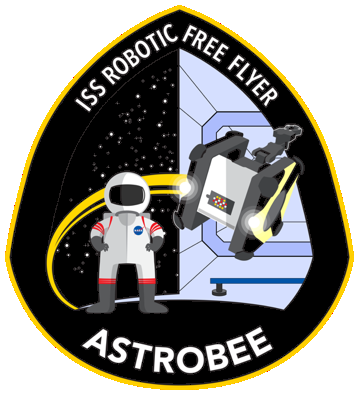 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef DDS_ROS_BRIDGE_ASTROBEE_ASTROBEE_BRIDGE_H_
20 #define DDS_ROS_BRIDGE_ASTROBEE_ASTROBEE_BRIDGE_H_
22 #include <pluginlib/class_list_macros.h>
32 #include "dds_ros_bridge/rapid_sub_ros_pub.h"
33 #include "dds_ros_bridge/ros_sub_rapid_pub.h"
40 #include "ff_msgs/ResponseOnly.h"
46 #include "knDds/DdsSupport.h"
47 #include "knDds/DdsEntitiesFactory.h"
48 #include "knDds/DdsEntitiesFactorySvc.h"
51 #include "miro/Configuration.h"
52 #include "miro/Robot.h"
56 class DdsEntitiesFactorySvc;
68 template<
typename T,
typename ... Args>
71 template<
typename T,
typename ... Args>
78 std::string &topic_name,
bool &enable,
float &rate);
82 bool Start(ff_msgs::ResponseOnly::Request& req,
83 ff_msgs::ResponseOnly::Response& res);
92 ros::ServiceServer trigger_srv_;
94 std::map<std::string, ff::RosSubRapidPubPtr> ros_sub_rapid_pubs_;
95 std::shared_ptr<kn::DdsEntitiesFactorySvc> dds_entities_factory_;
96 std::string agent_name_, participant_name_, read_params_error_;
97 std::vector<ff::RapidPubPtr> rapid_pubs_;
98 std::vector<ff::RapidSubRosPubPtr> rapid_sub_ros_pubs_;
103 #endif // DDS_ROS_BRIDGE_ASTROBEE_ASTROBEE_BRIDGE_H_
int BuildRosToRapid(const std::string &name, Args &&... args)
Definition: astrobee_astrobee_bridge.cc:380
AstrobeeAstrobeeBridge()
Definition: astrobee_astrobee_bridge.cc:22
bool ReadSharedItemConf(config_reader::ConfigReader::Table &conf, std::string &topic_name, bool &enable, float &rate)
Definition: astrobee_astrobee_bridge.cc:357
~AstrobeeAstrobeeBridge()
Definition: astrobee_astrobee_bridge.cc:30
bool ReadParams()
Definition: astrobee_astrobee_bridge.cc:206
std::string name
Definition: eps_simulator.cc:48
Definition: smart_dock_node.h:55
int BuildRapidToRos(Args &&... args)
Definition: astrobee_astrobee_bridge.cc:389
Definition: ff_nodelet.h:57
Definition: config_reader.h:48
virtual void Initialize(ros::NodeHandle *nh)
Definition: astrobee_astrobee_bridge.cc:33
Definition: config_reader.h:54
Definition: astrobee_astrobee_bridge.h:59
Definition: astrobee_astrobee_bridge.h:63