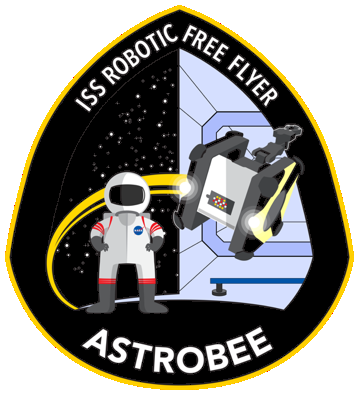 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
24 #include <libusb-1.0/libusb.h>
32 #define MAX_PACKET_LEN 64
33 #define PREAMBLE_LENGTH 17
35 #define MAX_NUM_LIGHTHOUSES 2
36 #define MAX_NUM_TRACKERS 128
37 #define MAX_NUM_SENSORS 32
38 #define MAX_SERIAL_LENGTH 32
39 #define USB_INT_BUFF_LENGTH 64
41 #define USB_VEND_HTC 0x28de
42 #define USB_PROD_TRACKER_V1 0x2022
43 #define USB_PROD_TRACKER_V2 0x2300
44 #define USB_PROD_CONTROLLER_V1 0x2012
45 #define USB_PROD_WATCHMAN 0x2101
47 #define USB_ENDPOINT_WATCHMAN 0x81
48 #define USB_ENDPOINT_V1_GENERAL 0x81
49 #define USB_ENDPOINT_V1_LIGHT 0x82
50 #define USB_ENDPOINT_V1_BUTTONS 0x83
51 #define USB_ENDPOINT_V2_GENERAL 0x81
52 #define USB_ENDPOINT_V2_LIGHT 0x83
53 #define USB_ENDPOINT_V2_BUTTONS 0x84
54 #define MAX_ENDPOINTS 3
56 #define DEFAULT_ACC_SCALE (float)(9.80665/4096.0)
57 #define DEFAULT_GYR_SCALE (float)((1./32.768)*(3.14159/180.));
61 #define DEBUG_PRINTF(f_, ...)
86 struct libusb_transfer *
tx;
151 struct libusb_device_handle *
udev;
224 uint32_t synctime, uint16_t num_sensors,
light_t const * measurement);
232 struct libusb_context*
usb;
278 #endif // VIVE_VIVE_H_
int32_t pulse_synctime_offset
Definition: vive.h:199
int32_t timecenter_ticks
Definition: vive.h:194
int activeAcode
Definition: vive.h:130
float head_transform[7]
Definition: vive.h:103
uint32_t timestamp
Definition: vive.h:92
struct LightcapSweepData lightcap_sweep_data_t
int32_t timebase_hz
Definition: vive.h:193
void vive_kill(driver_t *drv)
struct Endpoint endpoint_t
void(* imu_cb_t)(tracker_t const *tracker, imu_t const *measurement)
Definition: vive.h:225
uint8_t hw_version
Definition: vive.h:186
float positions[MAX_NUM_SENSORS][3]
Definition: vive.h:95
#define MAX_PACKET_LEN
Definition: vive.h:32
float acc_bias[3]
Definition: vive.h:97
uint16_t pos
Definition: vive.h:113
void(* lighthouse_cb_t)(lighthouse_t const *lighthouse)
Definition: vive.h:228
#define MAX_NUM_TRACKERS
Definition: vive.h:36
float curve
Definition: vive.h:174
@ EVENT_TRIGGER
Definition: vive.h:75
struct libusb_device_handle * udev
Definition: vive.h:151
@ DATA_LIGHT_9
Definition: vive.h:70
struct PerSweepData per_sweep_data_t
uint32_t sweep_time[MAX_NUM_SENSORS]
Definition: vive.h:122
#define MAX_ENDPOINTS
Definition: vive.h:54
tracker_t * vive_tracker(driver_t *tracker, const char *id)
int16_t gyr[3]
Definition: vive.h:212
uint16_t syn
Definition: vive.h:114
float imu_transform[7]
Definition: vive.h:102
@ MOTOR_AXIS0
Definition: vive.h:166
lightcap_data_t lcd
Definition: vive.h:155
state_t
Definition: vive.h:107
char serial[MAX_SERIAL_LENGTH]
Definition: vive.h:182
uint8_t sensor_id
Definition: vive.h:205
calibration_t cal
Definition: vive.h:154
#define MAX_SERIAL_LENGTH
Definition: vive.h:38
struct Tracker tracker_t
Definition: vive.h:65
state_t state
Definition: vive.h:109
uint8_t charge
Definition: vive.h:157
tracker_cb_t tracker_cb
Definition: vive.h:238
uint16_t fw_version
Definition: vive.h:181
uint8_t sys_faults
Definition: vive.h:188
void vive_install_lighthouse_fn(driver_t *drv, lighthouse_cb_t fbp)
void(* button_cb_t)(tracker_t const *tracker, button_t const *measurement)
Definition: vive.h:226
#define MAX_NUM_LIGHTHOUSES
Definition: vive.h:35
@ LENGTH
Definition: vive.h:107
ootx_t ootx[MAX_NUM_LIGHTHOUSES]
Definition: vive.h:156
uint16_t length
Definition: vive.h:111
uint16_t num_trackers
Definition: vive.h:233
int activeSweepStartTime
Definition: vive.h:128
uint32_t lasttime
Definition: vive.h:117
uint8_t ison
Definition: vive.h:159
@ MOTOR_AXIS1
Definition: vive.h:166
lighthouse_t lighthouses[MAX_NUM_LIGHTHOUSES]
Definition: vive.h:240
lighthouse_cb_t lighthouse_cb
Definition: vive.h:239
uint16_t sweep_len[MAX_NUM_SENSORS]
Definition: vive.h:123
int32_t pulsedist_max_ticks
Definition: vive.h:195
double acode_offset
Definition: vive.h:138
int current_lh
Definition: vive.h:134
void vive_install_light_fn(driver_t *drv, light_cb_t fbp)
general_t * vive_general(driver_t *drv)
lighthouse_t * vive_lighthouse(driver_t *drv, const char *id)
@ EVENT_GRIP
Definition: vive.h:76
@ PAYLOAD
Definition: vive.h:107
int lh_max_pulse_length[MAX_NUM_LIGHTHOUSES]
Definition: vive.h:132
float acc_scale[3]
Definition: vive.h:98
Event
Definition: vive.h:74
float phase
Definition: vive.h:170
@ EVENT_PAD_CLICK
Definition: vive.h:78
int lh_start_time[MAX_NUM_LIGHTHOUSES]
Definition: vive.h:131
uint8_t pad
Definition: vive.h:112
@ DATA_WATCHMAN
Definition: vive.h:70
general_t general
Definition: vive.h:241
struct GlobalData global_data_t
int recent_sync_time
Definition: vive.h:127
uint8_t preamble
Definition: vive.h:116
float normals[MAX_NUM_SENSORS][3]
Definition: vive.h:96
lightcap_sweep_data_t sweep
Definition: vive.h:142
@ PREAMBLE
Definition: vive.h:107
motor_t motors[MAX_NUM_MOTORS]
Definition: vive.h:183
int activeLighthouse
Definition: vive.h:129
#define MAX_NUM_SENSORS
Definition: vive.h:37
uint8_t data[MAX_PACKET_LEN]
Definition: vive.h:110
struct libusb_transfer * tx
Definition: vive.h:86
uint32_t timestamp
Definition: vive.h:204
global_data_t global
Definition: vive.h:144
uint8_t vive_poll(driver_t *drv)
uint8_t pushed
Definition: vive.h:242
uint8_t channels[MAX_NUM_SENSORS]
Definition: vive.h:94
int32_t pulse_max_for_sweep
Definition: vive.h:198
uint32_t crc
Definition: vive.h:115
float gyr_scale[3]
Definition: vive.h:100
#define USB_INT_BUFF_LENGTH
Definition: vive.h:39
@ EVENT_PAD_TOUCH
Definition: vive.h:79
struct Calibration calibration_t
float accel[3]
Definition: vive.h:184
void(* tracker_cb_t)(tracker_t const *tracker)
Definition: vive.h:227
@ CHECKSUM
Definition: vive.h:107
float tilt
Definition: vive.h:171
Axis
Definition: vive.h:166
struct Driver driver_t
Definition: vive.h:64
float gibmag
Definition: vive.h:173
struct LightcapData lightcap_data_t
uint16_t type
Definition: vive.h:149
struct Lighthouse lighthouse_t
Definition: vive.h:66
@ EVENT_MENU
Definition: vive.h:77
button_cb_t button_cb
Definition: vive.h:237
int32_t pulselength_min_sync
Definition: vive.h:196
uint8_t axis[3]
Definition: vive.h:160
uint8_t sys_unlock_count
Definition: vive.h:185
int32_t pulse_in_clear_time
Definition: vive.h:197
light_cb_t light_cb
Definition: vive.h:235
endpoint_t endpoints[MAX_ENDPOINTS]
Definition: vive.h:153
float gyr_bias[3]
Definition: vive.h:99
driver_t * driver
Definition: vive.h:150
int32_t pulse_synctime_slack
Definition: vive.h:200
int16_t acc[3]
Definition: vive.h:211
per_sweep_data_t per_sweep
Definition: vive.h:143
float gibphase
Definition: vive.h:172
uint32_t timecode
Definition: vive.h:162
uint32_t timestamp
Definition: vive.h:179
uint8_t id
Definition: vive.h:180
@ DATA_BUTTONS
Definition: vive.h:70
uint8_t buffer[USB_INT_BUFF_LENGTH]
Definition: vive.h:87
tracker_t * trackers[MAX_NUM_TRACKERS]
Definition: vive.h:234
void vive_install_imu_fn(driver_t *drv, imu_cb_t fbp)
data_t type
Definition: vive.h:85
void(* light_cb_t)(tracker_t const *tracker, uint8_t lh, uint8_t axis, uint32_t synctime, uint16_t num_sensors, light_t const *measurement)
Definition: vive.h:223
uint8_t buttonmask
Definition: vive.h:161
uint16_t length
Definition: vive.h:206
imu_cb_t imu_cb
Definition: vive.h:236
@ MAX_NUM_MOTORS
Definition: vive.h:166
uint8_t num_channels
Definition: vive.h:93
@ DATA_IMU
Definition: vive.h:70
tracker_t * tracker
Definition: vive.h:84
lighthouse_t * lighthouse
Definition: vive.h:118
void vive_install_tracker_fn(driver_t *drv, tracker_cb_t fbp)
uint8_t ischarging
Definition: vive.h:158
Data
Definition: vive.h:69
@ DATA_LIGHT_7
Definition: vive.h:70
uint32_t timestamp
Definition: vive.h:210
void vive_install_button_fn(driver_t *drv, button_cb_t fbp)
uint8_t mode_current
Definition: vive.h:187
char serial[MAX_SERIAL_LENGTH]
Definition: vive.h:152
int8_t lh_acode[MAX_NUM_LIGHTHOUSES]
Definition: vive.h:133
struct libusb_context * usb
Definition: vive.h:232