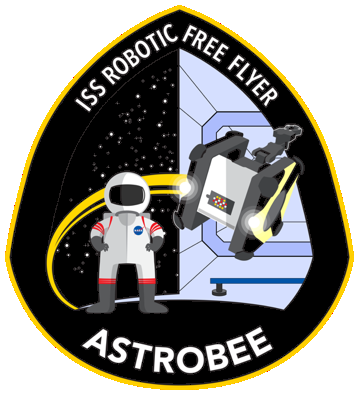 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_BASIC_TRAJ_DATA_H_
20 #define TRAJ_OPT_BASIC_TRAJ_DATA_H_
53 std::vector<PolynomialData>
segs;
63 std::vector<SplineData>
data;
86 #endif // TRAJ_OPT_BASIC_TRAJ_DATA_H_
std::vector< std::string > dimension_names
Definition: traj_data.h:64
PolyType
Definition: traj_data.h:27
PolynomialData(int d)
Definition: traj_data.h:47
@ LEGENDRE
Definition: traj_data.h:29
std::vector< float > slack
Definition: traj_data.h:78
std::vector< float > cost_history
Definition: traj_data.h:76
Definition: traj_data.h:61
SplineData()
Definition: traj_data.h:56
std::vector< PolynomialData > segs
Definition: traj_data.h:53
std::vector< float > gap_history
Definition: traj_data.h:75
std::vector< std::vector< float > > var_history
Definition: traj_data.h:81
PolyType basis
Definition: traj_data.h:40
SplineData(int deg, int seg)
Definition: traj_data.h:57
std::vector< std::vector< float > > time_history
Definition: traj_data.h:82
Definition: traj_data.h:49
std::vector< SplineData > data
Definition: traj_data.h:63
Definition: traj_data.h:74
float dt
Definition: traj_data.h:39
Definition: msg_traj.h:27
int segments
Definition: traj_data.h:50
@ STANDARD
Definition: traj_data.h:28
@ CHEBYSHEV
Definition: traj_data.h:34
@ WATTERSON
Definition: traj_data.h:30
@ ENDPOINT
Definition: traj_data.h:32
float cost
Definition: traj_data.h:79
std::vector< float > coeffs
Definition: traj_data.h:41
TrajData(int dim, int segs, int deg)
Definition: traj_data.h:71
float gap
Definition: traj_data.h:80
@ LIU
Definition: traj_data.h:33
int degree
Definition: traj_data.h:38
int iterations
Definition: traj_data.h:77
float t_total
Definition: traj_data.h:51
int dimensions
Definition: traj_data.h:62
@ BEZIER
Definition: traj_data.h:31
PolynomialData()
Definition: traj_data.h:46
Definition: traj_data.h:37
TrajData()
Definition: traj_data.h:70