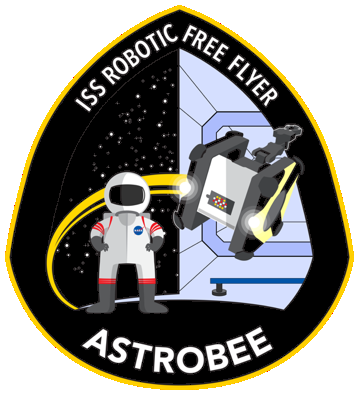 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_BASIC_POLYNOMIAL_BASIS_H_
20 #define TRAJ_OPT_BASIC_POLYNOMIAL_BASIS_H_
25 #include <boost/make_shared.hpp>
26 #include <boost/math/special_functions/binomial.hpp>
27 #include <boost/math/tools/polynomial.hpp>
39 explicit Basis(uint n_p_);
61 typedef boost::math::tools::polynomial<decimal_t>
Poly;
87 boost::shared_ptr<Basis>
getBasis(
int i);
121 #endif // TRAJ_OPT_BASIC_POLYNOMIAL_BASIS_H_
std::vector< boost::shared_ptr< Basis > > integrals
Definition: polynomial_basis.h:90
boost::shared_ptr< Basis > getBasis(int i)
Definition: polynomial_basis.cpp:142
PolyType
Definition: traj_data.h:27
const PolyType & type() const
Definition: polynomial_basis.h:49
bool orthogonal()
Definition: polynomial_basis.h:50
Basis(uint n_p_)
Definition: polynomial_basis.cpp:119
Definition: polynomial_basis.h:105
uint k_r
Definition: polynomial_basis.h:117
uint n_p
Definition: polynomial_basis.h:53
decimal_t getVal(decimal_t x, decimal_t dt, uint coeff, int derr) const
Definition: polynomial_basis.cpp:96
virtual Poly getPoly(uint i) const
Definition: polynomial_basis.cpp:128
Definition: polynomial_basis.h:71
uint n_p
Definition: polynomial_basis.h:93
BasisBundle()
Definition: polynomial_basis.h:79
virtual decimal_t evaluate(decimal_t x, uint coeff) const
Definition: polynomial_basis.cpp:78
double decimal_t
Definition: types.h:35
virtual void differentiate()
Definition: polynomial_basis.cpp:65
bool orthogonal_
Definition: polynomial_basis.h:55
StandardBasis(uint n_p_)
Definition: polynomial_basis.cpp:129
virtual decimal_t innerproduct(uint i, uint j) const =0
Definition: msg_traj.h:27
std::vector< Poly > polys
Definition: polynomial_basis.h:116
static Poly integrate(const Poly &p)
Definition: polynomial_basis.cpp:33
uint k_r
Definition: polynomial_basis.h:93
virtual void integrate()=0
virtual decimal_t innerproduct(uint i, uint j) const
Definition: polynomial_basis.cpp:122
PolyType type_
Definition: polynomial_basis.h:54
virtual void differentiate()=0
friend std::ostream & operator<<(std::ostream &os, const StandardBasis &lb)
Definition: polynomial_basis.cpp:88
Definition: polynomial_basis.h:63
static Poly differentiate(const Poly &p)
Definition: polynomial_basis.cpp:45
virtual uint dim()
Definition: polynomial_basis.cpp:58
virtual decimal_t evaluate(decimal_t x, uint i) const =0
std::vector< boost::shared_ptr< Basis > > derrivatives
Definition: polynomial_basis.h:89
boost::math::tools::polynomial< decimal_t > Poly
Definition: polynomial_basis.h:61
virtual void integrate()
Definition: polynomial_basis.cpp:71
Definition: polynomial_basis.h:37