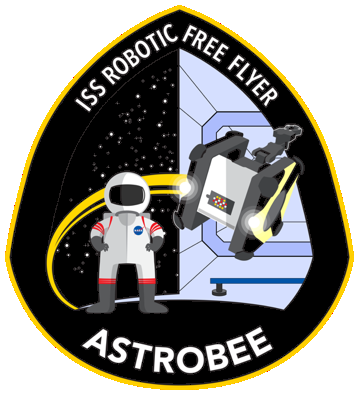 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef CALIBRATION_STATE_PARAMETERS_H_
19 #define CALIBRATION_STATE_PARAMETERS_H_
26 #include <Eigen/Geometry>
60 return optimized_state_parameters;
64 std::vector<Eigen::Isometry3d> optimized_camera_T_targets;
67 return optimized_camera_T_targets;
89 #endif // CALIBRATION_STATE_PARAMETERS_H_
Eigen::Isometry3d Isometry3d(const Eigen::Matrix< double, 6, 1 > &isometry_vector)
Definition: utilities.cc:39
#define LogInfo(msg)
Definition: logger.h:41
Definition: state_parameters.h:76
Eigen::Vector2d principal_points
Definition: state_parameters.h:39
Eigen::MatrixXd distortion
Definition: state_parameters.h:85
void Print()
Definition: state_parameters.h:32
Eigen::Vector2d focal_lengths
Definition: state_parameters.h:38
Eigen::VectorXd distortion
Definition: state_parameters.h:72
Eigen::Isometry3d Isometry3d(const Eigen::Vector3d &translation, const RotationType &rotation)
Definition: utilities.h:226
std::vector< Eigen::Matrix< double, 6, 1 > > camera_T_targets
Definition: state_parameters.h:73
Eigen::Matrix< double, 6, 1 > VectorFromIsometry3d(const Eigen::Isometry3d &isometry_3d)
Definition: utilities.cc:22
Definition: state_parameters.h:31
std::vector< Eigen::Isometry3d > OptimizedCameraTTargets() const
Definition: state_parameters.h:63
void AddCameraTTarget(const Eigen::Isometry3d &camera_T_target)
Definition: state_parameters.h:51
Eigen::Matrix2d focal_lengths
Definition: state_parameters.h:83
Eigen::Matrix2d principal_points
Definition: state_parameters.h:84
void Print()
Definition: state_parameters.h:77
Eigen::Vector2d focal_lengths
Definition: state_parameters.h:70
void SetInitialStateParameters(const StateParameters &initial_state_parameters)
Definition: state_parameters.h:45
Eigen::Vector2d principal_points
Definition: state_parameters.h:71
Definition: camera_target_based_intrinsics_calibrator.h:42
Definition: state_parameters.h:44
Eigen::VectorXd distortion
Definition: state_parameters.h:40
StateParameters OptimizedStateParameters() const
Definition: state_parameters.h:55