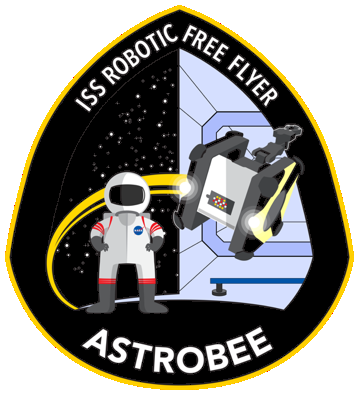 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef ASTROBEE_GAZEBO_ASTROBEE_GAZEBO_H_
20 #define ASTROBEE_GAZEBO_ASTROBEE_GAZEBO_H_
24 #include <ros/callback_queue.h>
27 #include <geometry_msgs/TransformStamped.h>
28 #include <sensor_msgs/PointCloud2.h>
29 #include <sensor_msgs/point_cloud2_iterator.h>
30 #include <sensor_msgs/Image.h>
31 #include <sensor_msgs/image_encodings.h>
32 #include <sensor_msgs/CameraInfo.h>
35 #include <tf2_ros/transform_listener.h>
41 #include <gazebo/common/common.hh>
42 #include <gazebo/physics/physics.hh>
43 #include <gazebo/sensors/sensors.hh>
44 #if GAZEBO_MAJOR_VERSION <= 7
45 #include <gazebo/math/gzmath.hh>
47 #include <gazebo/rendering/rendering.hh>
50 #include <Eigen/Geometry>
64 std::string
const& plugin_frame,
bool send_heartbeats =
false);
71 void InitializePlugin(std::string
const& robot_name, std::string
const& plugin_name);
77 std::string
GetFrame(std::string target =
"", std::string delim =
"/");
81 geometry_msgs::TransformStamped
const* tf) = 0;
96 std::shared_ptr<tf2_ros::TransformListener>
listener_;
108 std::string
const& plugin_frame,
bool send_heartbeats =
false);
115 virtual void Load(physics::ModelPtr model, sdf::ElementPtr sdf);
128 physics::ModelPtr model, sdf::ElementPtr sdf) = 0;
134 sdf::ElementPtr sdf_;
135 physics::LinkPtr link_;
136 physics::WorldPtr world_;
137 physics::ModelPtr model_;
146 std::string
const& plugin_frame,
bool send_heartbeats =
false);
153 void Load(sensors::SensorPtr sensor, sdf::ElementPtr sdf);
166 sensors::SensorPtr sensor, sdf::ElementPtr sdf) = 0;
172 sensors::SensorPtr sensor_;
173 physics::WorldPtr world_;
174 physics::ModelPtr model_;
175 sdf::ElementPtr sdf_;
181 #if GAZEBO_MAJOR_VERSION > 7
183 ignition::math::Pose3d
const& sensor_pose);
186 ignition::math::Pose3d
const& sensor_pose);
194 #endif // ASTROBEE_GAZEBO_ASTROBEE_GAZEBO_H_
ros::CallbackQueue callback_queue_
Definition: astrobee_gazebo.h:97
std::shared_ptr< tf2_ros::TransformListener > listener_
Definition: astrobee_gazebo.h:96
ros::NodeHandle nh_ff_mt_
Definition: astrobee_gazebo.h:95
Definition: astrobee_gazebo.h:57
physics::WorldPtr GetWorld()
Definition: astrobee_gazebo.cc:223
Definition: camera_model.h:26
physics::WorldPtr GetWorld()
Definition: astrobee_gazebo.cc:148
Definition: astrobee_gazebo.h:104
std::string plugin_name_
Definition: astrobee_gazebo.h:94
virtual void Load(physics::ModelPtr model, sdf::ElementPtr sdf)
Definition: astrobee_gazebo.cc:116
virtual ~FreeFlyerModelPlugin()
Definition: astrobee_gazebo.cc:113
void Load(sensors::SensorPtr sensor, sdf::ElementPtr sdf)
Definition: astrobee_gazebo.cc:190
ros::NodeHandle nh_
Definition: astrobee_gazebo.h:95
virtual void LoadCallback(ros::NodeHandle *nh, physics::ModelPtr model, sdf::ElementPtr sdf)=0
virtual bool ExtrinsicsCallback(geometry_msgs::TransformStamped const *tf)
Definition: astrobee_gazebo.cc:158
ros::NodeHandle nh_ff_
Definition: astrobee_gazebo.h:95
std::string parent_frame_
Definition: astrobee_gazebo.h:94
virtual void LoadCallback(ros::NodeHandle *nh, sensors::SensorPtr sensor, sdf::ElementPtr sdf)=0
Definition: astrobee_gazebo.h:60
virtual void OnExtrinsicsReceived(ros::NodeHandle *nh)
Definition: astrobee_gazebo.h:84
void InitializePlugin(std::string const &robot_name, std::string const &plugin_name)
Definition: astrobee_gazebo.cc:47
virtual ~FreeFlyerPlugin()
Definition: astrobee_gazebo.cc:36
std::string GetRotationType()
physics::ModelPtr GetModel()
Definition: astrobee_gazebo.cc:153
Definition: ff_nodelet.h:57
std::thread thread_
Definition: astrobee_gazebo.h:98
FreeFlyerModelPlugin(std::string const &plugin_name, std::string const &plugin_frame, bool send_heartbeats=false)
Definition: astrobee_gazebo.cc:107
Definition: astrobee_gazebo.h:142
physics::LinkPtr GetLink()
Definition: astrobee_gazebo.cc:143
std::string GetFrame(std::string target="", std::string delim="/")
Definition: astrobee_gazebo.cc:99
virtual ~FreeFlyerSensorPlugin()
Definition: astrobee_gazebo.cc:187
FreeFlyerPlugin(std::string const &plugin_name, std::string const &plugin_frame, bool send_heartbeats=false)
Definition: astrobee_gazebo.cc:29
Eigen::Affine3d SensorToWorld(gazebo::math::Pose const &world_pose, ignition::math::Pose3d const &sensor_pose)
Definition: astrobee_gazebo.cc:328
void SetupExtrinsics(const ros::TimerEvent &event)
Definition: astrobee_gazebo.cc:77
void SetParentFrame(std::string const &parent)
Definition: astrobee_gazebo.cc:42
Eigen::Affine3d Affine3d(const Eigen::Matrix< double, 7, 1 > &affine_vector)
Definition: utilities.cc:43
FreeFlyerSensorPlugin(std::string const &plugin_name, std::string const &plugin_frame, bool send_heartbeats=false)
Definition: astrobee_gazebo.cc:181
tf2_ros::Buffer buffer_
Definition: astrobee_gazebo.h:100
virtual bool ExtrinsicsCallback(geometry_msgs::TransformStamped const *tf)
Definition: astrobee_gazebo.cc:233
virtual bool ExtrinsicsCallback(geometry_msgs::TransformStamped const *tf)=0
void FillCameraInfo(rendering::CameraPtr camera, sensor_msgs::CameraInfo &info_msg)
Definition: astrobee_gazebo.cc:350
void CallbackThread()
Definition: astrobee_gazebo.cc:71
std::string robot_name_
Definition: astrobee_gazebo.h:94
physics::ModelPtr GetModel()
Definition: astrobee_gazebo.cc:228
ros::Timer timer_
Definition: astrobee_gazebo.h:99
std::string plugin_frame_
Definition: astrobee_gazebo.h:94