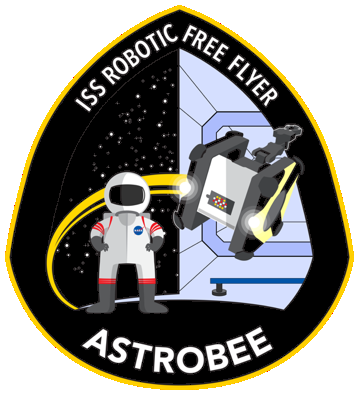 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_BASIC_MSG_TRAJ_H_
20 #define TRAJ_OPT_BASIC_MSG_TRAJ_H_
39 std::vector<std::vector<boost::shared_ptr<Poly>>>
polyies_;
40 std::vector<std::vector<std::vector<boost::shared_ptr<Poly>>>>
derrives_;
42 std::vector<decimal_t>
dts;
46 #endif // TRAJ_OPT_BASIC_MSG_TRAJ_H_
Definition: msg_traj.h:28
uint num_secs_
Definition: msg_traj.h:41
Definition: trajectory.h:35
std::vector< std::vector< boost::shared_ptr< Poly > > > polyies_
Definition: msg_traj.h:39
Definition: traj_data.h:61
double decimal_t
Definition: types.h:35
std::vector< std::vector< std::vector< boost::shared_ptr< Poly > > > > derrives_
Definition: msg_traj.h:40
Definition: msg_traj.h:27
TrajData serialize() override
Definition: msg_traj.cpp:119
TrajData traj_
Definition: msg_traj.h:38
bool evaluate(decimal_t t, uint derr, VecD &out) const override
Definition: msg_traj.cpp:67
uint deg_
Definition: msg_traj.h:43
decimal_t getCost() override
Definition: msg_traj.cpp:120
MsgTrajectory(const TrajData &traj)
Definition: msg_traj.cpp:24
decimal_t getTotalTime() const override
Definition: msg_traj.cpp:113
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49
std::vector< decimal_t > dts
Definition: msg_traj.h:42