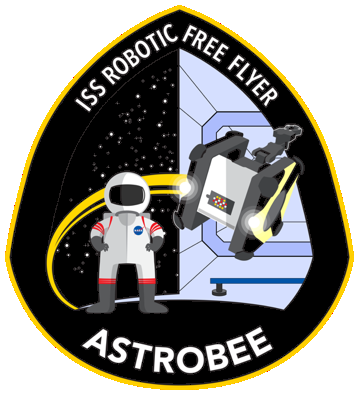 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
20 #ifndef SMART_DOCK_SMART_DOCK_NODE_H_
21 #define SMART_DOCK_SMART_DOCK_NODE_H_
31 #include "dds_msgs/AstrobeeConstants.h"
35 #include "dds_msgs/DockStateSupport.h"
37 #include "knDds/DdsEntitiesFactory.h"
38 #include "knDds/DdsEntitiesFactorySvc.h"
39 #include "knDds/DdsEventLoop.h"
40 #include "knDds/DdsSupport.h"
41 #include "knDds/DdsTypedSupplier.h"
43 #include "miro/Configuration.h"
44 #include "miro/Robot.h"
47 #include "rapidDds/RapidConstants.h"
48 #include "rapidDds/AckSupport.h"
49 #include "rapidDds/CommandSupport.h"
51 #include "rapidUtil/RapidHelper.h"
56 class DdsEntitiesFactorySvc;
64 std::string
const&
name);
79 bool AddDevice(std::string
const& serial, std::string
const&
name);
84 rapid::AckStatus status = rapid::ACK_COMPLETED,
85 rapid::AckCompletedStatus completed_status =
86 rapid::ACK_COMPLETED_OK,
87 std::string
const& message =
"");
93 using AckSupplier = kn::DdsTypedSupplier<rapid::Ack>;
94 using DockSupplier = kn::DdsTypedSupplier<rapid::ext::astrobee::DockState>;
98 std::map<std::string, std::string> devices_;
100 std::string ack_pub_suffix_, dock_state_pub_suffix_, sub_suffix_;
107 std::shared_ptr<kn::DdsEntitiesFactorySvc> dds_entities_factory_;
109 DockSupplier *dock_state_supplier_;
111 AckSupplier *ack_state_supplier_;
113 kn::DdsEventLoop dds_event_loop_;
118 #endif // SMART_DOCK_SMART_DOCK_NODE_H_
~SmartDockNode()
Definition: smart_dock_node.cc:126
Definition: smart_dock_node.h:60
void operator()(rapid::Command const *cmd)
Definition: smart_dock_node.cc:128
Definition: smart_dock.h:30
void ProcessComms()
Definition: smart_dock_node.cc:184
int64_t MakeTimestamp()
Definition: smart_dock_node.cc:258
Command
Definition: pmc_actuator.h:53
std::string name
Definition: eps_simulator.cc:48
Definition: smart_dock.h:35
Definition: smart_dock_node.h:55
void ProcessTelem()
Definition: smart_dock_node.cc:188
SmartDockNode(int argc, char **argv, const i2c::Device &i2c_dev, std::string const &name)
Definition: smart_dock_node.cc:55
bool AddDevice(std::string const &serial, std::string const &name)
Definition: smart_dock_node.cc:177
void PublishCmdAck(std::string const &cmd_id, rapid::AckStatus status=rapid::ACK_COMPLETED, rapid::AckCompletedStatus completed_status=rapid::ACK_COMPLETED_OK, std::string const &message="")
Definition: smart_dock_node.cc:266