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_6', 't': 0}, rangeid='', name='hist_30'),
ParameterScenario(sequence={}, times=(), p={'experience': 2.629719998704038}, r={'seed': 2737859614}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_6', 't': 40}, rangeid='', name='hist_31'),
ParameterScenario(sequence={}, times=(), p={'experience': 6.386297198063246}, r={'seed': 2737859614}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_6', 't': 50}, rangeid='', name='hist_32'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.974697629346908}, r={'seed': 2737859614}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_6', 't': 60}, rangeid='', name='hist_33'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.99810213629282}, r={'seed': 2737859614}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_6', 't': 100}, rangeid='', name='hist_34'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.003743727872745982}, r={'seed': 4256199784}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_7', 't': 0}, rangeid='', name='hist_35'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.632989788946346}, r={'seed': 4256199784}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_7', 't': 40}, rangeid='', name='hist_36'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.915297962215212}, r={'seed': 4256199784}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_7', 't': 50}, rangeid='', name='hist_37'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.272292001415185}, r={'seed': 4256199784}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_7', 't': 60}, rangeid='', name='hist_38'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996530961505558}, r={'seed': 4256199784}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_7', 't': 100}, rangeid='', name='hist_39'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.014556312791561945}, r={'seed': 479748714}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_8', 't': 0}, rangeid='', name='hist_40'),
ParameterScenario(sequence={}, times=(), p={'experience': 4.317146303403044}, r={'seed': 479748714}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_8', 't': 40}, rangeid='', name='hist_41'),
ParameterScenario(sequence={}, times=(), p={'experience': 7.900357779757452}, r={'seed': 479748714}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_8', 't': 50}, rangeid='', name='hist_42'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.490753491558548}, r={'seed': 479748714}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_8', 't': 60}, rangeid='', name='hist_43'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.99910853568146}, r={'seed': 479748714}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_8', 't': 100}, rangeid='', name='hist_44'),
ParameterScenario(sequence={}, times=(), p={'experience': 0.003917700316414757}, r={'seed': 705439826}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_9', 't': 0}, rangeid='', name='hist_45'),
ParameterScenario(sequence={}, times=(), p={'experience': 1.6960297046671722}, r={'seed': 705439826}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_9', 't': 40}, rangeid='', name='hist_46'),
ParameterScenario(sequence={}, times=(), p={'experience': 5.028890434716651}, r={'seed': 705439826}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_9', 't': 50}, rangeid='', name='hist_47'),
ParameterScenario(sequence={}, times=(), p={'experience': 8.336273464537053}, r={'seed': 705439826}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_9', 't': 60}, rangeid='', name='hist_48'),
ParameterScenario(sequence={}, times=(), p={'experience': 9.996685016931059}, r={'seed': 705439826}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'var_9', 't': 100}, rangeid='', name='hist_49')]
[51]:
ec, hist_short_long = prop.parameter_sample(psf_short, ps_short_long, run_stochastic=True)
SCENARIOS COMPLETE: 100%|██████████| 50/50 [00:00<00:00, 451.94it/s]
[52]:
fig, axs = hist_short_long.plot_line('s.fatigue', 's.stress', 'r.s.fatigue_param', aggregation="percentile")
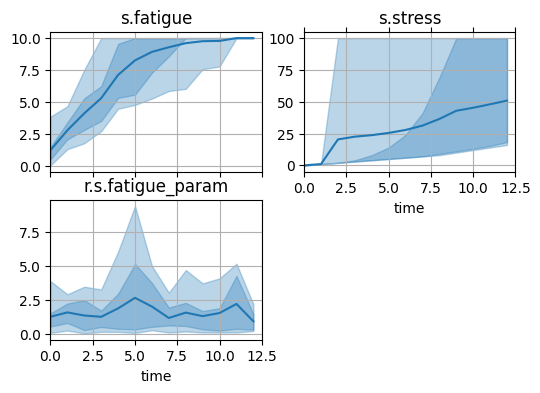
[53]:
# fig.savefig("stress_degradation.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Now sample in the model:
[54]:
from examples.rover.rover_model_human import RoverHuman, RoverHumanParam
pd_comb_mdl = ParameterDomain(RoverHumanParam)
# pd_comb_mdl.add_constant('drive_modes', {"mode_args": "degradation"})
pd_comb_mdl.add_variable("psfs.fatigue")
pd_comb_mdl.add_variables("psfs.stress")
rpd.add_constant('drive_modes', {"mode_args": "degradation"})
pd_comb_mdl(1,1)
[54]:
RoverHumanParam(ground=GroundParam(linetype='sine', amp=1.0, period=6.283185307179586, radius=20.0, x_start=10.0, y_end=10.0, x_min=0.0, x_max=30.0, x_res=0.1, path_buffer_on=0.2, path_buffer_poor=0.3, path_buffer_near=0.4, dest_buffer_on=1.0, dest_buffer_near=2.0), correction=ResCorrection(ub_f=10.0, lb_f=-1.0, ub_t=10.0, lb_t=0.0, ub_d=2.0, lb_d=-2.0, cor_d=0.0, cor_t=0.0, cor_f=0.0), degradation=DegParam(friction=0.0, drift=0.0), drive_modes={'mode_args': 'set'}, psfs=PSFParam(fatigue=1.0, stress=1.0))
[55]:
ps_comb_mdl = ParameterHistSample(hist_short_long, "s.fatigue", "s.stress", paramdomain=pd_comb_mdl)
ps_comb_mdl.add_hist_groups(reps= 10, ts = [0, 1, 3, 5, 6, 8])
ps_comb_mdl.scenarios()
[55]:
[ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1.0927235728185847, 'stress': 0.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 0}, rangeid='', name='hist_0'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.271649806862883, 'stress': 1.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 1}, rangeid='', name='hist_1'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4.329808380933794, 'stress': 100.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 3}, rangeid='', name='hist_2'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5.572591443505191, 'stress': 100.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 5}, rangeid='', name='hist_3'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 100.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 6}, rangeid='', name='hist_4'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 100.0}}, r={'seed': 3895460050}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_0', 't': 8}, rangeid='', name='hist_5'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1.0927235728185847, 'stress': 0.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 0}, rangeid='', name='hist_6'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.271649806862883, 'stress': 1.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 1}, rangeid='', name='hist_7'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4.329808380933794, 'stress': 3.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 3}, rangeid='', name='hist_8'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5.572591443505191, 'stress': 6.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 5}, rangeid='', name='hist_9'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 8.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 6}, rangeid='', name='hist_10'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 15.0}}, r={'seed': 2394736157}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_1', 't': 8}, rangeid='', name='hist_11'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1.0927235728185847, 'stress': 0.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 0}, rangeid='', name='hist_12'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.271649806862883, 'stress': 1.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 1}, rangeid='', name='hist_13'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4.329808380933794, 'stress': 3.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 3}, rangeid='', name='hist_14'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5.572591443505191, 'stress': 5.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 5}, rangeid='', name='hist_15'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 6}, rangeid='', name='hist_16'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 10.0}}, r={'seed': 239760074}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_2', 't': 8}, rangeid='', name='hist_17'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1.0927235728185847, 'stress': 0.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 0}, rangeid='', name='hist_18'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.271649806862883, 'stress': 1.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 1}, rangeid='', name='hist_19'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4.329808380933794, 'stress': 3.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 3}, rangeid='', name='hist_20'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5.572591443505191, 'stress': 5.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 5}, rangeid='', name='hist_21'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 6}, rangeid='', name='hist_22'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 9.0}}, r={'seed': 773722268}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_3', 't': 8}, rangeid='', name='hist_23'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1.0927235728185847, 'stress': 0.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 0}, rangeid='', name='hist_24'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.271649806862883, 'stress': 1.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 1}, rangeid='', name='hist_25'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4.329808380933794, 'stress': 3.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 3}, rangeid='', name='hist_26'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5.572591443505191, 'stress': 5.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 5}, rangeid='', name='hist_27'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 6}, rangeid='', name='hist_28'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 8.0}}, r={'seed': 1527804290}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_4', 't': 8}, rangeid='', name='hist_29'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0.5187639363463094, 'stress': 0.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 0}, rangeid='', name='hist_30'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.4127657765544988, 'stress': 1.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 1}, rangeid='', name='hist_31'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6.2414738429941785, 'stress': 100.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 3}, rangeid='', name='hist_32'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 100.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 5}, rangeid='', name='hist_33'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 100.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 6}, rangeid='', name='hist_34'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 100.0}}, r={'seed': 320346681}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_5', 't': 8}, rangeid='', name='hist_35'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0.5187639363463094, 'stress': 0.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 0}, rangeid='', name='hist_36'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.4127657765544988, 'stress': 1.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 1}, rangeid='', name='hist_37'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6.2414738429941785, 'stress': 4.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 3}, rangeid='', name='hist_38'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 14.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 5}, rangeid='', name='hist_39'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 25.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 6}, rangeid='', name='hist_40'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 70.0}}, r={'seed': 2849079553}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_6', 't': 8}, rangeid='', name='hist_41'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0.5187639363463094, 'stress': 0.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 0}, rangeid='', name='hist_42'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.4127657765544988, 'stress': 1.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 1}, rangeid='', name='hist_43'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6.2414738429941785, 'stress': 3.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 3}, rangeid='', name='hist_44'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 5}, rangeid='', name='hist_45'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 8.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 6}, rangeid='', name='hist_46'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 14.0}}, r={'seed': 3255028081}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_7', 't': 8}, rangeid='', name='hist_47'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0.5187639363463094, 'stress': 0.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 0}, rangeid='', name='hist_48'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.4127657765544988, 'stress': 1.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 1}, rangeid='', name='hist_49'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6.2414738429941785, 'stress': 3.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 3}, rangeid='', name='hist_50'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 5.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 5}, rangeid='', name='hist_51'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 6}, rangeid='', name='hist_52'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 9.0}}, r={'seed': 1739455535}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_8', 't': 8}, rangeid='', name='hist_53'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0.5187639363463094, 'stress': 0.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 0}, rangeid='', name='hist_54'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3.4127657765544988, 'stress': 1.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 1}, rangeid='', name='hist_55'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6.2414738429941785, 'stress': 3.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 3}, rangeid='', name='hist_56'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 5.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 5}, rangeid='', name='hist_57'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 6.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 6}, rangeid='', name='hist_58'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10.0, 'stress': 8.0}}, r={'seed': 3296049684}, sp={}, prob=1.0, inputparams={'comp_group': 'default', 'rep': 'hist_9', 't': 8}, rangeid='', name='hist_59')]
[56]:
mdl_hum = RoverHuman()
[57]:
ec, hist = prop.nominal(mdl_hum)
plot_map(mdl_hum, hist)
ec
[57]:
endclass:
--rate: 1.0
--cost: 0
--prob: 1.0
--expected_cost: 0
--in_bound: True
--at_finish: True
--line_dist: 1
--num_modes: 0
--end_dist: 0.0
--tot_deviation: 0.11859644458085598
--faults: {}
--classification: nominal mission
--end_x: 29.254775331608617
--end_y: -0.7827640334587979
--endpt: array(2)
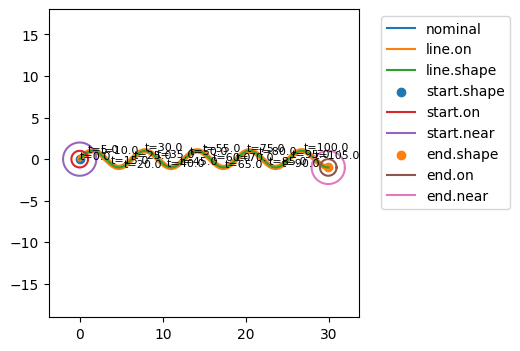
[58]:
ec_comb, hist_comb = prop.parameter_sample(mdl_hum, ps_comb_mdl)
SCENARIOS COMPLETE: 100%|██████████| 60/60 [00:23<00:00, 2.58it/s]
[59]:
fig, ax = plot_map(mdl_hum, hist_comb)
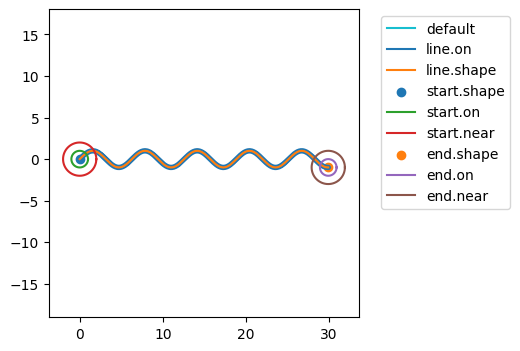
[60]:
hist_comb.plot_line('flows.psfs.s.attention', 'flows.motor_control.s.rpower', 'flows.motor_control.s.lpower')
[60]:
(<Figure size 600x400 with 4 Axes>,
array([<Axes: title={'center': 'flows.psfs.s.attention'}, xlabel=' '>,
<Axes: title={'center': 'flows.motor_control.s.rpower'}, xlabel='time'>,
<Axes: title={'center': 'flows.motor_control.s.lpower'}, xlabel='time'>,
<Axes: >], dtype=object))
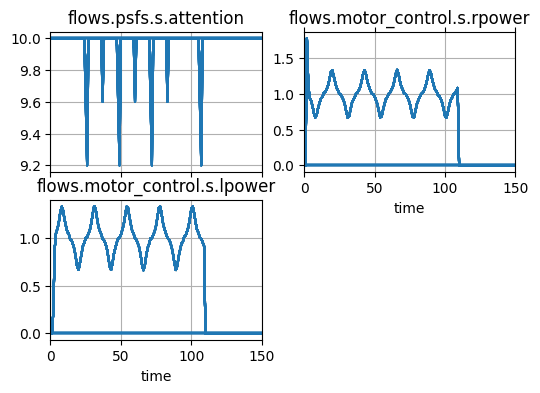
[61]:
ne = NominalEnvelope(ps_comb_mdl, ec_comb, 'at_finish',
'p.psfs.fatigue', 'p.psfs.stress',
func=lambda x: x == True)
ne.plot_scatter()
[61]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='p.psfs.fatigue', ylabel='p.psfs.stress'>)
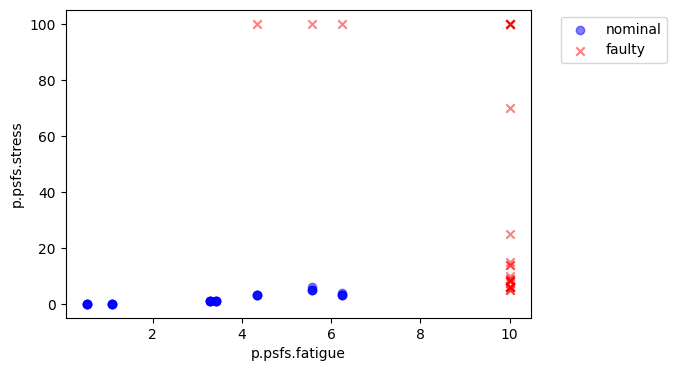
(note, this is different in the turn case because of the long straightaway)
[62]:
mdl_hum = RoverHuman(p={'ground': {'linetype': 'turn'}})
ec, hist = prop.nominal(mdl_hum)
plot_map(mdl_hum, hist)
ec
[62]:
endclass:
--rate: 1.0
--cost: 0
--prob: 1.0
--expected_cost: 0
--in_bound: True
--at_finish: True
--line_dist: 1
--num_modes: 0
--end_dist: 0.0
--tot_deviation: 0.005246344989065292
--faults: {}
--classification: nominal mission
--end_x: 29.813614084369863
--end_y: 17.26588133276667
--endpt: array(2)
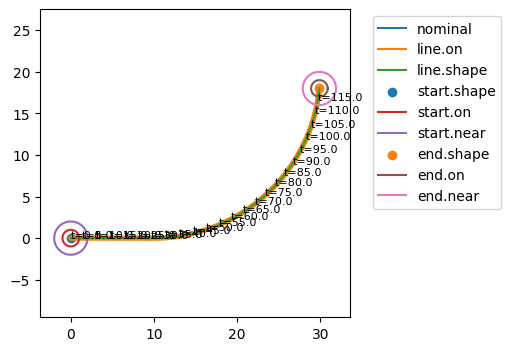
[63]:
ec_comb, hist_comb = prop.parameter_sample(mdl_hum, ps_comb_mdl)
SCENARIOS COMPLETE: 100%|██████████| 60/60 [00:23<00:00, 2.59it/s]
[64]:
fig, ax = plot_map(mdl_hum, hist_comb)
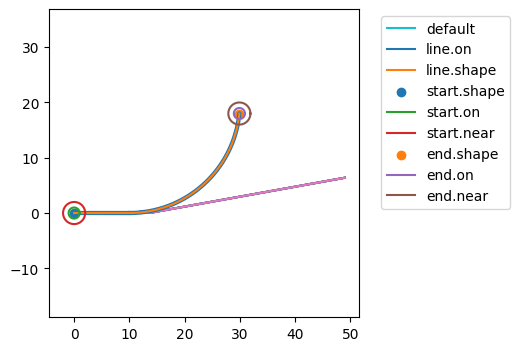
[65]:
hist_comb.plot_line('flows.psfs.s.attention', 'flows.motor_control.s.rpower', 'flows.motor_control.s.lpower')
[65]:
(<Figure size 600x400 with 4 Axes>,
array([<Axes: title={'center': 'flows.psfs.s.attention'}, xlabel=' '>,
<Axes: title={'center': 'flows.motor_control.s.rpower'}, xlabel='time'>,
<Axes: title={'center': 'flows.motor_control.s.lpower'}, xlabel='time'>,
<Axes: >], dtype=object))
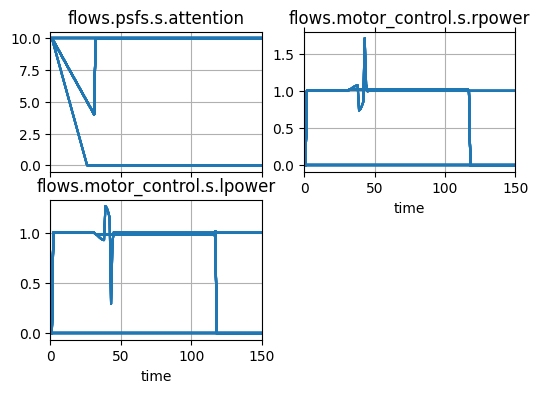
[66]:
ne = NominalEnvelope(ps_comb_mdl, ec_comb, 'at_finish',
'p.psfs.fatigue', 'p.psfs.stress',
func=lambda x: x == True)
ne.plot_scatter()
[66]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='p.psfs.fatigue', ylabel='p.psfs.stress'>)
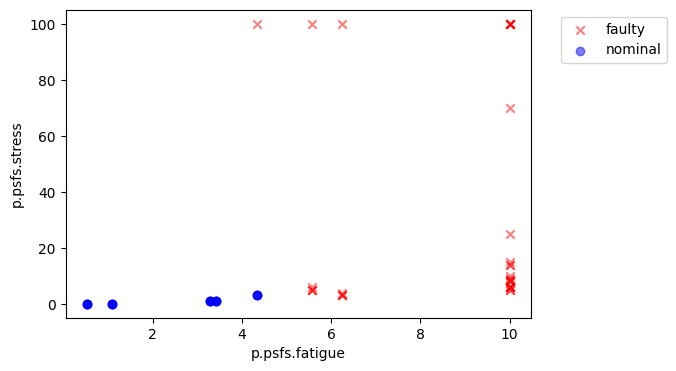
We can thus see how degradation time effects this:
[67]:
ne = NominalEnvelope(ps_comb_mdl, ec_comb, 'at_finish',
'inputparams.t', 'inputparams.rep',
func=lambda x: x == True)
ne.plot_scatter()
[67]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='inputparams.t', ylabel='inputparams.rep'>)
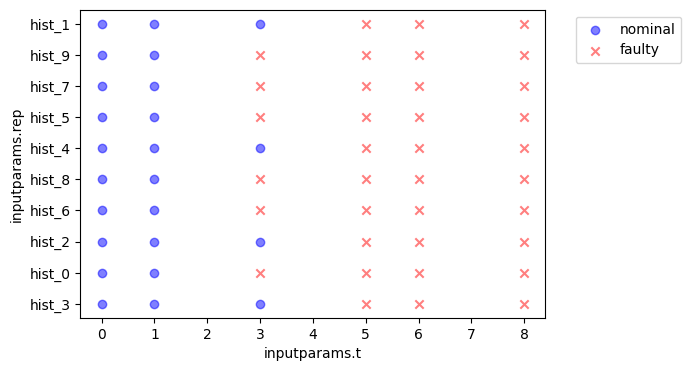
To re-implement: - Need to be able to jointly plot/tabulate by single-day time + multi-day time - Combining approaches?
As for the human resilience:
[68]:
ec_nest, hist_nest, app_nest = prop.nested_sample(mdl, phs, faultdomains={'drive_faults': (('all_fxnclass_modes', 'Drive'), {})},
faultsamples={'drive_faults': (('fault_phases', 'drive_faults', "start"), {})},
pool=mp.Pool(5))
NESTED SCENARIOS COMPLETE: 100%|██████████| 40/40 [00:17<00:00, 2.32it/s]
[69]:
nc = NestedComparison(ec_nest, phs, ['inputparams.t'], app_nest, ['fault'], metrics=['tot_deviation', 'end_dist'], default_stat=np.mean, ci_metrics=['end_dist', 'tot_deviation'])
[70]:
nc.sort_by_factor("fault")
nc.sort_by_factor("inputparams.t")
fig, ax = nc.as_plots('end_dist', 'tot_deviation', figsize=(8,4))
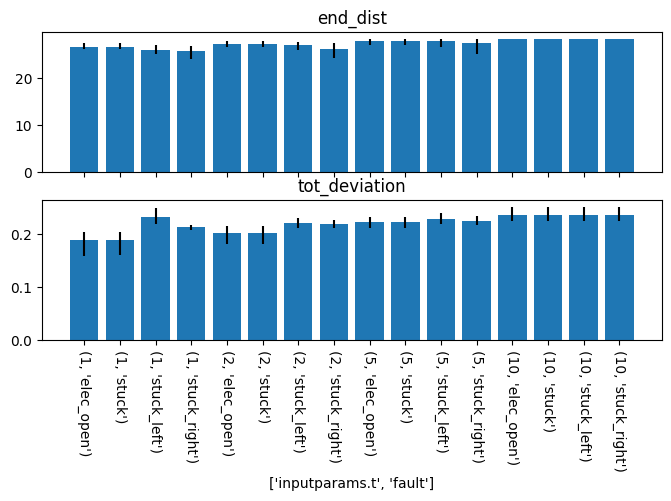
[71]:
# fig.savefig("human_resilience_degradation.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Combined Degradation
Idea: make same resilience plots as before but with degradation of resilience to drive faults at 0, 4, and 8 hours of fatigue.
Need method of doing this: - take params for one and replace with the other (kind of hacky and requires independence) - create composite of both? (how do you determine the merge between output params?) - use a combined method with both histories as input and sample over t_life and t_day (use this one)
[ ]:
Resilience assessment
[ ]: