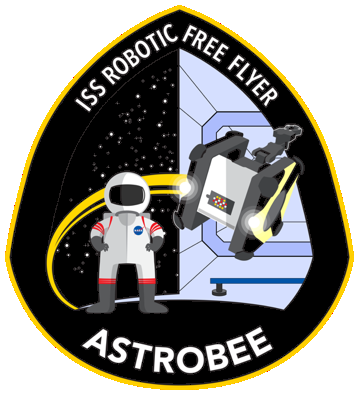 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef COMMS_BRIDGE_GENERIC_ROS_SUB_RAPID_PUB_H_
20 #define COMMS_BRIDGE_GENERIC_ROS_SUB_RAPID_PUB_H_
28 #include <ff_msgs/GenericCommsAdvertisementInfo.h>
29 #include <ff_msgs/GenericCommsContent.h>
30 #include <ff_msgs/GenericCommsReset.h>
37 #include "dds_msgs/GenericCommsRequestSupport.h"
51 TopicEntry(std::string robot_in, std::string topic_in,
double rate_in) :
62 std::string
const& md5_sum,
63 const size_t data_size,
64 uint8_t
const* data) {
90 std::vector<std::shared_ptr<TopicEntry>>>
const& link_entries);
94 void InitializeDDS(std::vector<std::string>
const& connections);
107 void ConvertRequest(rapid::ext::astrobee::GenericCommsRequest
const* data,
108 std::string
const& connecting_robot);
113 bool dds_initialized_, pub_rate_msgs_;
115 ros::Timer rate_msg_pub_timer_;
117 std::map<std::string, std::vector<std::shared_ptr<TopicEntry>>> topic_mapping_;
118 std::map<std::string, GenericRapidPubPtr> robot_connections_;
119 std::vector<std::shared_ptr<TopicEntry>> rate_topic_entries_;
124 #endif // COMMS_BRIDGE_GENERIC_ROS_SUB_RAPID_PUB_H_
Definition: generic_ros_sub_rapid_pub.h:84
int seq_num_
Definition: generic_ros_sub_rapid_pub.h:78
Definition: bridge_subscriber.h:71
GenericROSSubRapidPub(ros::NodeHandle const *nh)
Definition: generic_ros_sub_rapid_pub.cpp:28
virtual void advertiseTopic(const RelayTopicInfo &info)
Definition: generic_ros_sub_rapid_pub.cpp:106
void SetDataToSend(const int seq_num, std::string const &md5_sum, const size_t data_size, uint8_t const *data)
Definition: generic_ros_sub_rapid_pub.h:61
std::string out_topic_
Definition: generic_ros_sub_rapid_pub.h:72
uint8_t const * data_
Definition: generic_ros_sub_rapid_pub.h:81
std::string type_md5_sum_
Definition: generic_ros_sub_rapid_pub.h:79
float FloatMod(float x, float y)
Definition: generic_ros_sub_rapid_pub.cpp:81
void InitializeDDS(std::vector< std::string > const &connections)
Definition: generic_ros_sub_rapid_pub.cpp:87
Definition: bridge_subscriber.h:84
virtual void subscribeTopic(std::string const &in_topic, const RelayTopicInfo &info)
Definition: generic_ros_sub_rapid_pub.cpp:99
Definition: generic_rapid_msg_ros_pub.h:36
~GenericROSSubRapidPub()
Definition: generic_ros_sub_rapid_pub.cpp:39
std::string connecting_robot_
Definition: generic_ros_sub_rapid_pub.h:71
void CheckPubMsg(const ros::TimerEvent &event)
Definition: generic_ros_sub_rapid_pub.cpp:249
TopicEntry(std::string robot_in, std::string topic_in, double rate_in)
Definition: generic_ros_sub_rapid_pub.h:51
size_t data_size_
Definition: generic_ros_sub_rapid_pub.h:80
Definition: generic_ros_sub_rapid_pub.h:41
virtual void relayMessage(const RelayTopicInfo &topic_info, ContentInfo const &content_info)
Definition: generic_ros_sub_rapid_pub.cpp:142
void AddTopics(std::map< std::string, std::vector< std::shared_ptr< TopicEntry >>> const &link_entries)
Definition: generic_ros_sub_rapid_pub.cpp:41
double rate_seconds_
Definition: generic_ros_sub_rapid_pub.h:73
void ConvertRequest(rapid::ext::astrobee::GenericCommsRequest const *data, std::string const &connecting_robot)
Definition: generic_ros_sub_rapid_pub.cpp:186
Definition: bridge_subscriber.h:40
double last_time_pub_
Definition: generic_ros_sub_rapid_pub.h:77
TopicEntry()
Definition: generic_ros_sub_rapid_pub.h:43