Human Failure Representation
This paper covers the exploration and analysis of error producing conditions by a human operator in the rover model.
[1]:
from examples.rover.rover_model_human import RoverHuman
from examples.rover.rover_model import plot_map
import fmdtools.sim.propagate as prop
import fmdtools.analyze as an
from fmdtools.sim.sample import ParameterSample, ParameterDomain, FaultSample, FaultDomain
import numpy as np
import matplotlib.pyplot as plt
Visualizing Rover Structure
[2]:
mdl = RoverHuman()
mdl.fxns
[2]:
{'power': power Power
- PowerState(charge=100.0, power=0.0)
- PowerMode(mode=off, faults=set()),
'perception': perception Perception
- PerceptionMode(mode=off, faults=set()),
'communications': communications Communications,
'operator': operator Operator
- Mode(mode=nominal, faults=set()),
'plan_path': plan_path PlanPath
- PlanPathState(u_self=array([0., 0.]), u_lin=array([0., 0.]), u_lin_dev=array([0., 0.]), rdiff=0.0, vel_adj=1.0)
- PlanPathMode(mode=standby, faults=set()),
'override': override Override
- OverrideMode(mode=off, faults=set()),
'drive': drive Drive
- FaultStates(transfer=1.0, friction=0.0, drift=0.0)
- DriveMode(mode=nominal, faults=set())}
[3]:
#%matplotlib qt
#an.graph.set_pos(mdl, gtype='fxnflowgraph')
[4]:
#%matplotlib inline
[5]:
g = mdl.as_modelgraph()
fig, ax = g.draw()
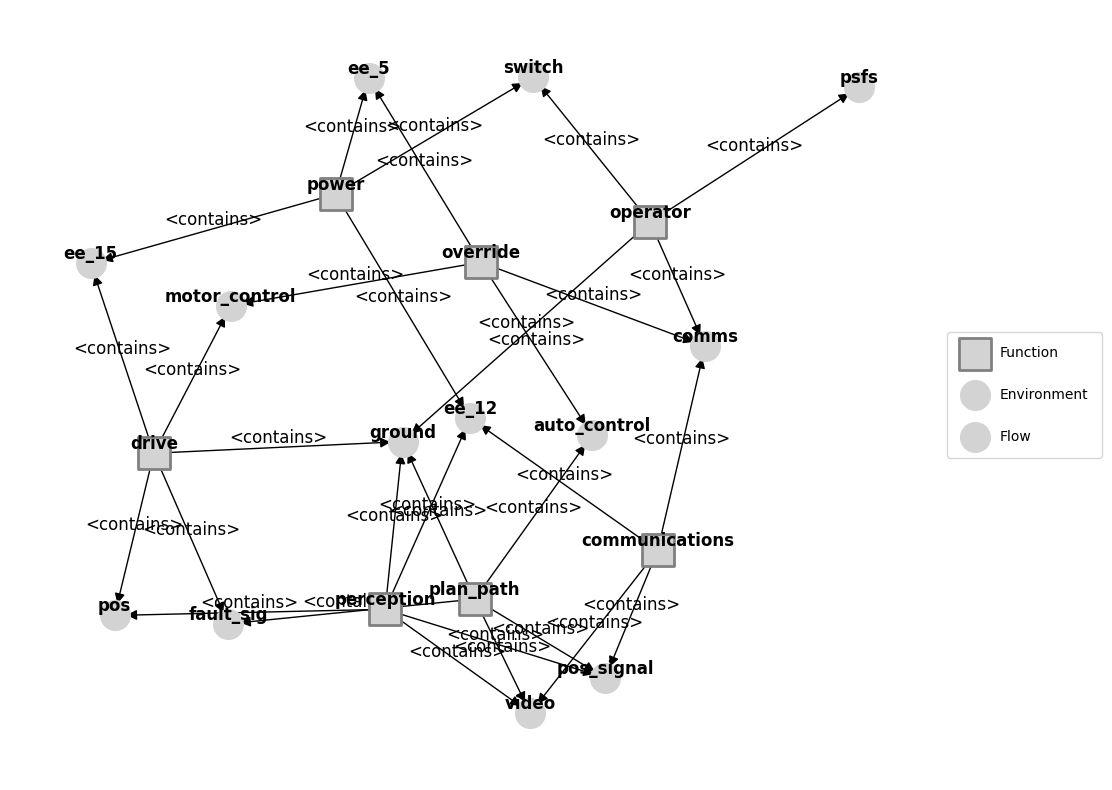
[6]:
fig.savefig("rover_structure.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Visualizing Action Sequence Graph for the Controller
[7]:
from examples.rover.rover_model_human import asg_pos
ag = mdl.fxns['operator'].aa.as_modelgraph()
ag.set_pos(**asg_pos)
fig, ax = ag.draw()
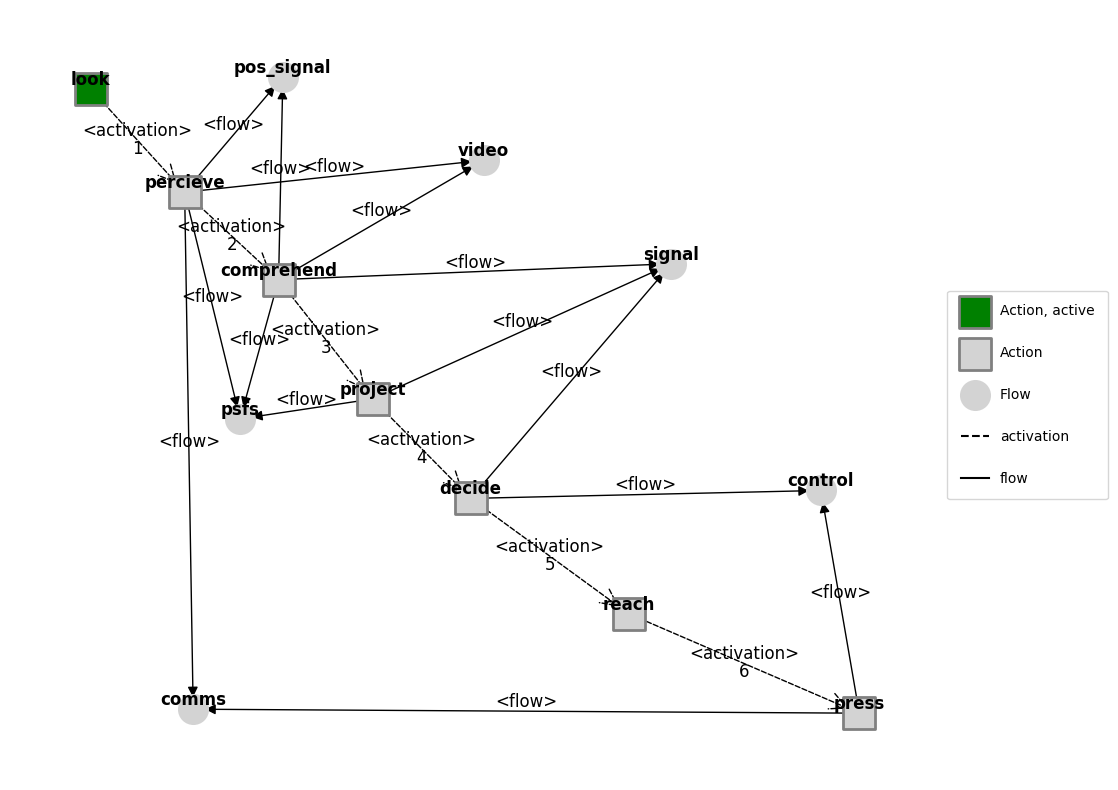
[8]:
fig.savefig("action_graph.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Testing behavioral simulation
Execution order
[9]:
g.set_exec_order(mdl)
fig, ax = g.draw()
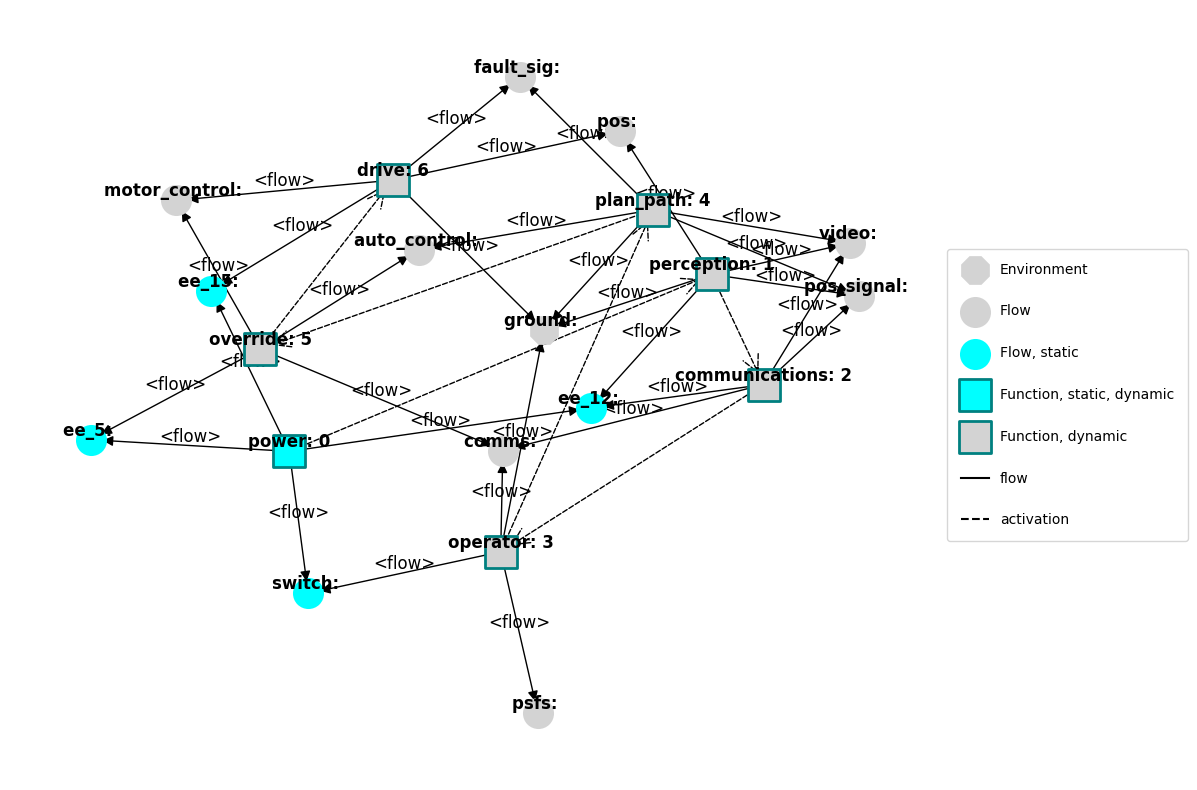
Performance on turn
[10]:
mdl = RoverHuman(p={'ground': 'turn'})
endresults, mdlhist = prop.nominal(mdl)
plot_map(mdl, mdlhist)
[10]:
(<Figure size 400x400 with 1 Axes>, <Axes: xlabel='x', ylabel='y'>)
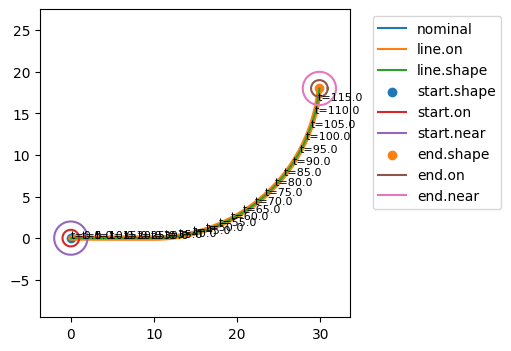
Performance on sine map
[11]:
mdl = RoverHuman()
endresults, mdlhist = prop.nominal(mdl)
plot_map(mdl, mdlhist)
[11]:
(<Figure size 400x400 with 1 Axes>, <Axes: xlabel='x', ylabel='y'>)
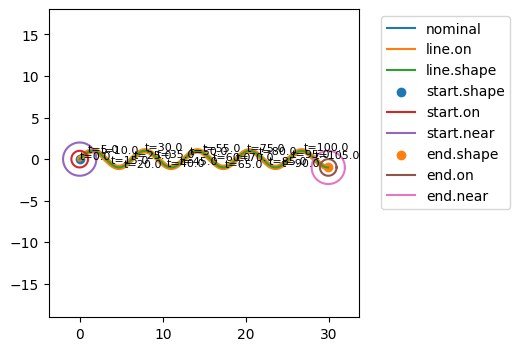
[12]:
from fmdtools.analyze.phases import from_hist
pm = from_hist(mdlhist)
[13]:
pm
[13]:
{'power': PhaseMap({'off': [0.0, 0.0], 'supply': [1.0, 109.0]}, {'off': {'off'}, 'supply': {'supply'}}),
'perception': PhaseMap({'off': [0.0, 1.0], 'feed': [2.0, 109.0]}, {'off': {'off'}, 'feed': {'feed'}}),
'look': PhaseMap({'nominal': [0.0, 109.0]}, {'nominal': {'nominal'}}),
'percieve': PhaseMap({'no_action': [0.0, 1.0], 'nominal': [2.0, 109.0]}, {'no_action': {'no_action'}, 'nominal': {'nominal'}}),
'comprehend': PhaseMap({'nominal': [0.0, 109.0]}, {'nominal': {'nominal'}}),
'project': PhaseMap({'nominal': [0.0, 109.0]}, {'nominal': {'nominal'}}),
'decide': PhaseMap({'nominal': [0.0, 109.0]}, {'nominal': {'nominal'}}),
'reach': PhaseMap({'nominal': [0.0, 109.0]}, {'nominal': {'nominal'}}),
'plan_path': PhaseMap({'standby': [0.0, 4.0], 'drive': [5.0, 109.0]}, {'standby': {'standby'}, 'drive': {'drive'}}),
'override': PhaseMap({'off': [0.0, 1.0], 'override': [2.0, 109.0]}, {'off': {'off'}, 'override': {'override'}})}
[14]:
phase_fig = pm['override'].plot()
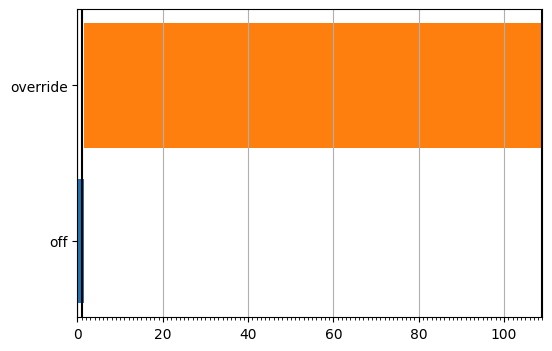
Testing Faults
[15]:
#app=SampleApproach(mdl, faults='Controller', phases={'drive':phases['Operations']['drive']})
fd = FaultDomain(mdl)
fd.add_all_fxn_modes('operator')
fd
[15]:
FaultDomain with faults:
-('roverhuman.fxns.operator.aa.acts.look', 'failed_no_action')
-('roverhuman.fxns.operator.aa.acts.percieve', 'failed_no_action')
-('roverhuman.fxns.operator.aa.acts.percieve', 'not_visible')
-('roverhuman.fxns.operator.aa.acts.percieve', 'wrong_position')
-('roverhuman.fxns.operator.aa.acts.comprehend', 'failed_no_action')
-('roverhuman.fxns.operator.aa.acts.project', 'failed_fast')
-('roverhuman.fxns.operator.aa.acts.project', 'failed_no_action')
-('roverhuman.fxns.operator.aa.acts.project', 'failed_slow')
-('roverhuman.fxns.operator.aa.acts.project', 'failed_turn_left')
-('roverhuman.fxns.operator.aa.acts.project', 'failed_turn_right')
-...more
[16]:
fs = FaultSample(fd, phasemap = pm['override'])
fs.add_fault_phases('override')
fs
[16]:
FaultSample of scenarios:
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t56p0
- roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t56p0
- roverhuman_fxns_operator_aa_acts_percieve_not_visible_t56p0
- roverhuman_fxns_operator_aa_acts_percieve_wrong_position_t56p0
- roverhuman_fxns_operator_aa_acts_comprehend_failed_no_action_t56p0
- roverhuman_fxns_operator_aa_acts_project_failed_fast_t56p0
- roverhuman_fxns_operator_aa_acts_project_failed_no_action_t56p0
- roverhuman_fxns_operator_aa_acts_project_failed_slow_t56p0
- roverhuman_fxns_operator_aa_acts_project_failed_turn_left_t56p0
- roverhuman_fxns_operator_aa_acts_project_failed_turn_right_t56p0
- ... (19 total)
[17]:
fs.scenarios()
[17]:
[SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.look': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.look', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_look_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.percieve': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.percieve', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.percieve': ['not_visible']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.percieve', fault='not_visible', rate=1.0, name='roverhuman_fxns_operator_aa_acts_percieve_not_visible_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.percieve': ['wrong_position']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.percieve', fault='wrong_position', rate=1.0, name='roverhuman_fxns_operator_aa_acts_percieve_wrong_position_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.comprehend': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.comprehend', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_comprehend_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.project': ['failed_fast']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.project', fault='failed_fast', rate=1.0, name='roverhuman_fxns_operator_aa_acts_project_failed_fast_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.project': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.project', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_project_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.project': ['failed_slow']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.project', fault='failed_slow', rate=1.0, name='roverhuman_fxns_operator_aa_acts_project_failed_slow_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.project': ['failed_turn_left']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.project', fault='failed_turn_left', rate=1.0, name='roverhuman_fxns_operator_aa_acts_project_failed_turn_left_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.project': ['failed_turn_right']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.project', fault='failed_turn_right', rate=1.0, name='roverhuman_fxns_operator_aa_acts_project_failed_turn_right_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.decide': ['failed_fast']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.decide', fault='failed_fast', rate=1.0, name='roverhuman_fxns_operator_aa_acts_decide_failed_fast_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.decide': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.decide', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_decide_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.decide': ['failed_slow']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.decide', fault='failed_slow', rate=1.0, name='roverhuman_fxns_operator_aa_acts_decide_failed_slow_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.decide': ['failed_turn_left']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.decide', fault='failed_turn_left', rate=1.0, name='roverhuman_fxns_operator_aa_acts_decide_failed_turn_left_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.decide': ['failed_turn_right']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.decide', fault='failed_turn_right', rate=1.0, name='roverhuman_fxns_operator_aa_acts_decide_failed_turn_right_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.reach': ['failed_no_action']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.reach', fault='failed_no_action', rate=1.0, name='roverhuman_fxns_operator_aa_acts_reach_failed_no_action_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.press': ['failed_left']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.press', fault='failed_left', rate=1.0, name='roverhuman_fxns_operator_aa_acts_press_failed_left_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.press': ['failed_right']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.press', fault='failed_right', rate=1.0, name='roverhuman_fxns_operator_aa_acts_press_failed_right_t56p0', time=56.0, phase='override'),
SingleFaultScenario(sequence={56.0: Injection(faults={'roverhuman.fxns.operator.aa.acts.press': ['no_press']}, disturbances={})}, times=(56.0,), function='roverhuman.fxns.operator.aa.acts.press', fault='no_press', rate=1.0, name='roverhuman_fxns_operator_aa_acts_press_no_press_t56p0', time=56.0, phase='override')]
[18]:
ecs, hists = prop.fault_sample(mdl, fs)
SCENARIOS COMPLETE: 100%|██████████| 19/19 [00:05<00:00, 3.68it/s]
[19]:
fig, ax = plot_map(mdl, hists)
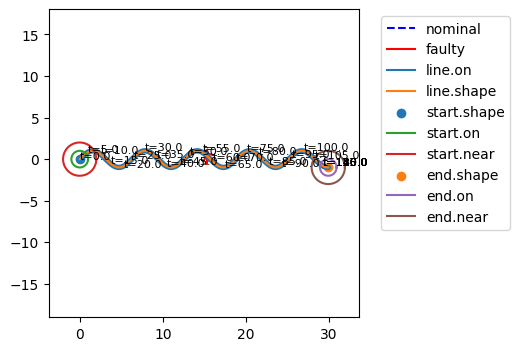
[20]:
fig, ax = plot_map(mdl, hists)
ax.set_xlim(10, 20)
ax.set_ylim(-5, 5)
[20]:
(-5.0, 5.0)
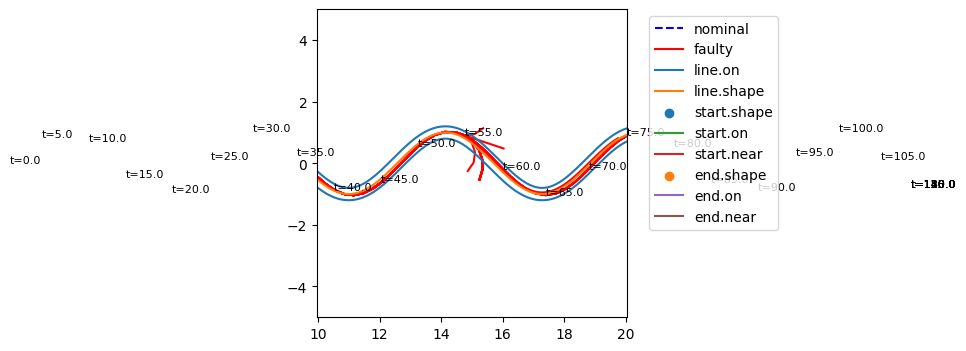
[21]:
fig.savefig("rover_map.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
Below shows the impact of each fault:
[22]:
tab = an.tabulate.result_summary_fmea(ecs, hists,
*mdl.flows, *mdl.fxns,
metrics = ["in_bound", "at_finish", "end_dist", "faults", "classification", "end_x", "end_y"])
tab
[22]:
degraded | faulty | in_bound | at_finish | end_dist | faults | classification | end_x | end_y | |
---|---|---|---|---|---|---|---|---|---|
roverhuman_fxns_operator_aa_acts_look_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [look.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_percieve_not_visible_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_percieve_wrong_position_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_comprehend_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, comprehend.failed_no_ac... | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_project_failed_fast_t56p0 | ['ground', 'pos_signal', 'pos', 'ee_15', 'vide... | ['operator'] | False | False | 12.955107 | [percieve.not_visible, project.failed_fast] | incomplete mission faulty | 16.023518 | 0.480458 |
roverhuman_fxns_operator_aa_acts_project_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, project.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_project_failed_slow_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'ee_12... | ['operator'] | True | False | 9.31949 | [project.failed_slow] | incomplete mission faulty | 19.740872 | 0.81243 |
roverhuman_fxns_operator_aa_acts_project_failed_turn_left_t56p0 | ['ground', 'pos_signal', 'pos', 'video', 'auto... | ['operator'] | False | False | 14.057688 | [percieve.not_visible, project.failed_turn_left] | incomplete mission faulty | 14.862043 | -0.251773 |
roverhuman_fxns_operator_aa_acts_project_failed_turn_right_t56p0 | ['ground', 'pos_signal', 'pos', 'video', 'auto... | ['operator'] | False | False | 13.693675 | [percieve.not_visible, project.failed_turn_right] | incomplete mission faulty | 15.363988 | 1.140047 |
roverhuman_fxns_operator_aa_acts_decide_failed_fast_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_fast] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_slow_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_slow] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_turn_left_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_turn_left] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_turn_right_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_turn_right] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_reach_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, reach.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_press_failed_left_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.failed_left] | faulty | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_press_failed_right_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.failed_right] | faulty | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_press_no_press_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.no_press] | faulty | 29.254775 | -0.782764 |
nominal | [] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
[23]:
tab.sort_values('end_dist', ascending=False)
[23]:
degraded | faulty | in_bound | at_finish | end_dist | faults | classification | end_x | end_y | |
---|---|---|---|---|---|---|---|---|---|
roverhuman_fxns_operator_aa_acts_project_failed_turn_left_t56p0 | ['ground', 'pos_signal', 'pos', 'video', 'auto... | ['operator'] | False | False | 14.057688 | [percieve.not_visible, project.failed_turn_left] | incomplete mission faulty | 14.862043 | -0.251773 |
roverhuman_fxns_operator_aa_acts_project_failed_turn_right_t56p0 | ['ground', 'pos_signal', 'pos', 'video', 'auto... | ['operator'] | False | False | 13.693675 | [percieve.not_visible, project.failed_turn_right] | incomplete mission faulty | 15.363988 | 1.140047 |
roverhuman_fxns_operator_aa_acts_look_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [look.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_reach_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, reach.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_turn_right_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_turn_right] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_turn_left_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_turn_left] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_slow_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_slow] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_decide_failed_fast_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, decide.failed_fast] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_project_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, project.failed_no_action] | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_comprehend_failed_no_action_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'video... | ['operator'] | False | False | 13.663813 | [percieve.not_visible, comprehend.failed_no_ac... | incomplete mission faulty | 15.24424 | -0.529458 |
roverhuman_fxns_operator_aa_acts_project_failed_fast_t56p0 | ['ground', 'pos_signal', 'pos', 'ee_15', 'vide... | ['operator'] | False | False | 12.955107 | [percieve.not_visible, project.failed_fast] | incomplete mission faulty | 16.023518 | 0.480458 |
roverhuman_fxns_operator_aa_acts_project_failed_slow_t56p0 | ['ground', 'psfs', 'pos_signal', 'pos', 'ee_12... | ['operator'] | True | False | 9.31949 | [project.failed_slow] | incomplete mission faulty | 19.740872 | 0.81243 |
roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_percieve_wrong_position_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_percieve_not_visible_t56p0 | ['ground'] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_press_failed_left_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.failed_left] | faulty | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_press_failed_right_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.failed_right] | faulty | 29.254775 | -0.782764 |
roverhuman_fxns_operator_aa_acts_press_no_press_t56p0 | ['ground', 'operator'] | ['operator'] | True | True | 0.0 | [press.no_press] | faulty | 29.254775 | -0.782764 |
nominal | [] | [] | True | True | 0.0 | [] | nominal mission | 29.254775 | -0.782764 |
[24]:
print(tab.to_latex())
\begin{tabular}{llllllllll}
\toprule
& degraded & faulty & in_bound & at_finish & end_dist & faults & classification & end_x & end_y \\
\midrule
roverhuman_fxns_operator_aa_acts_look_failed_no_action_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['look.failed_no_action'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t56p0 & ['ground'] & [] & True & True & 0.000000 & [] & nominal mission & 29.254775 & -0.782764 \\
roverhuman_fxns_operator_aa_acts_percieve_not_visible_t56p0 & ['ground'] & [] & True & True & 0.000000 & [] & nominal mission & 29.254775 & -0.782764 \\
roverhuman_fxns_operator_aa_acts_percieve_wrong_position_t56p0 & ['ground'] & [] & True & True & 0.000000 & [] & nominal mission & 29.254775 & -0.782764 \\
roverhuman_fxns_operator_aa_acts_comprehend_failed_no_action_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'comprehend.failed_no_action'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_project_failed_fast_t56p0 & ['ground', 'pos_signal', 'pos', 'ee_15', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 12.955107 & ['percieve.not_visible', 'project.failed_fast'] & incomplete mission faulty & 16.023518 & 0.480458 \\
roverhuman_fxns_operator_aa_acts_project_failed_no_action_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'project.failed_no_action'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_project_failed_slow_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'ee_12', 'ee_5', 'ee_15', 'video', 'auto_control', 'motor_control', 'switch', 'comms', 'power', 'perception', 'operator', 'plan_path', 'override'] & ['operator'] & True & False & 9.319490 & ['project.failed_slow'] & incomplete mission faulty & 19.740872 & 0.812430 \\
roverhuman_fxns_operator_aa_acts_project_failed_turn_left_t56p0 & ['ground', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 14.057688 & ['percieve.not_visible', 'project.failed_turn_left'] & incomplete mission faulty & 14.862043 & -0.251773 \\
roverhuman_fxns_operator_aa_acts_project_failed_turn_right_t56p0 & ['ground', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.693675 & ['percieve.not_visible', 'project.failed_turn_right'] & incomplete mission faulty & 15.363988 & 1.140047 \\
roverhuman_fxns_operator_aa_acts_decide_failed_fast_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'decide.failed_fast'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_decide_failed_no_action_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'decide.failed_no_action'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_decide_failed_slow_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'decide.failed_slow'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_decide_failed_turn_left_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'decide.failed_turn_left'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_decide_failed_turn_right_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'decide.failed_turn_right'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_reach_failed_no_action_t56p0 & ['ground', 'psfs', 'pos_signal', 'pos', 'video', 'auto_control', 'motor_control', 'comms', 'power', 'operator', 'plan_path'] & ['operator'] & False & False & 13.663813 & ['percieve.not_visible', 'reach.failed_no_action'] & incomplete mission faulty & 15.244240 & -0.529458 \\
roverhuman_fxns_operator_aa_acts_press_failed_left_t56p0 & ['ground', 'operator'] & ['operator'] & True & True & 0.000000 & ['press.failed_left'] & faulty & 29.254775 & -0.782764 \\
roverhuman_fxns_operator_aa_acts_press_failed_right_t56p0 & ['ground', 'operator'] & ['operator'] & True & True & 0.000000 & ['press.failed_right'] & faulty & 29.254775 & -0.782764 \\
roverhuman_fxns_operator_aa_acts_press_no_press_t56p0 & ['ground', 'operator'] & ['operator'] & True & True & 0.000000 & ['press.no_press'] & faulty & 29.254775 & -0.782764 \\
nominal & [] & [] & True & True & 0.000000 & [] & nominal mission & 29.254775 & -0.782764 \\
\bottomrule
\end{tabular}
As shown, most modes now have an effect.
Examining Performance Shaping Factors
[25]:
from examples.rover.rover_model_human import RoverHumanParam
pd = ParameterDomain(RoverHumanParam)
#pd.add_constant('ground.linetype', 'sine')
#pd.add_constant('ground.amp', 4)
pd.add_variable('psfs.fatigue', var_lim=(0, 11))
pd.add_variable('psfs.attention', var_lim=(0, 11))
pd
[25]:
ParameterDomain with:
- variables: {'psfs.fatigue': (0, 11), 'psfs.attention': (0, 11)}
- constants: {}
- parameter_initializer: RoverHumanParam
[26]:
ps = ParameterSample(pd)
ps.add_variable_ranges(comb_kwargs={'resolution': 1})
ps
[26]:
ParameterSample of scenarios:
- rep0_range_0
- rep0_range_1
- rep0_range_2
- rep0_range_3
- rep0_range_4
- rep0_range_5
- rep0_range_6
- rep0_range_7
- rep0_range_8
- rep0_range_9
- ... (144 total)
[27]:
ps.scenarios()
[27]:
[ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 0}, rangeid='', name='rep0_range_0'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 1}, rangeid='', name='rep0_range_1'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 2}, rangeid='', name='rep0_range_2'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 3}, rangeid='', name='rep0_range_3'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 4}, rangeid='', name='rep0_range_4'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 5}, rangeid='', name='rep0_range_5'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 6}, rangeid='', name='rep0_range_6'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 7}, rangeid='', name='rep0_range_7'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 8}, rangeid='', name='rep0_range_8'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 9}, rangeid='', name='rep0_range_9'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 10}, rangeid='', name='rep0_range_10'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 0, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 0, 1: 11}, rangeid='', name='rep0_range_11'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 0}, rangeid='', name='rep0_range_12'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 1}, rangeid='', name='rep0_range_13'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 2}, rangeid='', name='rep0_range_14'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 3}, rangeid='', name='rep0_range_15'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 4}, rangeid='', name='rep0_range_16'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 5}, rangeid='', name='rep0_range_17'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 6}, rangeid='', name='rep0_range_18'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 7}, rangeid='', name='rep0_range_19'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 8}, rangeid='', name='rep0_range_20'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 9}, rangeid='', name='rep0_range_21'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 10}, rangeid='', name='rep0_range_22'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 1, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 1, 1: 11}, rangeid='', name='rep0_range_23'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 0}, rangeid='', name='rep0_range_24'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 1}, rangeid='', name='rep0_range_25'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 2}, rangeid='', name='rep0_range_26'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 3}, rangeid='', name='rep0_range_27'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 4}, rangeid='', name='rep0_range_28'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 5}, rangeid='', name='rep0_range_29'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 6}, rangeid='', name='rep0_range_30'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 7}, rangeid='', name='rep0_range_31'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 8}, rangeid='', name='rep0_range_32'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 9}, rangeid='', name='rep0_range_33'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 10}, rangeid='', name='rep0_range_34'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 2, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 2, 1: 11}, rangeid='', name='rep0_range_35'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 0}, rangeid='', name='rep0_range_36'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 1}, rangeid='', name='rep0_range_37'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 2}, rangeid='', name='rep0_range_38'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 3}, rangeid='', name='rep0_range_39'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 4}, rangeid='', name='rep0_range_40'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 5}, rangeid='', name='rep0_range_41'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 6}, rangeid='', name='rep0_range_42'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 7}, rangeid='', name='rep0_range_43'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 8}, rangeid='', name='rep0_range_44'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 9}, rangeid='', name='rep0_range_45'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 10}, rangeid='', name='rep0_range_46'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 3, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 3, 1: 11}, rangeid='', name='rep0_range_47'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 0}, rangeid='', name='rep0_range_48'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 1}, rangeid='', name='rep0_range_49'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 2}, rangeid='', name='rep0_range_50'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 3}, rangeid='', name='rep0_range_51'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 4}, rangeid='', name='rep0_range_52'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 5}, rangeid='', name='rep0_range_53'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 6}, rangeid='', name='rep0_range_54'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 7}, rangeid='', name='rep0_range_55'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 8}, rangeid='', name='rep0_range_56'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 9}, rangeid='', name='rep0_range_57'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 10}, rangeid='', name='rep0_range_58'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 4, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 4, 1: 11}, rangeid='', name='rep0_range_59'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 0}, rangeid='', name='rep0_range_60'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 1}, rangeid='', name='rep0_range_61'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 2}, rangeid='', name='rep0_range_62'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 3}, rangeid='', name='rep0_range_63'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 4}, rangeid='', name='rep0_range_64'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 5}, rangeid='', name='rep0_range_65'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 6}, rangeid='', name='rep0_range_66'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 7}, rangeid='', name='rep0_range_67'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 8}, rangeid='', name='rep0_range_68'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 9}, rangeid='', name='rep0_range_69'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 10}, rangeid='', name='rep0_range_70'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 5, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 5, 1: 11}, rangeid='', name='rep0_range_71'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 0}, rangeid='', name='rep0_range_72'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 1}, rangeid='', name='rep0_range_73'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 2}, rangeid='', name='rep0_range_74'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 3}, rangeid='', name='rep0_range_75'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 4}, rangeid='', name='rep0_range_76'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 5}, rangeid='', name='rep0_range_77'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 6}, rangeid='', name='rep0_range_78'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 7}, rangeid='', name='rep0_range_79'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 8}, rangeid='', name='rep0_range_80'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 9}, rangeid='', name='rep0_range_81'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 10}, rangeid='', name='rep0_range_82'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 6, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 6, 1: 11}, rangeid='', name='rep0_range_83'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 0}, rangeid='', name='rep0_range_84'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 1}, rangeid='', name='rep0_range_85'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 2}, rangeid='', name='rep0_range_86'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 3}, rangeid='', name='rep0_range_87'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 4}, rangeid='', name='rep0_range_88'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 5}, rangeid='', name='rep0_range_89'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 6}, rangeid='', name='rep0_range_90'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 7}, rangeid='', name='rep0_range_91'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 8}, rangeid='', name='rep0_range_92'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 9}, rangeid='', name='rep0_range_93'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 10}, rangeid='', name='rep0_range_94'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 7, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 7, 1: 11}, rangeid='', name='rep0_range_95'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 0}, rangeid='', name='rep0_range_96'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 1}, rangeid='', name='rep0_range_97'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 2}, rangeid='', name='rep0_range_98'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 3}, rangeid='', name='rep0_range_99'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 4}, rangeid='', name='rep0_range_100'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 5}, rangeid='', name='rep0_range_101'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 6}, rangeid='', name='rep0_range_102'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 7}, rangeid='', name='rep0_range_103'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 8}, rangeid='', name='rep0_range_104'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 9}, rangeid='', name='rep0_range_105'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 10}, rangeid='', name='rep0_range_106'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 8, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 8, 1: 11}, rangeid='', name='rep0_range_107'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 0}, rangeid='', name='rep0_range_108'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 1}, rangeid='', name='rep0_range_109'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 2}, rangeid='', name='rep0_range_110'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 3}, rangeid='', name='rep0_range_111'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 4}, rangeid='', name='rep0_range_112'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 5}, rangeid='', name='rep0_range_113'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 6}, rangeid='', name='rep0_range_114'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 7}, rangeid='', name='rep0_range_115'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 8}, rangeid='', name='rep0_range_116'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 9}, rangeid='', name='rep0_range_117'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 10}, rangeid='', name='rep0_range_118'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 9, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 9, 1: 11}, rangeid='', name='rep0_range_119'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 0}, rangeid='', name='rep0_range_120'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 1}, rangeid='', name='rep0_range_121'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 2}, rangeid='', name='rep0_range_122'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 3}, rangeid='', name='rep0_range_123'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 4}, rangeid='', name='rep0_range_124'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 5}, rangeid='', name='rep0_range_125'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 6}, rangeid='', name='rep0_range_126'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 7}, rangeid='', name='rep0_range_127'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 8}, rangeid='', name='rep0_range_128'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 9}, rangeid='', name='rep0_range_129'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 10}, rangeid='', name='rep0_range_130'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 10, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 10, 1: 11}, rangeid='', name='rep0_range_131'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 0}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 0}, rangeid='', name='rep0_range_132'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 1}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 1}, rangeid='', name='rep0_range_133'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 2}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 2}, rangeid='', name='rep0_range_134'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 3}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 3}, rangeid='', name='rep0_range_135'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 4}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 4}, rangeid='', name='rep0_range_136'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 5}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 5}, rangeid='', name='rep0_range_137'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 6}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 6}, rangeid='', name='rep0_range_138'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 7}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 7}, rangeid='', name='rep0_range_139'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 8}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 8}, rangeid='', name='rep0_range_140'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 9}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 9}, rangeid='', name='rep0_range_141'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 10}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 10}, rangeid='', name='rep0_range_142'),
ParameterScenario(sequence={}, times=(), p={'psfs': {'fatigue': 11, 'attention': 11}}, r={}, sp={}, prob=0.006944444444444444, inputparams={0: 11, 1: 11}, rangeid='', name='rep0_range_143')]
[28]:
ers, hists = prop.parameter_sample(mdl, ps)
SCENARIOS COMPLETE: 100%|██████████| 144/144 [01:05<00:00, 2.19it/s]
[29]:
plot_map(mdl, hists)
[29]:
(<Figure size 400x400 with 1 Axes>, <Axes: xlabel='x', ylabel='y'>)
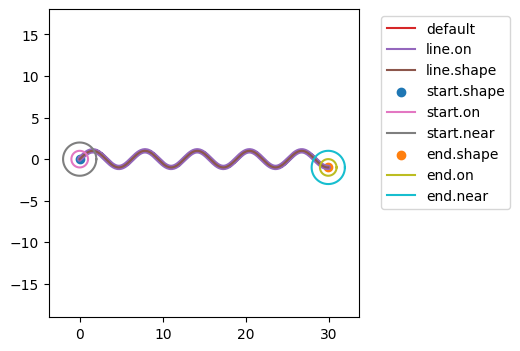
[30]:
ers.get_values("end_x")
[30]:
rep0_range_0.endclass.end_x: 29.254775331608617
rep0_range_1.endclass.end_x: 29.254775331608617
rep0_range_2.endclass.end_x: 29.254775331608617
rep0_range_3.endclass.end_x: 29.254775331608617
rep0_range_4.endclass.end_x: 29.254775331608617
rep0_range_5.endclass.end_x: 29.254775331608617
rep0_range_6.endclass.end_x: 29.254775331608617
rep0_range_7.endclass.end_x: 29.254775331608617
rep0_range_8.endclass.end_x: 29.254775331608617
rep0_range_9.endclass.end_x: 29.254775331608617
rep0_range_10.endclass.end_x: 29.254775331608617
rep0_range_11.endclass.end_x: 29.254775331608617
rep0_range_12.endclass.end_x: 29.254775331608617
rep0_range_13.endclass.end_x: 29.254775331608617
rep0_range_14.endclass.end_x: 29.254775331608617
rep0_range_15.endclass.end_x: 29.254775331608617
rep0_range_16.endclass.end_x: 29.254775331608617
rep0_range_17.endclass.end_x: 29.254775331608617
rep0_range_18.endclass.end_x: 29.254775331608617
rep0_range_19.endclass.end_x: 29.254775331608617
rep0_range_20.endclass.end_x: 29.254775331608617
rep0_range_21.endclass.end_x: 29.254775331608617
rep0_range_22.endclass.end_x: 29.254775331608617
rep0_range_23.endclass.end_x: 29.254775331608617
rep0_range_24.endclass.end_x: 29.254775331608617
rep0_range_25.endclass.end_x: 29.254775331608617
rep0_range_26.endclass.end_x: 29.254775331608617
rep0_range_27.endclass.end_x: 29.254775331608617
rep0_range_28.endclass.end_x: 29.254775331608617
rep0_range_29.endclass.end_x: 29.254775331608617
rep0_range_30.endclass.end_x: 29.254775331608617
rep0_range_31.endclass.end_x: 29.254775331608617
rep0_range_32.endclass.end_x: 29.254775331608617
rep0_range_33.endclass.end_x: 29.254775331608617
rep0_range_34.endclass.end_x: 29.254775331608617
rep0_range_35.endclass.end_x: 29.254775331608617
rep0_range_36.endclass.end_x: 29.254775331608617
rep0_range_37.endclass.end_x: 29.254775331608617
rep0_range_38.endclass.end_x: 29.254775331608617
rep0_range_39.endclass.end_x: 29.254775331608617
rep0_range_40.endclass.end_x: 29.254775331608617
rep0_range_41.endclass.end_x: 29.254775331608617
rep0_range_42.endclass.end_x: 29.254775331608617
rep0_range_43.endclass.end_x: 29.254775331608617
rep0_range_44.endclass.end_x: 29.254775331608617
rep0_range_45.endclass.end_x: 29.254775331608617
rep0_range_46.endclass.end_x: 29.254775331608617
rep0_range_47.endclass.end_x: 29.254775331608617
rep0_range_48.endclass.end_x: 29.254775331608617
rep0_range_49.endclass.end_x: 29.254775331608617
rep0_range_50.endclass.end_x: 29.254775331608617
rep0_range_51.endclass.end_x: 29.254775331608617
rep0_range_52.endclass.end_x: 29.254775331608617
rep0_range_53.endclass.end_x: 29.254775331608617
rep0_range_54.endclass.end_x: 29.254775331608617
rep0_range_55.endclass.end_x: 29.254775331608617
rep0_range_56.endclass.end_x: 29.254775331608617
rep0_range_57.endclass.end_x: 29.254775331608617
rep0_range_58.endclass.end_x: 29.254775331608617
rep0_range_59.endclass.end_x: 29.254775331608617
rep0_range_60.endclass.end_x: 29.254775331608617
rep0_range_61.endclass.end_x: 29.254775331608617
rep0_range_62.endclass.end_x: 29.254775331608617
rep0_range_63.endclass.end_x: 29.254775331608617
rep0_range_64.endclass.end_x: 29.254775331608617
rep0_range_65.endclass.end_x: 29.254775331608617
rep0_range_66.endclass.end_x: 29.254775331608617
rep0_range_67.endclass.end_x: 29.254775331608617
rep0_range_68.endclass.end_x: 29.254775331608617
rep0_range_69.endclass.end_x: 29.254775331608617
rep0_range_70.endclass.end_x: 29.254775331608617
rep0_range_71.endclass.end_x: 29.254775331608617
rep0_range_72.endclass.end_x: 29.254775331608617
rep0_range_73.endclass.end_x: 29.254775331608617
rep0_range_74.endclass.end_x: 29.254775331608617
rep0_range_75.endclass.end_x: 29.254775331608617
rep0_range_76.endclass.end_x: 29.254775331608617
rep0_range_77.endclass.end_x: 29.254775331608617
rep0_range_78.endclass.end_x: 29.254775331608617
rep0_range_79.endclass.end_x: 29.254775331608617
rep0_range_80.endclass.end_x: 29.254775331608617
rep0_range_81.endclass.end_x: 29.254775331608617
rep0_range_82.endclass.end_x: 29.254775331608617
rep0_range_83.endclass.end_x: 29.254775331608617
rep0_range_84.endclass.end_x: 29.254775331608617
rep0_range_85.endclass.end_x: 29.254775331608617
rep0_range_86.endclass.end_x: 29.254775331608617
rep0_range_87.endclass.end_x: 29.254775331608617
rep0_range_88.endclass.end_x: 29.254775331608617
rep0_range_89.endclass.end_x: 29.254775331608617
rep0_range_90.endclass.end_x: 29.254775331608617
rep0_range_91.endclass.end_x: 29.254775331608617
rep0_range_92.endclass.end_x: 29.254775331608617
rep0_range_93.endclass.end_x: 29.254775331608617
rep0_range_94.endclass.end_x: 29.254775331608617
rep0_range_95.endclass.end_x: 29.254775331608617
rep0_range_96.endclass.end_x: 29.254775331608617
rep0_range_97.endclass.end_x: 29.254775331608617
rep0_range_98.endclass.end_x: 29.254775331608617
rep0_range_99.endclass.end_x: 29.254775331608617
rep0_range_100.endclass.end_x: 29.254775331608617
rep0_range_101.endclass.end_x: 29.254775331608617
rep0_range_102.endclass.end_x: 29.254775331608617
rep0_range_103.endclass.end_x: 29.254775331608617
rep0_range_104.endclass.end_x: 29.254775331608617
rep0_range_105.endclass.end_x: 29.254775331608617
rep0_range_106.endclass.end_x: 29.254775331608617
rep0_range_107.endclass.end_x: 29.254775331608617
rep0_range_108.endclass.end_x: 0.0
rep0_range_109.endclass.end_x: 0.0
rep0_range_110.endclass.end_x: 0.0
rep0_range_111.endclass.end_x: 0.0
rep0_range_112.endclass.end_x: 0.0
rep0_range_113.endclass.end_x: 0.0
rep0_range_114.endclass.end_x: 0.0
rep0_range_115.endclass.end_x: 0.0
rep0_range_116.endclass.end_x: 0.0
rep0_range_117.endclass.end_x: 0.0
rep0_range_118.endclass.end_x: 0.0
rep0_range_119.endclass.end_x: 0.0
rep0_range_120.endclass.end_x: 0.0
rep0_range_121.endclass.end_x: 0.0
rep0_range_122.endclass.end_x: 0.0
rep0_range_123.endclass.end_x: 0.0
rep0_range_124.endclass.end_x: 0.0
rep0_range_125.endclass.end_x: 0.0
rep0_range_126.endclass.end_x: 0.0
rep0_range_127.endclass.end_x: 0.0
rep0_range_128.endclass.end_x: 0.0
rep0_range_129.endclass.end_x: 0.0
rep0_range_130.endclass.end_x: 0.0
rep0_range_131.endclass.end_x: 0.0
rep0_range_132.endclass.end_x: 0.0
rep0_range_133.endclass.end_x: 0.0
rep0_range_134.endclass.end_x: 0.0
rep0_range_135.endclass.end_x: 0.0
rep0_range_136.endclass.end_x: 0.0
rep0_range_137.endclass.end_x: 0.0
rep0_range_138.endclass.end_x: 0.0
rep0_range_139.endclass.end_x: 0.0
rep0_range_140.endclass.end_x: 0.0
rep0_range_141.endclass.end_x: 0.0
rep0_range_142.endclass.end_x: 0.0
rep0_range_143.endclass.end_x: 0.0
[31]:
from fmdtools.analyze.tabulate import NominalEnvelope
na = NominalEnvelope(ps, ers, 'at_finish', 'p.psfs.fatigue', 'p.psfs.attention', func= lambda x: x)
fig, ax = na.plot_scatter()
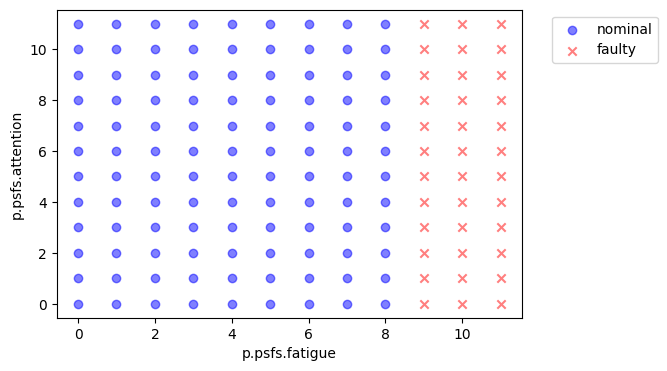
[32]:
fig.savefig("nominal_psfs.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[33]:
mdl = RoverHuman(p={'ground': {'linetype': 'turn'}})
endresults, mdlhist = prop.one_fault(mdl, 'operator.aa.acts.look', 'failed_no_action', time=15)
[34]:
endresults
[34]:
endclass.rate: 1.0
endclass.cost: 0
endclass.prob: 1.0
endclass.expected_cost: 0
endclass.in_bound: False
endclass.at_finish: False
endclass.line_dist: 1
endclass.num_modes: 1
endclass.end_dist: 23.466067426611588
endclass.tot_deviation: 0.2759017544282031
endclass.faults: array(1)
endclass.classification: incomplete mission faulty
endclass.end_x: 13.33333333333334
endclass.end_y: 0.0
endclass.endpt: array(2)
[35]:
traj_fig = plot_map(mdl, mdlhist)
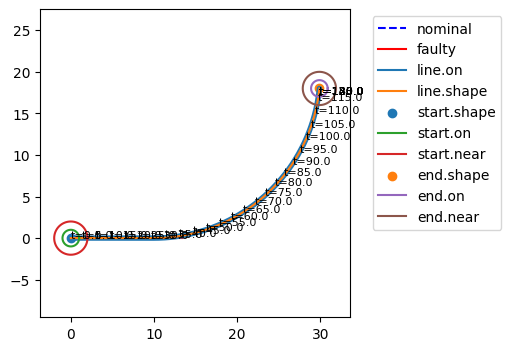
[36]:
mdlhist.plot_trajectories('flows.pos.s.x', 'flows.pos.s.y')
[36]:
(<Figure size 400x400 with 1 Axes>, <Axes: xlabel='x', ylabel='y'>)
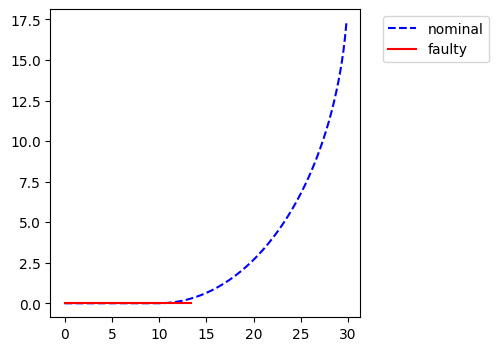
[37]:
mdlhist.plot_line('flows.pos.s.vel', 'flows.pos.s.ux', 'flows.pos.s.uy')
[37]:
(<Figure size 600x400 with 4 Axes>,
array([<Axes: title={'center': 'flows.pos.s.vel'}, xlabel=' '>,
<Axes: title={'center': 'flows.pos.s.ux'}, xlabel='time'>,
<Axes: title={'center': 'flows.pos.s.uy'}, xlabel='time'>,
<Axes: >], dtype=object))
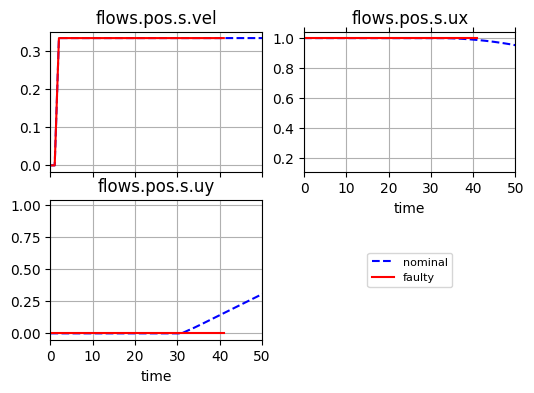
[38]:
fs = FaultSample(fd)
fs.add_fault_times([j for j in range(0, 30, 5)])
fs
[38]:
FaultSample of scenarios:
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t0
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t5
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t10
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t15
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t20
- roverhuman_fxns_operator_aa_acts_look_failed_no_action_t25
- roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t0
- roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t5
- roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t10
- roverhuman_fxns_operator_aa_acts_percieve_failed_no_action_t15
- ... (114 total)
[39]:
ers, hists = prop.fault_sample(mdl, fs)
SCENARIOS COMPLETE: 100%|██████████| 114/114 [00:30<00:00, 3.74it/s]
[40]:
fig, ax = plot_map(mdl, hists)
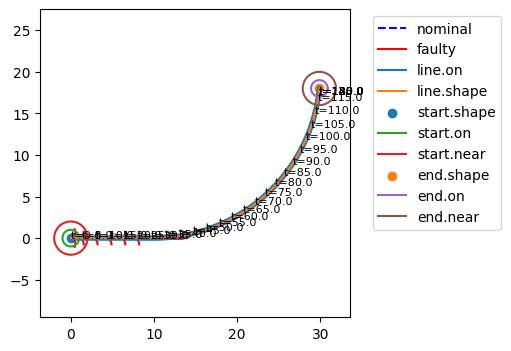
[41]:
fig.savefig("nocon_trajectories.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[42]:
fig, ax = hists.plot_line('flows.psfs.s.attention')
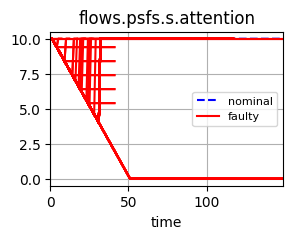
[43]:
fig.savefig("nocon_attention.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[44]:
mdl = RoverHuman(p={'ground': {'linetype': 'sine', 'amp': 2, 'period': 2/3.14*30}})
[45]:
ers, hists = prop.fault_sample(mdl, fs)
SCENARIOS COMPLETE: 100%|██████████| 114/114 [00:26<00:00, 4.28it/s]
[46]:
fig, ax = plot_map(mdl, hists)
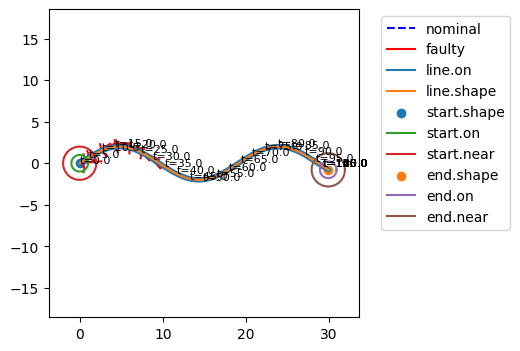
[47]:
fig.savefig("nocon_trajectories_sine.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[48]:
fig, ax = hists.plot_line('flows.psfs.s.attention')
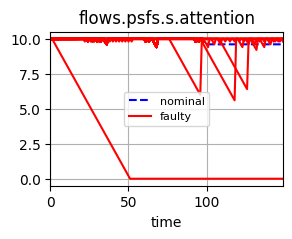
[49]:
fig.savefig("nocon_attention_sine.pdf", format="pdf", bbox_inches = 'tight', pad_inches = 0)
[ ]: