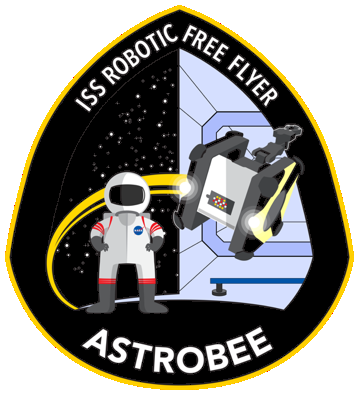 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef EPSON_IMU_G362P_H_
20 #define EPSON_IMU_G362P_H_
22 #define EPSON_POWER_ON_DELAY 800000 // usec
24 #define STALL_NORMAL_MODE 20 // usec
25 #define STALL_BURST_MODE_BEFORE_DATA 45 // usec
26 #define STALL_BURST_MODE_BETWEEN_DATA 5 // usec
29 #define REG_BURST_H 0x00
30 #define REG_BURST_L 0x01
31 #define REG_MODE_CTRL_H 0x02
32 #define REG_MODE_CTRL_L 0x03
33 #define REG_DIAG_STAT_H 0x04
34 #define REG_DIAG_STAT_L 0x05
35 #define REG_FLAG_H 0x06
36 #define REG_FLAG_L 0x07
37 #define REG_GPIO_H 0x08
38 #define REG_GPIO_L 0x09
39 #define REG_COUNT 0x0A
40 #define REG_TEMP_HIGH 0x0E
41 #define REG_TEMP_LOW 0x10
42 #define REG_XGYRO_HIGH 0x12
43 #define REG_XGYRO_LOW 0x14
44 #define REG_YGYRO_HIGH 0x16
45 #define REG_YGYRO_LOW 0x18
46 #define REG_ZGYRO_HIGH 0x1A
47 #define REG_ZGYRO_LOW 0x1C
48 #define REG_XACCL_HIGH 0x1E
49 #define REG_XACCL_LOW 0x20
50 #define REG_YACCL_HIGH 0x22
51 #define REG_YACCL_LOW 0x24
52 #define REG_ZACCL_HIGH 0x26
53 #define REG_ZACCL_LOW 0x28
56 #define REG_SIG_CTRL_H 0x00
57 #define REG_SIG_CTRL_L 0x01
58 #define REG_MSC_CTRL_H 0x02
59 #define REG_MSC_CTRL_L 0x03
60 #define REG_SMPL_CTRL_H 0x04
61 #define REG_SMPL_CTRL_L 0x05
62 #define REG_FILTER_CTRL_H 0x06
63 #define REG_FILTER_CTRL_L 0x07
64 #define REG_UART_CTRL_H 0x08
65 #define REG_UART_CTRL_L 0x09
66 #define REG_GLOB_CMD_H 0x0A
67 #define REG_GLOB_CMD_L 0x0B
68 #define REG_BURST_CTRL1_H 0x0C
69 #define REG_BURST_CTRL1_L 0x0D
70 #define REG_BURST_CTRL2_H 0x0E
71 #define REG_BURST_CTRL2_L 0x0F
72 #define REG_WIN_CTRL_H 0x7E
73 #define REG_WIN_CTRL_L 0x7F
76 #define SF_TEMP (0.0042725)
77 #define SF_GYRO (0.005)
78 #define SF_ACCL (0.125)
80 #define ARRAY_SIZE(a) (sizeof(a) / sizeof((a)[0]))
82 #define CHECK_REG_VALUE 1
214 uint32_t spi_speed_hz_;
224 struct timespec timeout_data_ready_;
227 bool flag_enabled_, temp_enabled_, gyro_enabled_;
228 bool accl_enabled_, gpio_enabled_, count_enabled_, chksm_enabled_;
231 OutputBits temp_bits_, gyro_bits_, accl_bits_;
261 bool EnableOutput(
bool flag,
bool temp,
bool gyro,
bool accl,
bool gpio,
bool count,
bool chksm);
276 uint16_t
TransferWord(uint16_t word, uint16_t delay_usecs);
279 uint16_t MakeWord(uint8_t high, uint8_t low);
280 void BurstRead(
Data *data);
285 #endif // EPSON_IMU_G362P_H_
uint8_t mode
Definition: signal_lights.h:74
#define REG_YGYRO_LOW
Definition: G362P.h:45
#define REG_BURST_L
Definition: G362P.h:30
#define REG_MSC_CTRL_H
Definition: G362P.h:58
Window window
Definition: G362P.h:151
#define REG_GPIO_H
Definition: G362P.h:37
double temp_
Definition: G362P.h:141
#define REG_XGYRO_HIGH
Definition: G362P.h:42
@ MOV_AVG_TAP_32
Definition: G362P.h:112
#define REG_XACCL_LOW
Definition: G362P.h:49
Definition: epson_imu_nodelet.h:37
bool readable
Definition: G362P.h:157
uint16_t ReadRegister(Window window, uint8_t address)
Definition: G362P.cc:127
bool PowerOn(void)
Definition: G362P.cc:81
#define REG_BURST_CTRL2_L
Definition: G362P.h:71
struct epson_imu::_Data Data
bool HasHardError(void)
Definition: G362P.cc:64
@ SPS62_5
Definition: G362P.h:135
@ FIR_TAP_128_FC_100
Definition: G362P.h:124
#define REG_WIN_CTRL_L
Definition: G362P.h:73
@ FIR_TAP_64_FC_200
Definition: G362P.h:121
#define REG_MODE_CTRL_L
Definition: G362P.h:32
#define REG_FLAG_L
Definition: G362P.h:36
bool writable
Definition: G362P.h:159
bool SetFilter(Filter filter)
Definition: G362P.cc:205
#define REG_YGYRO_HIGH
Definition: G362P.h:44
@ FIR_TAP_128_FC_200
Definition: G362P.h:125
@ FIR_TAP_32_FC_50
Definition: G362P.h:115
@ FIR_TAP_32_FC_200
Definition: G362P.h:117
@ MOV_AVG_TAP_8
Definition: G362P.h:110
#define REG_BURST_CTRL2_H
Definition: G362P.h:70
#define REG_BURST_CTRL1_L
Definition: G362P.h:69
Mode
Definition: G362P.h:92
Mode GetMode(void)
Definition: G362P.cc:339
void DumpRegisters(void)
Definition: G362P.cc:138
bool SetMode(Mode mode)
Definition: G362P.cc:302
Filter
Definition: G362P.h:107
struct epson_imu::_Register Register
#define REG_TEMP_HIGH
Definition: G362P.h:40
@ FIR_TAP_32_FC_100
Definition: G362P.h:116
void WriteRegister(Window window, uint8_t address, uint8_t value)
Definition: G362P.cc:122
OutputBits
Definition: G362P.h:102
#define REG_DIAG_STAT_H
Definition: G362P.h:33
#define REG_BURST_CTRL1_H
Definition: G362P.h:68
@ SPS2000
Definition: G362P.h:130
#define REG_GLOB_CMD_H
Definition: G362P.h:66
@ B16
Definition: G362P.h:103
uint16_t gpio_
Definition: G362P.h:144
bool IsReady(void)
Definition: G362P.cc:60
@ FIR_TAP_64_FC_400
Definition: G362P.h:122
#define REG_BURST_H
Definition: G362P.h:29
#define REG_FLAG_H
Definition: G362P.h:35
#define REG_ZGYRO_LOW
Definition: G362P.h:47
@ MOV_AVG_TAP_128
Definition: G362P.h:114
#define REG_UART_CTRL_L
Definition: G362P.h:65
~G362P(void)
Definition: G362P.cc:57
G362P(int fd_spi_dev, uint32_t spi_speed_hz, gpio::GPIO *gpio_data_ready, gpio::GPIO *gpio_reset)
Definition: G362P.cc:35
SamplingRate
Definition: G362P.h:129
@ MOV_AVG_TAP_2
Definition: G362P.h:108
#define REG_ZACCL_HIGH
Definition: G362P.h:52
void SetWindow(Window window)
Definition: G362P.cc:118
#define REG_SIG_CTRL_L
Definition: G362P.h:57
@ SPS250
Definition: G362P.h:133
void EnableUART(bool enable)
Definition: G362P.cc:231
uint16_t chksm_
Definition: G362P.h:146
@ SPS1000
Definition: G362P.h:131
#define REG_SIG_CTRL_H
Definition: G362P.h:56
void PrintData(const Data &data)
Definition: G362P.cc:460
bool SetSamplingRate(SamplingRate sampling_rate)
Definition: G362P.cc:158
#define REG_MSC_CTRL_L
Definition: G362P.h:59
@ WIN1
Definition: G362P.h:99
@ SPS31_25
Definition: G362P.h:136
constexpr Register kRegisters[]
Definition: G362P.h:162
void SoftReset(void)
Definition: G362P.cc:75
bool EnableOutput(bool flag, bool temp, bool gyro, bool accl, bool gpio, bool count, bool chksm)
Definition: G362P.cc:260
#define REG_XACCL_HIGH
Definition: G362P.h:48
#define REG_UART_CTRL_H
Definition: G362P.h:64
@ SPS125
Definition: G362P.h:134
@ MOV_AVG_TAP_4
Definition: G362P.h:109
#define REG_SMPL_CTRL_H
Definition: G362P.h:60
@ MOV_AVG_TAP_64
Definition: G362P.h:113
@ MOV_AVG_TAP_16
Definition: G362P.h:111
void HardReset(void)
Definition: G362P.cc:68
#define REG_DIAG_STAT_L
Definition: G362P.h:34
uint8_t address
Definition: G362P.h:153
const char * name
Definition: G362P.h:155
#define REG_SMPL_CTRL_L
Definition: G362P.h:61
bool SetOutputBits(OutputBits temp, OutputBits gyro, OutputBits accl)
Definition: G362P.cc:236
#define REG_ZACCL_LOW
Definition: G362P.h:53
#define REG_GPIO_L
Definition: G362P.h:38
@ SPS500
Definition: G362P.h:132
double accl_[3]
Definition: G362P.h:143
@ FIR_TAP_64_FC_100
Definition: G362P.h:120
bool ReadData(Data *data)
Definition: G362P.cc:477
@ CONFIGURATION
Definition: G362P.h:94
#define REG_COUNT
Definition: G362P.h:39
@ FIR_TAP_128_FC_400
Definition: G362P.h:126
double gyro_[3]
Definition: G362P.h:142
@ FIR_TAP_64_FC_50
Definition: G362P.h:119
@ WIN0
Definition: G362P.h:98
@ SAMPLING
Definition: G362P.h:93
@ FIR_TAP_128_FC_50
Definition: G362P.h:123
#define REG_YACCL_LOW
Definition: G362P.h:51
#define REG_FILTER_CTRL_H
Definition: G362P.h:62
@ FIR_TAP_32_FC_400
Definition: G362P.h:118
#define REG_FILTER_CTRL_L
Definition: G362P.h:63
#define REG_ZGYRO_HIGH
Definition: G362P.h:46
#define REG_XGYRO_LOW
Definition: G362P.h:43
uint16_t TransferWord(uint16_t word, uint16_t delay_usecs)
Definition: G362P.cc:500
#define REG_WIN_CTRL_H
Definition: G362P.h:72
uint16_t count_
Definition: G362P.h:145
#define REG_GLOB_CMD_L
Definition: G362P.h:67
#define REG_YACCL_HIGH
Definition: G362P.h:50
uint16_t flag_
Definition: G362P.h:140
@ B32
Definition: G362P.h:104
#define REG_MODE_CTRL_H
Definition: G362P.h:31
Window
Definition: G362P.h:97
#define REG_TEMP_LOW
Definition: G362P.h:41