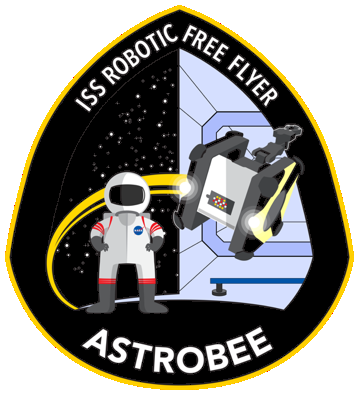 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_SAMPLED_TRAJECTORY_H_
20 #define MAPPER_SAMPLED_TRAJECTORY_H_
22 #include <geometry_msgs/Point.h>
23 #include <geometry_msgs/PointStamped.h>
24 #include <octomap/octomap.h>
25 #include <octomap/OcTree.h>
26 #include <visualization_msgs/MarkerArray.h>
27 #include <pcl/kdtree/kdtree_flann.h>
28 #include <pcl/kdtree/impl/kdtree_flann.hpp>
30 #include <Eigen/Dense>
50 pcl::PointCloud<pcl::PointXYZ>
pos_;
52 pcl::PointCloud<pcl::PointXYZ>::Ptr(
new pcl::PointCloud<pcl::PointXYZ>);
74 const pcl::PointCloud<pcl::PointXYZ> &pos_vec);
85 std::vector<octomap::point3d> *points);
90 void SortCollisions(
const std::vector<octomap::point3d> &colliding_nodes,
91 std::vector<geometry_msgs::PointStamped> *samples);
92 void TrajVisMarkers(visualization_msgs::MarkerArray* marker_array);
100 const geometry_msgs::PointStamped &sample2);
105 #endif // MAPPER_SAMPLED_TRAJECTORY_H_
int n_points_
Definition: sampled_trajectory.h:55
Definition: sampled_trajectory.h:38
void PrintSamples()
Definition: sampled_trajectory.cc:62
bool ComparePointStamped(const geometry_msgs::PointStamped &sample1, const geometry_msgs::PointStamped &sample2)
Definition: sampled_trajectory.cc:541
octomap::OcTree thick_traj_
Definition: sampled_trajectory.h:64
pcl::PointCloud< pcl::PointXYZ >::Ptr cloud_ptr_
Definition: sampled_trajectory.h:51
void SetResolution(const double &resolution)
Definition: sampled_trajectory.cc:75
double thickness_
Definition: sampled_trajectory.h:67
void SetMaxDev(const double &max_dev)
Definition: sampled_trajectory.cc:70
Definition: sampled_trajectory.h:47
void SamplesVisMarkers(visualization_msgs::MarkerArray *marker_array)
Definition: sampled_trajectory.cc:445
pcl::PointCloud< pcl::PointXYZ > pos_
Definition: sampled_trajectory.h:50
int n_compressed_points_
Definition: sampled_trajectory.h:60
void Bresenham(const Eigen::Vector3d &p0, const Eigen::Vector3d &pf, std::vector< octomap::point3d > *points)
Definition: sampled_trajectory.cc:169
void DeleteSample(const int &index)
Definition: sampled_trajectory.cc:83
void CreateKdTree()
Definition: sampled_trajectory.cc:364
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
void CompressedVisMarkers(visualization_msgs::MarkerArray *marker_array)
Definition: sampled_trajectory.cc:486
SampledTrajectory3D()
Definition: sampled_trajectory.cc:58
std::vector< double > compressed_time_
Definition: sampled_trajectory.h:59
void SortCollisions(const std::vector< octomap::point3d > &colliding_nodes, std::vector< geometry_msgs::PointStamped > *samples)
Definition: sampled_trajectory.cc:369
double resolution_
Definition: sampled_trajectory.h:66
pcl::PointCloud< pcl::PointXYZ > point_cloud_traj_
Definition: sampled_trajectory.h:65
std::vector< Eigen::Vector3d > compressed_pos_
Definition: sampled_trajectory.h:58
double max_dev_
Definition: sampled_trajectory.h:61
void CompressSamples()
Definition: sampled_trajectory.cc:89
pcl::KdTreeFLANN< pcl::PointXYZ > kdtree_pos_
Definition: sampled_trajectory.h:53
void TrajVisMarkers(visualization_msgs::MarkerArray *marker_array)
Definition: sampled_trajectory.cc:393
void ClearObject()
Definition: sampled_trajectory.cc:528
Definition: polynomials.h:94
std::vector< double > time_
Definition: sampled_trajectory.h:54
void ThickBresenham(const Eigen::Vector3d &p0, const Eigen::Vector3d &pf)
Definition: sampled_trajectory.cc:287
void ThickTrajToPcl()
Definition: sampled_trajectory.cc:349