Rover Model Explanation and Preliminaries
This notebook covers the setup of a lane-following rover fault model for understanding the effects of faults in AI-driven systems. This model uses the fmdtools simulation toolkit to simulate the nominal and faulty behaviors of the rover over a set of fault scenarios and classify/assess risk.
[1]:
import fmdtools.sim.propagate as prop
import multiprocessing as mp
This model is defined in rover_model.py
. - Rover
is the class defining the model, - RoverParam
is the class used to generate model parameters (given design variables), and - plot_map
is used to visualize the trajectory of the rover over time
[2]:
from rover_model import Rover, RoverParam, GroundParam
Model Setup
[3]:
mdl = Rover()
The functions and flows of the model are shown below, and were taken from the design of the system:
[4]:
from fmdtools.analyze.graph import FunctionArchitectureFxnGraph
mg = FunctionArchitectureFxnGraph(mdl)
fig, ax = mg.draw()
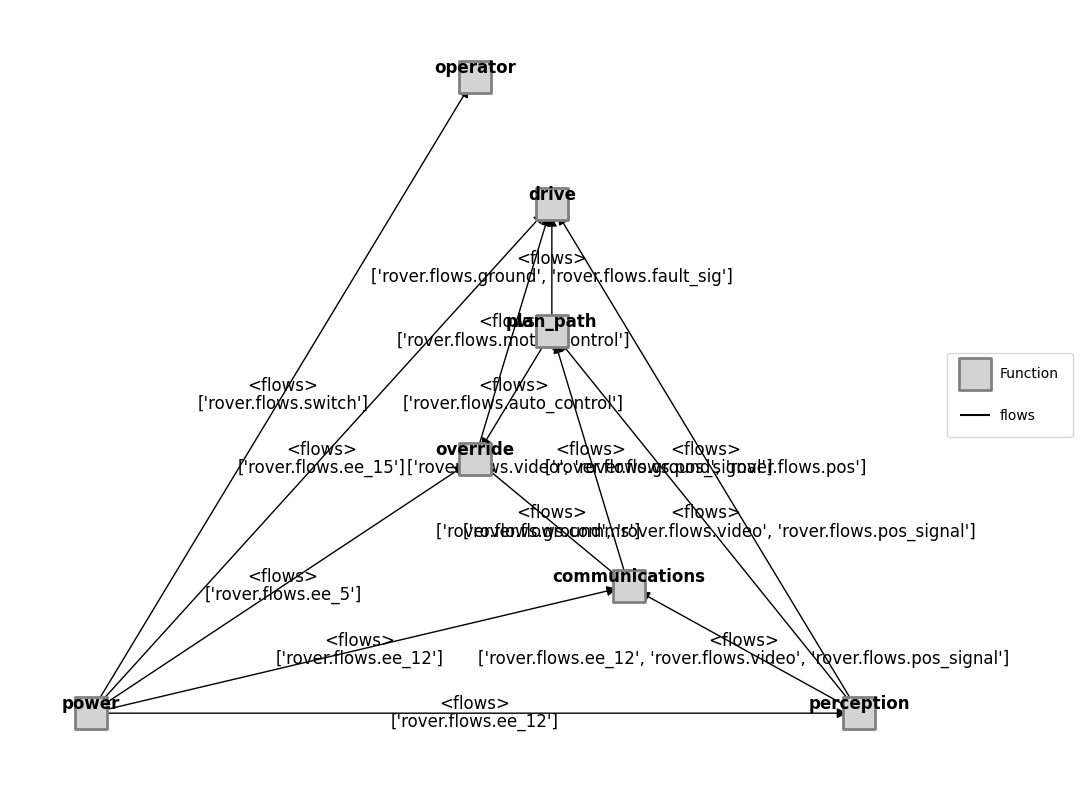
The functions are: - Operator
, the operator of the rover who turns it on/off and takes control if needed (not fully implemented) - Power
, the battery and power supply - Communications
, the radio used to relay back position/etc. to the operator - Avionics
, the computer/control system which determines where to direct the given position information - Override
, the system that enables the operator to control the rover instead - Perception
, the camera/AI system which percieves
the ground and then (not fully implemented) - Environment
, which defines the lines and/or changes to the environment based on environmental conditions and/or the movement of the system
The flows are: - Control
, the physical interactions between the operator and the rover (i.e. on/off) - Comms
, the communications between the operator and the rover (position, video, etc.) - OverrideComms
, the communications between the operator and the - Pos_Signal
, the rover’s internal idea of its position and velocity - EE5
, EE12
, EE15
, the electrical power provided by the battery - AvionicsControl
, the contol signals from the avionics to the drive system -
Video
, the video feed/signal from the camera to the avionics - MotorControl
, the control signals to the drive system(s) - Ground
, the real position of the rover and the ground/environment/etc.
The model is presently a mixed static/dynamic propagation model in which most function behaviors propagate over time, except for the power system, which propagates in a dynamic step. This may change depending on the necessity to model interactions between these systems. This is shown below.
[5]:
mg.set_exec_order(mdl)
fig, ax = mg.draw()
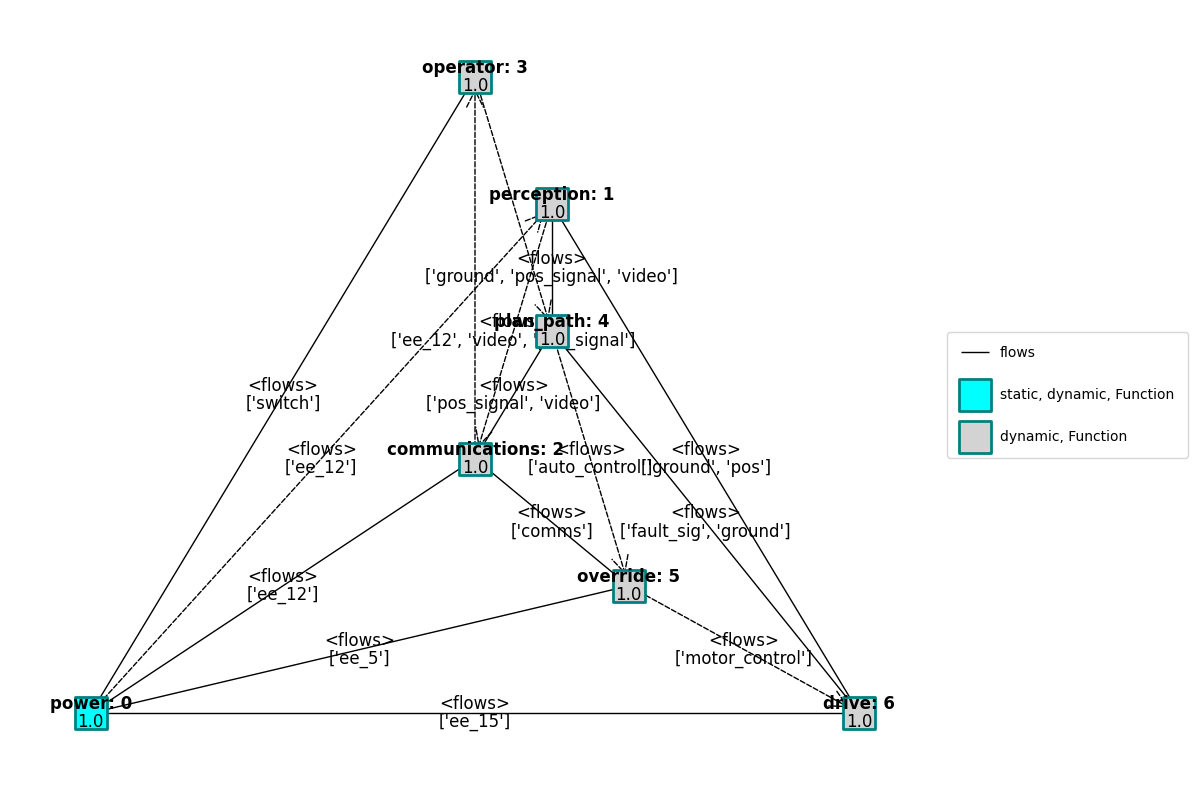
The run order for this model is additionally shown below.
[6]:
mdl.plot_dynamic_run_order(rotateticks=True)
[6]:
(<Figure size 640x480 with 1 Axes>, <Axes: >)
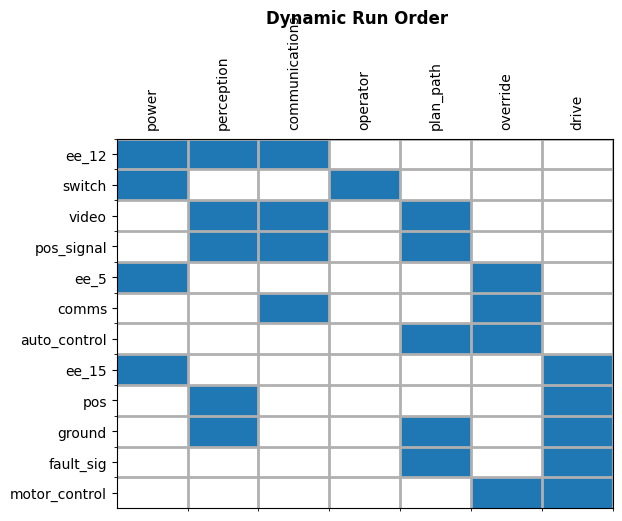
Model simulation
Currently, the model can be simulated in a few situations for lane-tracking: a sine wave and a 90-degree turn.
Below shows the 90-degree turn. As shown, the centerline tracking is not perfect, with a fairly large deviation in distance (current limit for viewing the line in the model is 1 meter). This should be controlled in the future by lowering the timestep (enabling more corrections) or enabling some reduction to velocity when going around corners.
[7]:
mdl = Rover(p=RoverParam(ground = GroundParam(linetype='turn')))
endresults, mdlhist = prop.nominal(mdl)
mdlhist.plot_trajectories('pos.s.x', 'pos.s.y')
[7]:
(<Figure size 400x400 with 1 Axes>, <Axes: >)
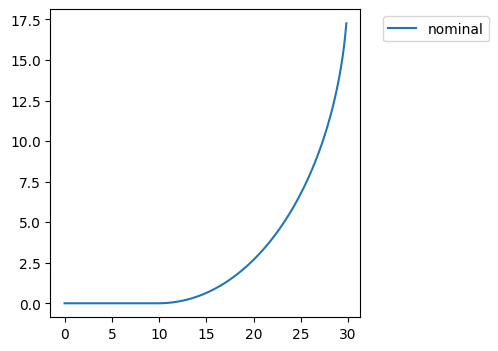
[8]:
mdlhist['time']
[8]:
array([ 0., 1., 2., 3., 4., 5., 6., 7., 8., 9., 10.,
11., 12., 13., 14., 15., 16., 17., 18., 19., 20., 21.,
22., 23., 24., 25., 26., 27., 28., 29., 30., 31., 32.,
33., 34., 35., 36., 37., 38., 39., 40., 41., 42., 43.,
44., 45., 46., 47., 48., 49., 50., 51., 52., 53., 54.,
55., 56., 57., 58., 59., 60., 61., 62., 63., 64., 65.,
66., 67., 68., 69., 70., 71., 72., 73., 74., 75., 76.,
77., 78., 79., 80., 81., 82., 83., 84., 85., 86., 87.,
88., 89., 90., 91., 92., 93., 94., 95., 96., 97., 98.,
99., 100., 101., 102., 103., 104., 105., 106., 107., 108., 109.,
110., 111., 112., 113., 114., 115., 116., 117., 118., 119., 120.])
Below shows the sine wave. As shown, this curve has a relatively low amplitude (see the y-axis), which results in low tracking error.
[9]:
mdl = Rover(p=RoverParam(ground = GroundParam(linetype='sine')))
endresults, mdlhist = prop.nominal(mdl)
mdlhist.plot_trajectories('pos.s.x', 'pos.s.y')
[9]:
(<Figure size 400x400 with 1 Axes>, <Axes: >)
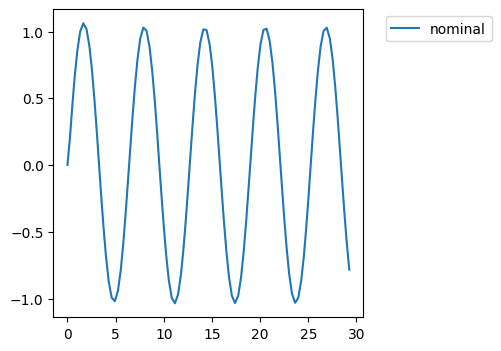
[10]:
mdlhist['time']
[10]:
array([ 0., 1., 2., 3., 4., 5., 6., 7., 8., 9., 10.,
11., 12., 13., 14., 15., 16., 17., 18., 19., 20., 21.,
22., 23., 24., 25., 26., 27., 28., 29., 30., 31., 32.,
33., 34., 35., 36., 37., 38., 39., 40., 41., 42., 43.,
44., 45., 46., 47., 48., 49., 50., 51., 52., 53., 54.,
55., 56., 57., 58., 59., 60., 61., 62., 63., 64., 65.,
66., 67., 68., 69., 70., 71., 72., 73., 74., 75., 76.,
77., 78., 79., 80., 81., 82., 83., 84., 85., 86., 87.,
88., 89., 90., 91., 92., 93., 94., 95., 96., 97., 98.,
99., 100., 101., 102., 103., 104., 105., 106., 107., 108., 109.,
110., 111., 112.])
The performance of the rover in these situations is dependent on the parameters of the situation (e.g., the radius of the curve and the amplitude of the sine wave). Thus, it is important to define the operational envelope for the system. This can be done using a ParameterSample
and ParameterDomain
, which can be used to define ranges of variables to simulate the system under.
[11]:
from fmdtools.sim.sample import ParameterDomain, ParameterSample
pd_turn = ParameterDomain(RoverParam)
pd_turn.add_constant("ground.linetype", "turn")
pd_turn.add_variables("ground.radius", "ground.x_start", lims={"ground.radius": (5, 25), "ground.x_start": (0, 5)})
pd_turn
[11]:
ParameterDomain with:
- variables: {'ground.radius': (5, 25), 'ground.x_start': (0, 5)}
- constants: {'ground.linetype': 'turn'}
- parameter_initializer: RoverParam
[12]:
pd_sine = ParameterDomain(RoverParam)
pd_sine.add_constant("ground.linetype", "sine")
pd_sine.add_variables("ground.amp", "ground.period", lims={"ground.amp":(0, 8), "ground.period": (10, 50)})
pd_sine
[12]:
ParameterDomain with:
- variables: {'ground.amp': (0, 8), 'ground.period': (10, 50)}
- constants: {'ground.linetype': 'sine'}
- parameter_initializer: RoverParam
In this sample we then sample two major situations–a wavelength and amplitude for the sine wave, and a radius and start location for the turn.
[13]:
ps_sine = ParameterSample(pd_sine)
ps_sine.add_variable_ranges(comb_kwargs={'resolutions':{'ground.amp': 0.2, "ground.period": 10}})
ps_sine.scenarios()
[13]:
[ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.0, 1: 10}, rangeid='', name='rep0_range_0'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.0, 1: 20}, rangeid='', name='rep0_range_1'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.0, 1: 30}, rangeid='', name='rep0_range_2'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.0, 1: 40}, rangeid='', name='rep0_range_3'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.0, 1: 50}, rangeid='', name='rep0_range_4'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.2, 1: 10}, rangeid='', name='rep0_range_5'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.2, 1: 20}, rangeid='', name='rep0_range_6'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.2, 1: 30}, rangeid='', name='rep0_range_7'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.2, 1: 40}, rangeid='', name='rep0_range_8'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.2, 1: 50}, rangeid='', name='rep0_range_9'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.4, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.4, 1: 10}, rangeid='', name='rep0_range_10'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.4, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.4, 1: 20}, rangeid='', name='rep0_range_11'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.4, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.4, 1: 30}, rangeid='', name='rep0_range_12'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.4, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.4, 1: 40}, rangeid='', name='rep0_range_13'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.4, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.4, 1: 50}, rangeid='', name='rep0_range_14'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.6000000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.6000000000000001, 1: 10}, rangeid='', name='rep0_range_15'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.6000000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.6000000000000001, 1: 20}, rangeid='', name='rep0_range_16'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.6000000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.6000000000000001, 1: 30}, rangeid='', name='rep0_range_17'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.6000000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.6000000000000001, 1: 40}, rangeid='', name='rep0_range_18'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.6000000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.6000000000000001, 1: 50}, rangeid='', name='rep0_range_19'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.8, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.8, 1: 10}, rangeid='', name='rep0_range_20'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.8, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.8, 1: 20}, rangeid='', name='rep0_range_21'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.8, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.8, 1: 30}, rangeid='', name='rep0_range_22'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.8, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.8, 1: 40}, rangeid='', name='rep0_range_23'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 0.8, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 0.8, 1: 50}, rangeid='', name='rep0_range_24'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.0, 1: 10}, rangeid='', name='rep0_range_25'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.0, 1: 20}, rangeid='', name='rep0_range_26'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.0, 1: 30}, rangeid='', name='rep0_range_27'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.0, 1: 40}, rangeid='', name='rep0_range_28'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.0, 1: 50}, rangeid='', name='rep0_range_29'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.2000000000000002, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.2000000000000002, 1: 10}, rangeid='', name='rep0_range_30'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.2000000000000002, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.2000000000000002, 1: 20}, rangeid='', name='rep0_range_31'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.2000000000000002, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.2000000000000002, 1: 30}, rangeid='', name='rep0_range_32'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.2000000000000002, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.2000000000000002, 1: 40}, rangeid='', name='rep0_range_33'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.2000000000000002, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.2000000000000002, 1: 50}, rangeid='', name='rep0_range_34'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.4000000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.4000000000000001, 1: 10}, rangeid='', name='rep0_range_35'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.4000000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.4000000000000001, 1: 20}, rangeid='', name='rep0_range_36'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.4000000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.4000000000000001, 1: 30}, rangeid='', name='rep0_range_37'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.4000000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.4000000000000001, 1: 40}, rangeid='', name='rep0_range_38'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.4000000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.4000000000000001, 1: 50}, rangeid='', name='rep0_range_39'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.6, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.6, 1: 10}, rangeid='', name='rep0_range_40'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.6, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.6, 1: 20}, rangeid='', name='rep0_range_41'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.6, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.6, 1: 30}, rangeid='', name='rep0_range_42'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.6, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.6, 1: 40}, rangeid='', name='rep0_range_43'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.6, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.6, 1: 50}, rangeid='', name='rep0_range_44'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.8, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.8, 1: 10}, rangeid='', name='rep0_range_45'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.8, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.8, 1: 20}, rangeid='', name='rep0_range_46'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.8, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.8, 1: 30}, rangeid='', name='rep0_range_47'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.8, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.8, 1: 40}, rangeid='', name='rep0_range_48'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 1.8, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 1.8, 1: 50}, rangeid='', name='rep0_range_49'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.0, 1: 10}, rangeid='', name='rep0_range_50'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.0, 1: 20}, rangeid='', name='rep0_range_51'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.0, 1: 30}, rangeid='', name='rep0_range_52'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.0, 1: 40}, rangeid='', name='rep0_range_53'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.0, 1: 50}, rangeid='', name='rep0_range_54'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.2, 1: 10}, rangeid='', name='rep0_range_55'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.2, 1: 20}, rangeid='', name='rep0_range_56'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.2, 1: 30}, rangeid='', name='rep0_range_57'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.2, 1: 40}, rangeid='', name='rep0_range_58'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.2, 1: 50}, rangeid='', name='rep0_range_59'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.4000000000000004, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.4000000000000004, 1: 10}, rangeid='', name='rep0_range_60'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.4000000000000004, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.4000000000000004, 1: 20}, rangeid='', name='rep0_range_61'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.4000000000000004, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.4000000000000004, 1: 30}, rangeid='', name='rep0_range_62'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.4000000000000004, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.4000000000000004, 1: 40}, rangeid='', name='rep0_range_63'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.4000000000000004, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.4000000000000004, 1: 50}, rangeid='', name='rep0_range_64'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.6, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.6, 1: 10}, rangeid='', name='rep0_range_65'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.6, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.6, 1: 20}, rangeid='', name='rep0_range_66'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.6, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.6, 1: 30}, rangeid='', name='rep0_range_67'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.6, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.6, 1: 40}, rangeid='', name='rep0_range_68'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.6, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.6, 1: 50}, rangeid='', name='rep0_range_69'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.8000000000000003, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.8000000000000003, 1: 10}, rangeid='', name='rep0_range_70'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.8000000000000003, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.8000000000000003, 1: 20}, rangeid='', name='rep0_range_71'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.8000000000000003, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.8000000000000003, 1: 30}, rangeid='', name='rep0_range_72'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.8000000000000003, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.8000000000000003, 1: 40}, rangeid='', name='rep0_range_73'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 2.8000000000000003, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 2.8000000000000003, 1: 50}, rangeid='', name='rep0_range_74'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.0, 1: 10}, rangeid='', name='rep0_range_75'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.0, 1: 20}, rangeid='', name='rep0_range_76'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.0, 1: 30}, rangeid='', name='rep0_range_77'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.0, 1: 40}, rangeid='', name='rep0_range_78'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.0, 1: 50}, rangeid='', name='rep0_range_79'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.2, 1: 10}, rangeid='', name='rep0_range_80'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.2, 1: 20}, rangeid='', name='rep0_range_81'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.2, 1: 30}, rangeid='', name='rep0_range_82'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.2, 1: 40}, rangeid='', name='rep0_range_83'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.2, 1: 50}, rangeid='', name='rep0_range_84'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.4000000000000004, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.4000000000000004, 1: 10}, rangeid='', name='rep0_range_85'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.4000000000000004, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.4000000000000004, 1: 20}, rangeid='', name='rep0_range_86'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.4000000000000004, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.4000000000000004, 1: 30}, rangeid='', name='rep0_range_87'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.4000000000000004, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.4000000000000004, 1: 40}, rangeid='', name='rep0_range_88'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.4000000000000004, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.4000000000000004, 1: 50}, rangeid='', name='rep0_range_89'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.6, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.6, 1: 10}, rangeid='', name='rep0_range_90'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.6, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.6, 1: 20}, rangeid='', name='rep0_range_91'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.6, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.6, 1: 30}, rangeid='', name='rep0_range_92'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.6, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.6, 1: 40}, rangeid='', name='rep0_range_93'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.6, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.6, 1: 50}, rangeid='', name='rep0_range_94'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.8000000000000003, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.8000000000000003, 1: 10}, rangeid='', name='rep0_range_95'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.8000000000000003, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.8000000000000003, 1: 20}, rangeid='', name='rep0_range_96'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.8000000000000003, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.8000000000000003, 1: 30}, rangeid='', name='rep0_range_97'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.8000000000000003, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.8000000000000003, 1: 40}, rangeid='', name='rep0_range_98'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 3.8000000000000003, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 3.8000000000000003, 1: 50}, rangeid='', name='rep0_range_99'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.0, 1: 10}, rangeid='', name='rep0_range_100'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.0, 1: 20}, rangeid='', name='rep0_range_101'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.0, 1: 30}, rangeid='', name='rep0_range_102'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.0, 1: 40}, rangeid='', name='rep0_range_103'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.0, 1: 50}, rangeid='', name='rep0_range_104'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.2, 1: 10}, rangeid='', name='rep0_range_105'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.2, 1: 20}, rangeid='', name='rep0_range_106'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.2, 1: 30}, rangeid='', name='rep0_range_107'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.2, 1: 40}, rangeid='', name='rep0_range_108'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.2, 1: 50}, rangeid='', name='rep0_range_109'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.4, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.4, 1: 10}, rangeid='', name='rep0_range_110'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.4, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.4, 1: 20}, rangeid='', name='rep0_range_111'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.4, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.4, 1: 30}, rangeid='', name='rep0_range_112'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.4, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.4, 1: 40}, rangeid='', name='rep0_range_113'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.4, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.4, 1: 50}, rangeid='', name='rep0_range_114'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.6000000000000005, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.6000000000000005, 1: 10}, rangeid='', name='rep0_range_115'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.6000000000000005, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.6000000000000005, 1: 20}, rangeid='', name='rep0_range_116'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.6000000000000005, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.6000000000000005, 1: 30}, rangeid='', name='rep0_range_117'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.6000000000000005, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.6000000000000005, 1: 40}, rangeid='', name='rep0_range_118'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.6000000000000005, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.6000000000000005, 1: 50}, rangeid='', name='rep0_range_119'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.800000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.800000000000001, 1: 10}, rangeid='', name='rep0_range_120'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.800000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.800000000000001, 1: 20}, rangeid='', name='rep0_range_121'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.800000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.800000000000001, 1: 30}, rangeid='', name='rep0_range_122'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.800000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.800000000000001, 1: 40}, rangeid='', name='rep0_range_123'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 4.800000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 4.800000000000001, 1: 50}, rangeid='', name='rep0_range_124'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.0, 1: 10}, rangeid='', name='rep0_range_125'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.0, 1: 20}, rangeid='', name='rep0_range_126'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.0, 1: 30}, rangeid='', name='rep0_range_127'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.0, 1: 40}, rangeid='', name='rep0_range_128'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.0, 1: 50}, rangeid='', name='rep0_range_129'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.2, 1: 10}, rangeid='', name='rep0_range_130'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.2, 1: 20}, rangeid='', name='rep0_range_131'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.2, 1: 30}, rangeid='', name='rep0_range_132'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.2, 1: 40}, rangeid='', name='rep0_range_133'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.2, 1: 50}, rangeid='', name='rep0_range_134'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.4, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.4, 1: 10}, rangeid='', name='rep0_range_135'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.4, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.4, 1: 20}, rangeid='', name='rep0_range_136'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.4, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.4, 1: 30}, rangeid='', name='rep0_range_137'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.4, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.4, 1: 40}, rangeid='', name='rep0_range_138'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.4, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.4, 1: 50}, rangeid='', name='rep0_range_139'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.6000000000000005, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.6000000000000005, 1: 10}, rangeid='', name='rep0_range_140'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.6000000000000005, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.6000000000000005, 1: 20}, rangeid='', name='rep0_range_141'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.6000000000000005, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.6000000000000005, 1: 30}, rangeid='', name='rep0_range_142'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.6000000000000005, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.6000000000000005, 1: 40}, rangeid='', name='rep0_range_143'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.6000000000000005, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.6000000000000005, 1: 50}, rangeid='', name='rep0_range_144'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.800000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.800000000000001, 1: 10}, rangeid='', name='rep0_range_145'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.800000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.800000000000001, 1: 20}, rangeid='', name='rep0_range_146'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.800000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.800000000000001, 1: 30}, rangeid='', name='rep0_range_147'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.800000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.800000000000001, 1: 40}, rangeid='', name='rep0_range_148'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 5.800000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 5.800000000000001, 1: 50}, rangeid='', name='rep0_range_149'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.0, 1: 10}, rangeid='', name='rep0_range_150'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.0, 1: 20}, rangeid='', name='rep0_range_151'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.0, 1: 30}, rangeid='', name='rep0_range_152'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.0, 1: 40}, rangeid='', name='rep0_range_153'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.0, 1: 50}, rangeid='', name='rep0_range_154'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.2, 1: 10}, rangeid='', name='rep0_range_155'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.2, 1: 20}, rangeid='', name='rep0_range_156'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.2, 1: 30}, rangeid='', name='rep0_range_157'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.2, 1: 40}, rangeid='', name='rep0_range_158'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.2, 1: 50}, rangeid='', name='rep0_range_159'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.4, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.4, 1: 10}, rangeid='', name='rep0_range_160'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.4, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.4, 1: 20}, rangeid='', name='rep0_range_161'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.4, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.4, 1: 30}, rangeid='', name='rep0_range_162'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.4, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.4, 1: 40}, rangeid='', name='rep0_range_163'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.4, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.4, 1: 50}, rangeid='', name='rep0_range_164'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.6000000000000005, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.6000000000000005, 1: 10}, rangeid='', name='rep0_range_165'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.6000000000000005, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.6000000000000005, 1: 20}, rangeid='', name='rep0_range_166'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.6000000000000005, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.6000000000000005, 1: 30}, rangeid='', name='rep0_range_167'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.6000000000000005, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.6000000000000005, 1: 40}, rangeid='', name='rep0_range_168'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.6000000000000005, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.6000000000000005, 1: 50}, rangeid='', name='rep0_range_169'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.800000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.800000000000001, 1: 10}, rangeid='', name='rep0_range_170'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.800000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.800000000000001, 1: 20}, rangeid='', name='rep0_range_171'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.800000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.800000000000001, 1: 30}, rangeid='', name='rep0_range_172'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.800000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.800000000000001, 1: 40}, rangeid='', name='rep0_range_173'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 6.800000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 6.800000000000001, 1: 50}, rangeid='', name='rep0_range_174'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.0, 1: 10}, rangeid='', name='rep0_range_175'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.0, 1: 20}, rangeid='', name='rep0_range_176'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.0, 1: 30}, rangeid='', name='rep0_range_177'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.0, 1: 40}, rangeid='', name='rep0_range_178'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.0, 1: 50}, rangeid='', name='rep0_range_179'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.2, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.2, 1: 10}, rangeid='', name='rep0_range_180'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.2, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.2, 1: 20}, rangeid='', name='rep0_range_181'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.2, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.2, 1: 30}, rangeid='', name='rep0_range_182'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.2, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.2, 1: 40}, rangeid='', name='rep0_range_183'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.2, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.2, 1: 50}, rangeid='', name='rep0_range_184'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.4, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.4, 1: 10}, rangeid='', name='rep0_range_185'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.4, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.4, 1: 20}, rangeid='', name='rep0_range_186'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.4, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.4, 1: 30}, rangeid='', name='rep0_range_187'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.4, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.4, 1: 40}, rangeid='', name='rep0_range_188'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.4, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.4, 1: 50}, rangeid='', name='rep0_range_189'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.6000000000000005, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.6000000000000005, 1: 10}, rangeid='', name='rep0_range_190'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.6000000000000005, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.6000000000000005, 1: 20}, rangeid='', name='rep0_range_191'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.6000000000000005, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.6000000000000005, 1: 30}, rangeid='', name='rep0_range_192'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.6000000000000005, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.6000000000000005, 1: 40}, rangeid='', name='rep0_range_193'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.6000000000000005, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.6000000000000005, 1: 50}, rangeid='', name='rep0_range_194'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.800000000000001, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.800000000000001, 1: 10}, rangeid='', name='rep0_range_195'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.800000000000001, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.800000000000001, 1: 20}, rangeid='', name='rep0_range_196'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.800000000000001, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.800000000000001, 1: 30}, rangeid='', name='rep0_range_197'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.800000000000001, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.800000000000001, 1: 40}, rangeid='', name='rep0_range_198'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 7.800000000000001, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 7.800000000000001, 1: 50}, rangeid='', name='rep0_range_199'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 8.0, 'period': 10, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 8.0, 1: 10}, rangeid='', name='rep0_range_200'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 8.0, 'period': 20, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 8.0, 1: 20}, rangeid='', name='rep0_range_201'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 8.0, 'period': 30, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 8.0, 1: 30}, rangeid='', name='rep0_range_202'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 8.0, 'period': 40, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 8.0, 1: 40}, rangeid='', name='rep0_range_203'),
ParameterScenario(sequence={}, times=(), p={'ground': {'amp': 8.0, 'period': 50, 'linetype': 'sine'}}, r={}, sp={}, prob=0.004878048780487805, inputparams={0: 8.0, 1: 50}, rangeid='', name='rep0_range_204')]
[14]:
ps_turn = ParameterSample(pd_turn)
ps_turn.add_variable_ranges(comb_kwargs={'resolutions':{'ground.radius': 5.0, "ground.start": 5}})
ps_turn.scenarios()
[14]:
[ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 0, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 0}, rangeid='', name='rep0_range_0'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 1, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 1}, rangeid='', name='rep0_range_1'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 2, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 2}, rangeid='', name='rep0_range_2'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 3, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 3}, rangeid='', name='rep0_range_3'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 4, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 4}, rangeid='', name='rep0_range_4'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 5.0, 'x_start': 5, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 5.0, 1: 5}, rangeid='', name='rep0_range_5'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 0, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 0}, rangeid='', name='rep0_range_6'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 1, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 1}, rangeid='', name='rep0_range_7'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 2, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 2}, rangeid='', name='rep0_range_8'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 3, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 3}, rangeid='', name='rep0_range_9'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 4, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 4}, rangeid='', name='rep0_range_10'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 10.0, 'x_start': 5, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 10.0, 1: 5}, rangeid='', name='rep0_range_11'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 0, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 0}, rangeid='', name='rep0_range_12'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 1, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 1}, rangeid='', name='rep0_range_13'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 2, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 2}, rangeid='', name='rep0_range_14'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 3, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 3}, rangeid='', name='rep0_range_15'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 4, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 4}, rangeid='', name='rep0_range_16'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 15.0, 'x_start': 5, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 15.0, 1: 5}, rangeid='', name='rep0_range_17'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 0, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 0}, rangeid='', name='rep0_range_18'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 1, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 1}, rangeid='', name='rep0_range_19'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 2, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 2}, rangeid='', name='rep0_range_20'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 3, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 3}, rangeid='', name='rep0_range_21'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 4, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 4}, rangeid='', name='rep0_range_22'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 20.0, 'x_start': 5, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 20.0, 1: 5}, rangeid='', name='rep0_range_23'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 0, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 0}, rangeid='', name='rep0_range_24'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 1, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 1}, rangeid='', name='rep0_range_25'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 2, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 2}, rangeid='', name='rep0_range_26'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 3, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 3}, rangeid='', name='rep0_range_27'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 4, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 4}, rangeid='', name='rep0_range_28'),
ParameterScenario(sequence={}, times=(), p={'ground': {'radius': 25.0, 'x_start': 5, 'linetype': 'turn'}}, r={}, sp={}, prob=0.03333333333333333, inputparams={0: 25.0, 1: 5}, rangeid='', name='rep0_range_29')]
These samples can then be run using prop.parameter_sample
[15]:
res_turn, hist_turn = prop.parameter_sample(mdl, ps_turn, pool = mp.Pool(5))
SCENARIOS COMPLETE: 100%|██████████| 30/30 [00:03<00:00, 9.75it/s]
[16]:
res_sine, hist_sine = prop.parameter_sample(mdl, ps_sine, pool=mp.Pool(5))
SCENARIOS COMPLETE: 100%|██████████| 205/205 [00:11<00:00, 17.27it/s]
We can then use these results to visualize the operational envelope for the system–which sets of parameters lead to the system getting lost/going off track, and which sets of parameters the system is capable of accomodating.
[17]:
from fmdtools.analyze.tabulate import NominalEnvelope
na = NominalEnvelope(ps_sine, res_sine, 'at_finish', 'p.ground.amp', 'p.ground.period', func=lambda x: x == True)
na.as_plot()
[17]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='p.ground.amp', ylabel='p.ground.period'>)
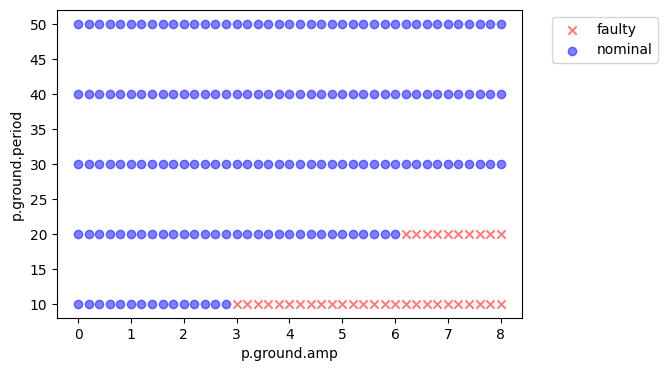
As shown, if the amplitude of the sine curve is too high at low wavelength, it causes the rover to go off track and not be able to complete the mission.
[18]:
na = NominalEnvelope(ps_turn, res_turn, 'at_finish', 'p.ground.x_start', 'p.ground.radius', func=lambda x: x == True)
na.as_plot()
[18]:
(<Figure size 600x400 with 1 Axes>,
<Axes: xlabel='p.ground.x_start', ylabel='p.ground.radius'>)
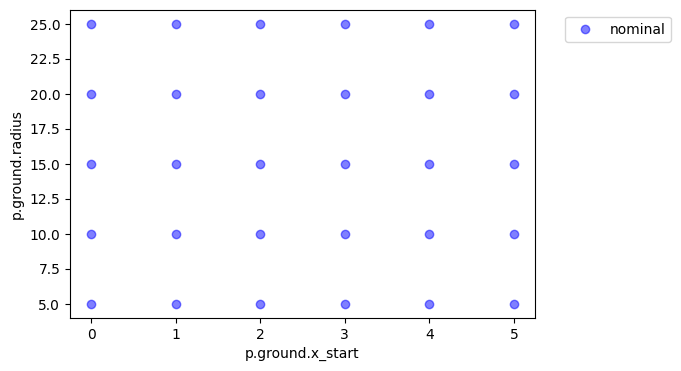
Similarly, if the radius of the curve is too small, it can additionally lead to the rover going off course by overshooting the curve.
[19]:
fig = hist_turn.plot_trajectories('flows.pos.s.x','flows.pos.s.y')
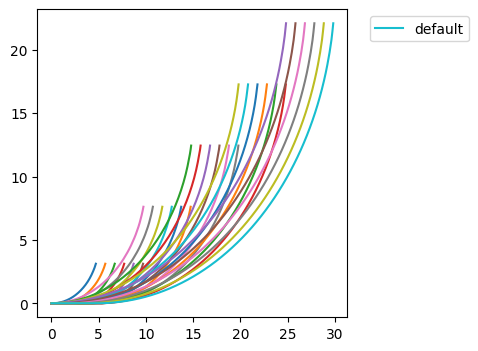
[20]:
fig = hist_sine.plot_trajectories('flows.pos.s.x','flows.pos.s.y')
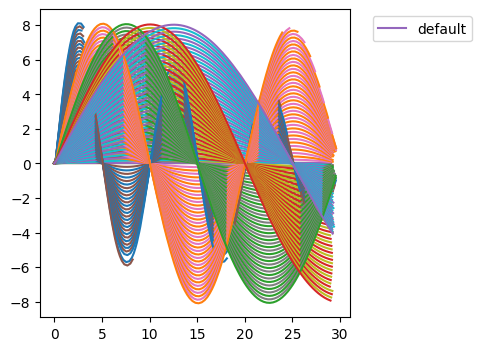
[ ]:
[ ]: