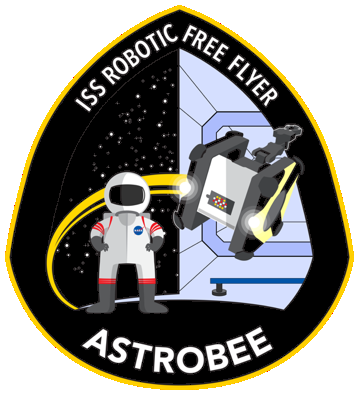 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef PMC_ACTUATOR_PMC_ACTUATOR_H_
20 #define PMC_ACTUATOR_PMC_ACTUATOR_H_
79 uint8_t motor_current;
133 bool metadata_received_;
134 uint16_t metadata_index_;
161 uint8_t ComputeChecksum(
const uint8_t *buf,
size_t size);
167 std::fstream &cmds_output_;
168 std::fstream &telem_output_;
174 std::fstream &telem_out);
197 #endif // PMC_ACTUATOR_PMC_ACTUATOR_H_
uint8_t mode
Definition: signal_lights.h:74
bool GetFirmwareType(uint8_t &type)
Definition: pmc_actuator.cc:134
constexpr uint8_t kMetadataTypePrototype
Definition: pmc_actuator.h:44
uint8_t errOvrCurrent
Definition: pmc_actuator.h:69
constexpr size_t kGitHashLength
Definition: pmc_actuator.h:41
i2c::Address GetAddress()
Definition: pmc_actuator.cc:148
constexpr size_t kTemperatureSensorsCount
Definition: pmc_actuator.h:39
std::uint16_t Address
Definition: i2c_new.h:31
constexpr size_t kMaxMetadataLength
Definition: pmc_actuator.h:43
virtual bool GetFirmwareTime(std::string &date)=0
bool GetFirmwareTime(std::string &date)
Definition: pmc_actuator.cc:207
Definition: pmc_actuator.h:29
uint8_t reserved
Definition: pmc_actuator.h:73
@ SHUTDOWN
Definition: pmc_actuator.h:31
uint8_t mcDisabled
Definition: pmc_actuator.h:60
uint8_t mode
Definition: pmc_actuator.h:58
struct __attribute__((packed))
Definition: pmc_actuator.h:47
Command
Definition: pmc_actuator.h:53
constexpr size_t kTelemetryMsgLength
Definition: pmc_actuator.h:40
Telemetry
Definition: pmc_actuator.h:87
bool GetFirmwareHash(std::string &hash)
Definition: pmc_actuator.cc:112
virtual bool GetFirmwareHash(std::string &hash)=0
uint8_t control
Definition: pmc_actuator.h:71
virtual ~PmcActuatorStub()
Definition: pmc_actuator.cc:158
constexpr size_t kCommandMsgLength
Definition: pmc_actuator.h:38
bool GetTelemetry(Telemetry *telemetry)
Definition: pmc_actuator.cc:58
Definition: pmc_actuator.h:104
uint8_t errNozCmdOOR
Definition: pmc_actuator.h:62
@ NORMAL
Definition: pmc_actuator.h:31
constexpr size_t kDateLength
Definition: pmc_actuator.h:42
MetadataVersion
Definition: pmc_actuator.h:95
bool GetFirmwareTime(std::string &date)
Definition: pmc_actuator.cc:123
virtual bool SendCommand(const Command &command)=0
PmcActuator(const i2c::Device &i2c_dev)
Definition: pmc_actuator.cc:30
uint8_t speedCamState
Definition: pmc_actuator.h:70
bool GetFirmwareType(uint8_t &type)
Definition: pmc_actuator.cc:213
uint8_t stateStatus
Definition: pmc_actuator.h:59
uint8_t checksum(uint8_t *buf, size_t len)
Definition: fam_cmd_i2c_node.cc:53
void PrintTelemetry(std::ostream &out, const Telemetry &telem)
Definition: pmc_actuator.cc:222
constexpr int kNumNozzles
Definition: pmc_actuator.h:37
virtual bool GetFirmwareType(uint8_t &type)=0
@ RESTART
Definition: pmc_actuator.h:31
uint8_t asUint8
Definition: pmc_actuator.h:67
uint8_t metadata
Definition: pmc_actuator.h:72
bool GetTelemetry(Telemetry *telemetry)
Definition: pmc_actuator.cc:183
bool GetFirmwareHash(std::string &hash)
Definition: pmc_actuator.cc:201
CmdMode
Definition: pmc_actuator.h:31
bool SendCommand(const Command &command)
Definition: pmc_actuator.cc:35
virtual bool GetTelemetry(Telemetry *telemetry)=0
uint8_t asUint8
Definition: pmc_actuator.h:56
Definition: pmc_actuator.h:164
uint8_t errBadCRC
Definition: pmc_actuator.h:61
virtual i2c::Address GetAddress()=0
virtual ~PmcActuator(void)
Definition: pmc_actuator.cc:33
PmcActuatorStub(int addr, std::fstream &cmds_out, std::fstream &telem_out)
Definition: pmc_actuator.cc:152
i2c::Address GetAddress()
Definition: pmc_actuator.cc:218
constexpr uint8_t kMetadataTypeFlight
Definition: pmc_actuator.h:45
bool SendCommand(const Command &command)
Definition: pmc_actuator.cc:162
Definition: pmc_actuator.h:130