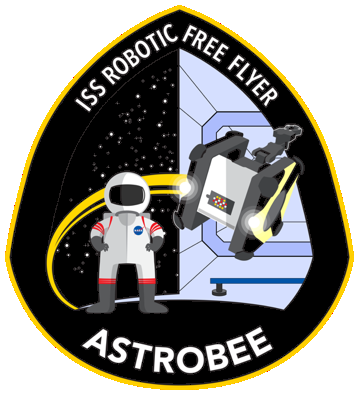 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef ROS_GRAPH_LOCALIZER_ROS_GRAPH_LOCALIZER_WRAPPER_H_
19 #define ROS_GRAPH_LOCALIZER_ROS_GRAPH_LOCALIZER_WRAPPER_H_
21 #include <ff_msgs/EkfState.h>
22 #include <ff_msgs/Feature2dArray.h>
23 #include <ff_msgs/FlightMode.h>
24 #include <ff_msgs/GraphVIOState.h>
25 #include <ff_msgs/GraphLocState.h>
26 #include <ff_msgs/VisualLandmarks.h>
36 #include <sensor_msgs/Imu.h>
50 void LoadConfigs(
const std::string& graph_config_path_prefix);
83 boost::optional<gtsam::Pose3>
LatestPose()
const;
86 boost::optional<gtsam::Pose3>
WorldTDock()
const;
97 const std::unique_ptr<graph_localizer::GraphLocalizer>&
graph_localizer()
const;
103 std::unique_ptr<graph_localizer::GraphLocalizer> graph_localizer_;
104 std::unique_ptr<imu_integration::ImuIntegrator> imu_integrator_;
109 boost::optional<localization_common::CombinedNavState> latest_vio_state_;
110 boost::optional<localization_common::Time> latest_msg_time_;
111 boost::optional<localization_common::Time> last_vio_msg_time_;
112 boost::optional<gtsam::Pose3> world_T_dock_;
113 int latest_num_detected_ml_features_;
114 int latest_num_detected_ar_features_;
118 #endif // ROS_GRAPH_LOCALIZER_ROS_GRAPH_LOCALIZER_WRAPPER_H_
void FlightModeCallback(const ff_msgs::FlightMode &flight_mode)
Definition: ros_graph_localizer_wrapper.cc:225
boost::optional< localization_common::Time > LatestTimestamp() const
Definition: ros_graph_localizer_wrapper.cc:251
void ARVisualLandmarksCallback(const ff_msgs::VisualLandmarks &visual_landmarks_msg)
Definition: ros_graph_localizer_wrapper.cc:100
Definition: ros_graph_localizer_wrapper_params.h:26
bool GraphVIOStateCallback(const ff_msgs::GraphVIOState &graph_vio_state_msg)
Definition: ros_graph_localizer_wrapper.cc:180
void ImuCallback(const sensor_msgs::Imu &imu_msg)
Definition: ros_graph_localizer_wrapper.cc:221
Definition: parameter_reader.h:27
void LoadConfigs(const std::string &graph_config_path_prefix)
Definition: ros_graph_localizer_wrapper.cc:48
void ResetLocalizer()
Definition: ros_graph_localizer_wrapper.cc:235
bool Initialized() const
Definition: ros_graph_localizer_wrapper.cc:233
void SparseMapVisualLandmarksCallback(const ff_msgs::VisualLandmarks &visual_landmarks_msg)
Definition: ros_graph_localizer_wrapper.cc:58
boost::optional< ff_msgs::GraphLocState > GraphLocStateMsg()
Definition: ros_graph_localizer_wrapper.cc:279
boost::optional< gtsam::Pose3 > WorldTDock() const
Definition: ros_graph_localizer_wrapper.cc:269
Definition: timestamped_interpolater.h:40
Definition: graph_localizer_params.h:31
boost::optional< gtsam::Pose3 > LatestPose() const
Definition: ros_graph_localizer_wrapper.cc:259
void Update()
Definition: ros_graph_localizer_wrapper.cc:229
Definition: ros_graph_localizer_wrapper.h:45
std::unique_ptr< graph_localizer::GraphLocalizer > & graph_localizer()
Definition: ros_graph_localizer_wrapper.cc:271
RosGraphLocalizerWrapper(const std::string &graph_config_path_prefix="")
Definition: ros_graph_localizer_wrapper.cc:40
void ResetWorldTDock()
Definition: ros_graph_localizer_wrapper.cc:267