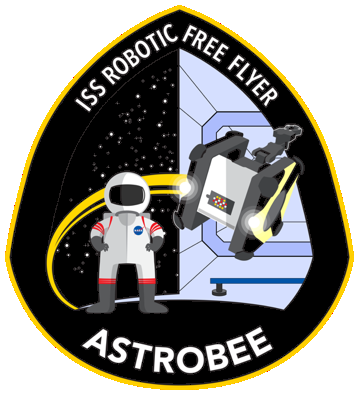 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef SMART_DOCK_SMART_DOCK_H_
20 #define SMART_DOCK_SMART_DOCK_H_
37 static constexpr uint8_t
OFF = 0;
38 static constexpr uint8_t
ON = 1;
57 uint8_t serial_number[6];
61 uint8_t actuator_state[2];
62 uint8_t conn_state[2];
219 bool GetStrings(uint32_t
mask, std::map<String, std::string> & data);
268 void Sleep(uint32_t microseconds);
271 uint16_t
Read(uint8_t *buff);
274 uint16_t
Read(uint8_t *buff, uint16_t len);
277 uint16_t
Write(uint8_t *buff, uint16_t len);
288 #endif // SMART_DOCK_SMART_DOCK_H_
@ FAULT_OC_BERTH_2
Definition: smart_dock.h:152
@ FAULT_WDT2_DOCKCTL_REBOOT
Definition: smart_dock.h:159
static constexpr uint8_t I2C_CMD_GET_SW_STATES
Definition: smart_dock.h:239
@ ACT_DEPLOYED
Definition: smart_dock.h:52
uint8_t dock_state
Definition: smart_dock.h:75
@ HK_AS1_I
Definition: smart_dock.h:176
@ STRING_SW_VERSION
Definition: smart_dock.h:106
@ NUM_CONNECTION_STATES
Definition: smart_dock.h:45
static constexpr uint8_t I2C_CMD_CLR_HW_EXCEPTIONS
Definition: smart_dock.h:244
static constexpr uint8_t I2C_CMD_CLR_TERMINATE_EVT
Definition: smart_dock.h:251
@ CHANNEL_RESERVED3
Definition: smart_dock.h:121
static constexpr uint8_t I2C_CMD_GET_CONN_STATE
Definition: smart_dock.h:259
@ COMMAND_ENABLE_ALL_PMCS
Definition: smart_dock.h:91
@ CHANNEL_RESERVED5
Definition: smart_dock.h:128
static constexpr uint8_t I2C_RESP_ACK
Definition: smart_dock.h:232
static constexpr uint8_t I2C_CMD_GET_SERIAL_NUMBER
Definition: smart_dock.h:246
bool GetHousekeeping(uint32_t mask, std::map< Housekeeping, double > &data)
Definition: smart_dock.cc:304
@ NUM_CHANNELS
Definition: smart_dock.h:146
~SmartDock(void)
Definition: smart_dock.cc:34
bool SetLeds(uint32_t mask, LedMode const value)
Definition: smart_dock.cc:320
@ CHANNEL_LED_5
Definition: smart_dock.h:134
uint8_t charge_mask
Definition: smart_dock.h:74
static constexpr size_t I2C_BUF_MAX_LEN
Definition: smart_dock.h:231
@ HK_AS2_I
Definition: smart_dock.h:175
@ CONN_DISCONNECTED
Definition: smart_dock.h:42
static constexpr uint8_t I2C_CMD_SET_EPS_POWER_MODE
Definition: smart_dock.h:256
@ CHANNEL_RESERVED4
Definition: smart_dock.h:127
@ CHANNEL_LED_2
Definition: smart_dock.h:131
@ FAULT_OT_CHARGER
Definition: smart_dock.h:155
@ LED_2
Definition: smart_dock.h:189
Definition: smart_dock.h:56
@ CHANNEL_DEV1_PWR_EN
Definition: smart_dock.h:117
@ STRING_BUILD
Definition: smart_dock.h:107
bool GetSystemState(DockState &data)
Definition: smart_dock.cc:294
static constexpr uint8_t I2C_CMD_UNDOCK
Definition: smart_dock.h:252
uint32_t fault_mask
Definition: smart_dock.h:77
@ HK_EC_PWR_I
Definition: smart_dock.h:173
static constexpr uint8_t I2C_CMD_GET_HW_EXCEPTIONS
Definition: smart_dock.h:243
@ HK_FAN_MAG_I
Definition: smart_dock.h:167
Definition: smart_dock.h:30
@ CHANNEL_ACT_SIGOUT1
Definition: smart_dock.h:123
@ HK_DEV1_I
Definition: smart_dock.h:182
@ CHANNEL_LED_4
Definition: smart_dock.h:133
Led
Definition: smart_dock.h:187
@ CHANNEL_ACT_SIGOUT2
Definition: smart_dock.h:122
static constexpr uint8_t I2C_CMD_GET_BUILD_TIME
Definition: smart_dock.h:236
uint32_t channel_mask
Definition: smart_dock.h:73
static constexpr size_t kDockStateLength
Definition: smart_dock.h:265
@ CHANNEL_LED_6
Definition: smart_dock.h:135
@ CHANNEL_LED_1
Definition: smart_dock.h:130
@ LED_3
Definition: smart_dock.h:190
static constexpr uint8_t ON
Definition: smart_dock.h:38
Fault
Definition: smart_dock.h:150
SmartDock(const i2c::Device &i2c_dev)
Definition: smart_dock.cc:32
static constexpr uint8_t I2C_RESP_NACK
Definition: smart_dock.h:233
@ NUM_BERTHS
Definition: smart_dock.h:101
static constexpr uint8_t I2C_CMD_NONE
Definition: smart_dock.h:234
bool ClearFaults(void)
Definition: smart_dock.cc:45
@ COMMAND_CLEAR_TERMINATE
Definition: smart_dock.h:87
@ CHANNEL_RESERVED13
Definition: smart_dock.h:142
@ NUM_FAULTS
Definition: smart_dock.h:162
@ CHANNEL_RESERVED0
Definition: smart_dock.h:118
@ CHANNEL_RESERVED7
Definition: smart_dock.h:136
uint8_t power_state
Definition: smart_dock.h:70
uint8_t led_state
Definition: smart_dock.h:60
@ COMMAND_SET_POWER_MODE_AWAKE_SAFE
Definition: smart_dock.h:85
@ BERTH_2
Definition: smart_dock.h:100
@ HK_DEV2_T_PROTECT
Definition: smart_dock.h:179
uint8_t mask
Definition: signal_lights.h:72
static constexpr uint8_t I2C_CMD_GET_SYSTEM_STATE
Definition: smart_dock.h:240
@ CHANNEL_RESERVED6
Definition: smart_dock.h:129
static constexpr uint8_t I2C_CMD_GET_EPS_POWER_MODE
Definition: smart_dock.h:257
@ HK_DEV2_I
Definition: smart_dock.h:181
uint16_t Read(uint8_t *buff)
Definition: smart_dock.cc:400
@ CHANNEL_DEV_EN
Definition: smart_dock.h:114
@ COMMAND_SET_POWER_MODE_AWAKE_NOMINAL
Definition: smart_dock.h:84
@ CHANNEL_RESERVED9
Definition: smart_dock.h:138
@ HK_A_GND_V1
Definition: smart_dock.h:174
@ CHANNEL_FAN_EN
Definition: smart_dock.h:126
static constexpr uint8_t I2C_CMD_SET_LED_MODES
Definition: smart_dock.h:241
@ COMMAND_CLEAR_FAULTS
Definition: smart_dock.h:88
Definition: eps_driver.h:234
ConnectionState
Definition: smart_dock.h:41
uint32_t flags
Definition: smart_dock.h:64
@ FAULT_OC_BERTH_1
Definition: smart_dock.h:151
static constexpr uint8_t OFF
Definition: smart_dock.h:37
@ FAULT_WDT3_DOCKPC_REBOOT
Definition: smart_dock.h:160
@ CHANNEL_EC_PWR_EN
Definition: smart_dock.h:115
@ FAULT_OC_DOCK_PROCESSOR
Definition: smart_dock.h:154
static constexpr uint8_t DOCK_BERTH_ID_MIN
Definition: smart_dock.h:263
static constexpr uint8_t I2C_CMD_REBOOT
Definition: smart_dock.h:247
@ FAULT_OT_ACTUATOR_2
Definition: smart_dock.h:157
@ FAULT_WDT4_DOCKCTL_REBOOT
Definition: smart_dock.h:161
@ COMMAND_SET_POWER_MODE_CRITICAL_FAULT
Definition: smart_dock.h:86
Definition: smart_dock.h:35
static constexpr uint8_t I2C_CMD_SET_CHARGE_STATE
Definition: smart_dock.h:254
@ COMMAND_UNKNOWN
Definition: smart_dock.h:82
String
Definition: smart_dock.h:105
@ NUM_LEDS
Definition: smart_dock.h:194
@ CHANNEL_RESERVED8
Definition: smart_dock.h:137
@ CHANNEL_RESERVED14
Definition: smart_dock.h:143
@ LED_MODE_BLINK_0_5HZ
Definition: smart_dock.h:203
uint8_t subsys_1_pwr
Definition: smart_dock.h:58
@ LED_4
Definition: smart_dock.h:191
static constexpr uint8_t I2C_CMD_GET_CONNECTED_EPS_STATE
Definition: smart_dock.h:258
@ HK_VLIVE_I
Definition: smart_dock.h:170
@ LED_1
Definition: smart_dock.h:188
bool GetStrings(uint32_t mask, std::map< String, std::string > &data)
Definition: smart_dock.cc:179
@ NUM_STRINGS
Definition: smart_dock.h:109
@ LED_MODE_BLINK_2HZ
Definition: smart_dock.h:201
static constexpr uint8_t I2C_CMD_ENTER_BOOTLOADER
Definition: smart_dock.h:248
@ CONN_CONNECTED
Definition: smart_dock.h:44
@ STRING_SERIAL
Definition: smart_dock.h:108
uint8_t terminate
Definition: smart_dock.h:72
@ NUM_COMMANDS
Definition: smart_dock.h:94
@ HK_A_GND_V3
Definition: smart_dock.h:180
@ HK_DEV1_T_PROTECT
Definition: smart_dock.h:178
bool GetFaults(uint32_t mask, std::map< Fault, bool > &data)
Definition: smart_dock.cc:224
@ HK_A_GND_V2
Definition: smart_dock.h:177
Channel
Definition: smart_dock.h:113
Housekeeping
Definition: smart_dock.h:166
@ NUM_LED_MODES
Definition: smart_dock.h:204
uint16_t Write(uint8_t *buff, uint16_t len)
Definition: smart_dock.cc:434
@ CHANNEL_DEV2_PWR_EN
Definition: smart_dock.h:116
uint8_t ComputeChecksum(uint8_t *buf, size_t size)
Definition: smart_dock.cc:448
@ CHANNEL_RESERVED15
Definition: smart_dock.h:144
static constexpr uint8_t I2C_CMD_GET_BATTERY_STATUS
Definition: smart_dock.h:249
static constexpr uint8_t I2C_CMD_GET_DIGITAL_TEMPS
Definition: smart_dock.h:245
Definition: smart_dock.h:69
@ COMMAND_SET_POWER_MODE_HIBERNATE
Definition: smart_dock.h:83
static constexpr uint8_t I2C_CMD_GET_HK
Definition: smart_dock.h:242
static constexpr uint8_t I2C_CMD_GET_CONNECTION_STATE
Definition: smart_dock.h:253
@ CHANNEL_RESERVED16
Definition: smart_dock.h:145
static constexpr uint8_t DOCK_BERTH_ID_MAX
Definition: smart_dock.h:264
@ LED_MODE_OFF
Definition: smart_dock.h:199
ActuatorState
Definition: smart_dock.h:49
void Sleep(uint32_t microseconds)
Definition: smart_dock.cc:357
@ COMMAND_DISABLE_ALL_PAYLOADS
Definition: smart_dock.h:90
@ COMMAND_DISABLE_ALL_PMCS
Definition: smart_dock.h:92
@ ACT_RETRACTING
Definition: smart_dock.h:51
@ FAULT_WDT1_EPS_REBOOT
Definition: smart_dock.h:158
static constexpr uint8_t I2C_CMD_GET_EPS_CMD
Definition: smart_dock.h:260
bool GetChannels(uint32_t mask, std::map< Channel, bool > &data)
Definition: smart_dock.cc:211
@ CHANNEL_AS1_PWR_EN
Definition: smart_dock.h:125
@ NUM_HOUSEKEEPING
Definition: smart_dock.h:183
static constexpr uint8_t I2C_CMD_SW_OFF
Definition: smart_dock.h:238
bool Reboot(void)
Definition: smart_dock.cc:39
@ COMMAND_REBOOT
Definition: smart_dock.h:93
@ CHANNEL_AS2_PWR_EN
Definition: smart_dock.h:124
@ NUM_BATTERIES
Definition: eps_driver.h:197
@ CHANNEL_LED_3
Definition: smart_dock.h:132
@ FAULT_OC_SYSTEM
Definition: smart_dock.h:153
@ LED_MODE_ON
Definition: smart_dock.h:200
@ CHANNEL_RESERVED10
Definition: smart_dock.h:139
eps_driver::EPS EPS
Definition: smart_dock.h:32
BerthCommand
Definition: smart_dock.h:81
@ ACT_RETRACT
Definition: smart_dock.h:50
Berth
Definition: smart_dock.h:98
bool SendBerthCommand(uint32_t mask, BerthCommand const value)
Definition: smart_dock.cc:51
@ HK_CHR_V_V
Definition: smart_dock.h:168
static constexpr uint8_t I2C_CMD_GET_CHARGE_STATE
Definition: smart_dock.h:255
bool GetBerthStates(uint32_t mask, std::map< Berth, BerthState > &data)
Definition: smart_dock.cc:236
bool SetChannels(uint32_t mask, bool const value)
Definition: smart_dock.cc:342
Definition: eps_driver.h:32
@ HK_MAIN5_PWR_I
Definition: smart_dock.h:171
@ LED_6
Definition: smart_dock.h:193
@ CHANNEL_RESERVED2
Definition: smart_dock.h:120
@ CHANNEL_RESERVED12
Definition: smart_dock.h:141
@ FAULT_OT_ACTUATOR_1
Definition: smart_dock.h:156
static constexpr uint8_t I2C_CMD_SW_ON
Definition: smart_dock.h:237
static constexpr uint8_t I2C_CMD_SET_EPS_CMD
Definition: smart_dock.h:261
@ CHANNEL_RESERVED11
Definition: smart_dock.h:140
static constexpr uint8_t I2C_CMD_GET_SW_VERSION
Definition: smart_dock.h:235
@ BERTH_1
Definition: smart_dock.h:99
@ LED_5
Definition: smart_dock.h:192
@ HK_CHR_T_PROTECT
Definition: smart_dock.h:169
uint8_t subsys_2_pwr
Definition: smart_dock.h:59
LedMode
Definition: smart_dock.h:198
static constexpr uint8_t I2C_CMD_SEND_EPS_STATE_TO_DOCK
Definition: smart_dock.h:262
@ COMMAND_ENABLE_ALL_PAYLOADS
Definition: smart_dock.h:89
@ CONN_CONNECTING
Definition: smart_dock.h:43
@ HK_DEV_I
Definition: smart_dock.h:172
@ LED_MODE_BLINK_1HZ
Definition: smart_dock.h:202
@ CHANNEL_RESERVED1
Definition: smart_dock.h:119
static constexpr uint8_t I2C_CMD_RING_BUZZER
Definition: smart_dock.h:250