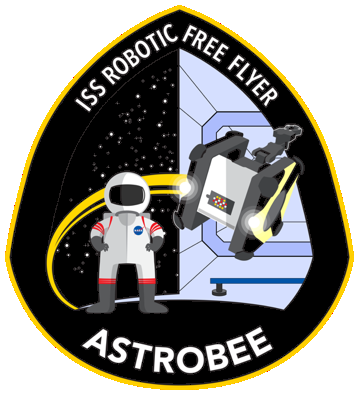 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
20 #ifndef EXECUTIVE_UTILS_SEQUENCER_SEQUENCER_H_
21 #define EXECUTIVE_UTILS_SEQUENCER_SEQUENCER_H_
23 #include <ros/console.h>
26 #include <ff_msgs/AgentStateStamped.h>
27 #include <ff_msgs/ControlState.h>
28 #include <ff_msgs/CommandStamped.h>
29 #include <ff_msgs/CompressedFile.h>
30 #include <ff_msgs/ControlFeedback.h>
31 #include <ff_msgs/PlanStatusStamped.h>
33 #include <geometry_msgs/InertiaStamped.h>
62 bool Feedback(ff_msgs::AckCompletedStatus
const& ack) noexcept;
65 void Feedback(ff_msgs::ControlFeedback
const& progress) noexcept;
68 ff_msgs::PlanStatusStamped
const&
plan_status() noexcept;
71 bool valid()
const noexcept;
79 geometry_msgs::InertiaStamped
GetInertia()
const noexcept;
83 int AppendStatus(ff_msgs::Status
const& msg) noexcept;
85 void Reset() noexcept;
87 friend bool LoadPlan(ff_msgs::CompressedFile::ConstPtr
const& cf,
93 ff_msgs::PlanStatusStamped status_;
99 int current_milestone_;
102 int current_command_;
109 int station_duration_;
116 std::vector<ff_msgs::ControlState>
121 #endif // EXECUTIVE_UTILS_SEQUENCER_SEQUENCER_H_
Definition: sequencer.h:49
ff_msgs::PlanStatusStamped const & plan_status() noexcept
Definition: sequencer.cc:366
jsonloader::Segment CurrentSegment() noexcept
Definition: sequencer.cc:238
bool HaveOperatingLimits() const noexcept
Definition: sequencer.cc:172
ff_msgs::CommandStamped::Ptr CurrentCommand() noexcept
Definition: sequencer.cc:250
ItemType CurrentType(bool reset_time=true) noexcept
Definition: sequencer.cc:196
bool GetOperatingLimits(ff_msgs::AgentStateStamped &state) const noexcept
Definition: sequencer.cc:176
geometry_msgs::InertiaStamped GetInertia() const noexcept
Definition: sequencer.cc:147
ItemType
Definition: sequencer.h:43
bool valid() const noexcept
Definition: sequencer.cc:362
bool LoadPlan(ff_msgs::CompressedFile::ConstPtr const &cf, Sequencer *seq)
Definition: sequencer.cc:85
bool HaveInertia() const noexcept
Definition: sequencer.cc:143
std::vector< ff_msgs::ControlState > Segment2Trajectory(jsonloader::Segment const &segment)
Definition: sequencer.cc:115
uint8_t state
Definition: signal_lights.h:90
bool Feedback(ff_msgs::AckCompletedStatus const &ack) noexcept
Definition: sequencer.cc:289
Sequencer()
Definition: sequencer.cc:124
double Time
Definition: time.h:23
jsonloader::Plan const & plan() const noexcept
Definition: sequencer.cc:139
Definition: command_conversion.h:41
friend bool LoadPlan(ff_msgs::CompressedFile::ConstPtr const &cf, Sequencer *seq)
Definition: sequencer.cc:85