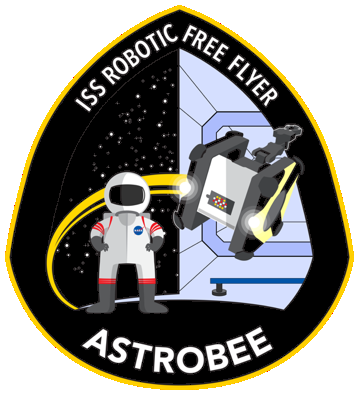 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef SPARSE_MAPPING_SPARSE_MAP_H_
20 #define SPARSE_MAPPING_SPARSE_MAP_H_
30 #include <boost/optional.hpp>
31 #include <Eigen/Geometry>
32 #include <opencv2/core/core.hpp>
33 #include <opencv2/imgproc.hpp>
54 std::vector<std::map<int, int> >
const& pid_to_cid_fid,
55 std::vector<std::map<int, int> > * cid_fid_to_pid);
64 EIGEN_MAKE_ALIGNED_OPERATOR_NEW
72 SparseMap(
const std::vector<std::string> & filenames,
80 SparseMap(
const std::string & protobuf_file,
81 bool localization =
false);
86 SparseMap(
const std::vector<Eigen::Affine3d>& cid_to_cam_t,
87 const std::vector<std::string> & filenames,
92 SparseMap(
bool bundler_format, std::string
const& filename, std::vector<std::string>
const& files);
102 void SetDetectorParams(
int min_features,
int max_features,
int retries,
double min_thresh,
double default_thresh,
103 double max_thresh,
double too_many_ratio,
double too_few_ratio);
113 void Save(
const std::string & protobuf_file)
const;
119 std::vector<Eigen::Vector3d>* inlier_landmarks = NULL,
120 std::vector<Eigen::Vector2d>* inlier_observations = NULL,
121 std::vector<int> * cid_list = NULL);
122 bool Localize(
const cv::Mat & image,
124 std::vector<Eigen::Vector2d>* inlier_observations = NULL,
125 std::vector<int> * cid_list = NULL);
127 std::vector<Eigen::Vector3d>* inlier_landmarks, std::vector<Eigen::Vector2d>* inlier_observations,
128 std::vector<int>* cid_list = NULL,
const cv::Mat& image = cv::Mat());
187 0, [](
size_t v, std::map<int, int>
const& map)
188 { return v + map.size(); }); }
203 void Load(
const std::string & protobuf_file,
bool localization =
false);
216 cv::Mat* descriptors,
217 Eigen::Matrix2Xd* keypoints);
220 cv::Mat* descriptors,
221 Eigen::Matrix2Xd* keypoints);
281 void reorderMap(std::map<int, int>
const& old_cid_to_new_cid);
285 #endif // SPARSE_MAPPING_SPARSE_MAP_H_
const std::map< int, int > & GetFrameFidToPidMap(int frame) const
Definition: sparse_map.h:159
std::vector< std::map< int, int > > cid_fid_to_pid_
Definition: sparse_map.h:238
std::vector< Eigen::Matrix2Xd > cid_to_keypoint_map_
Definition: sparse_map.h:232
const Eigen::Affine3d & GetFrameGlobalTransform(int frame) const
Definition: sparse_map.h:142
void InitializeCidFidToPid(int num_cid, std::vector< std::map< int, int > > const &pid_to_cid_fid, std::vector< std::map< int, int > > *cid_fid_to_pid)
Definition: sparse_map.cc:645
std::map< int, int > db_to_cid_map_
Definition: sparse_map.h:248
void SetDetectorParams(int min_features, int max_features, int retries, double min_thresh, double default_thresh, double max_thresh, double too_many_ratio, double too_few_ratio)
Definition: sparse_map.cc:509
void LoadKeypoints(const std::string &protobuf_file)
Definition: sparse_map.cc:474
void PruneMap(void)
Definition: sparse_map.cc:956
std::vector< Eigen::Affine3d > cid_to_cam_t_global_
Definition: sparse_map.h:235
const Eigen::Matrix2Xd & GetFrameKeypoints(int frame) const
Definition: sparse_map.h:151
void DetectFeatures()
Definition: sparse_map.cc:272
Definition: localization_parameters.h:22
Definition: matching.h:58
std::vector< std::string > cid_to_filename_
Definition: sparse_map.h:230
const LocalizationParameters & loc_params() const
Definition: sparse_map.h:99
Definition: camera_params.h:58
void Save(const std::string &protobuf_file) const
Definition: sparse_map.cc:518
LocalizationParameters & loc_params()
Definition: sparse_map.h:100
void SetDefaultLocParams()
Definition: sparse_map.cc:241
interest_point::FeatureDetector detector_
Definition: sparse_map.h:241
void SetFrameGlobalTransform(int frame, const Eigen::Affine3d &transform)
Definition: sparse_map.h:145
Eigen::Affine3d world_transform_
Definition: sparse_map.h:259
std::vector< cv::Mat > cid_to_descriptor_map_
Definition: sparse_map.h:236
cv::Mat GetDescriptor(int frame, int fid) const
Definition: sparse_map.h:155
Definition: camera_model.h:31
boost::optional< int > best_previous_cid_
Definition: sparse_map.h:265
const std::map< int, int > & GetLandmarkCidToFidMap(int landmark) const
Definition: sparse_map.h:174
sparse_mapping::VocabDB vocab_db_
Definition: sparse_map.h:243
camera::CameraParameters camera_params_
Definition: sparse_map.h:242
std::string protobuf_file_
Definition: sparse_map.h:245
LocalizationParameters loc_params_
Definition: sparse_map.h:244
bool Localize(std::string const &img_file, camera::CameraModel *pose, std::vector< Eigen::Vector3d > *inlier_landmarks=NULL, std::vector< Eigen::Vector2d > *inlier_observations=NULL, std::vector< int > *cid_list=NULL)
Definition: sparse_map.cc:940
void SetLocParams(const LocalizationParameters &loc_params)
Definition: sparse_map.cc:263
std::vector< std::map< int, int > > user_pid_to_cid_fid_
Definition: sparse_map.h:261
int histogram_equalization
Definition: localization_parameters.h:29
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
std::string GetDetectorName()
Definition: sparse_map.h:225
void Load(const std::string &protobuf_file, bool localization=false)
Definition: sparse_map.cc:305
interest_point::FeatureDetector & detector()
Definition: sparse_map.h:226
Eigen::Affine3d GetWorldTransform() const
Definition: sparse_map.h:192
camera::CameraParameters GetCameraParameters(void) const
Definition: sparse_map.h:180
std::vector< std::map< int, int > > pid_to_cid_fid_
Definition: sparse_map.h:233
std::vector< Eigen::Vector3d > user_pid_to_xyz_
Definition: sparse_map.h:262
void SetCameraParameters(camera::CameraParameters camera_params)
Definition: sparse_map.h:181
Definition: vocab_tree.h:74
size_t GetNumLandmarks(void) const
Definition: sparse_map.h:165
Eigen::Vector3d GetLandmarkPosition(int landmark) const
Definition: sparse_map.h:169
Definition: localization_parameters.h:23
void InitializeCidFidToPid()
Definition: sparse_map.cc:658
const std::string & GetFrameFilename(int frame) const
Definition: sparse_map.h:138
std::map< int, std::set< int > > cid_to_cid_
Definition: sparse_map.h:254
std::vector< Eigen::Vector3d > pid_to_xyz_
Definition: sparse_map.h:234
size_t GetNumObservations(void) const
Definition: sparse_map.h:185
size_t GetNumFrames(void) const
Definition: sparse_map.h:134
Eigen::Affine3d Affine3d(const Eigen::Matrix< double, 7, 1 > &affine_vector)
Definition: utilities.cc:43
void TransformCamerasAndPoints(Eigen::Affine3d const &A, std::vector< Eigen::Affine3d > *cid_to_cam_t, std::vector< Eigen::Vector3d > *xyz)
Definition: sparse_mapping.cc:992
void DetectFeaturesFromFile(std::string const &filename, bool multithreaded, cv::Mat *descriptors, Eigen::Matrix2Xd *keypoints)
Definition: sparse_map.cc:664
std::mutex mutex_detector_
Definition: sparse_map.h:266
cv::Ptr< cv::CLAHE > clahe_
Definition: sparse_map.h:264
std::vector< std::map< int, int > > cid_to_matching_cid_counts_
Definition: sparse_map.h:239
int GetHistogramEqualization()
Definition: sparse_map.h:227
Definition: camera_params.h:31
std::string GetDetectorName() const
Definition: matching.h:81
Definition: sparse_map.h:63
std::vector< Eigen::Matrix2Xd > user_cid_to_keypoint_map_
Definition: sparse_map.h:260
void ApplyTransform(Eigen::Affine3d const &T)
Definition: sparse_map.h:197