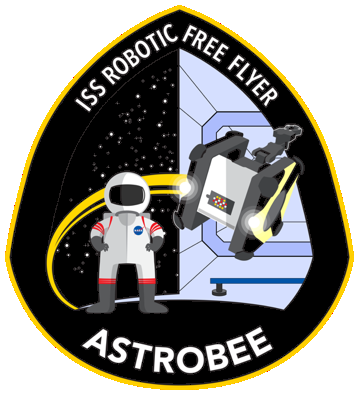 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef INTEREST_POINT_MATCHING_H_
19 #define INTEREST_POINT_MATCHING_H_
21 #include <boost/optional.hpp>
22 #include <opencv2/features2d/features2d.hpp>
34 double min_thresh,
double default_thresh,
double max_thresh,
35 double too_many_ratio,
double too_few_ratio);
37 void Detect(
const cv::Mat& image,
38 std::vector<cv::KeyPoint>* keypoints,
39 cv::Mat* keypoints_description);
41 std::vector<cv::KeyPoint>* keypoints) = 0;
43 std::vector<cv::KeyPoint>* keypoints,
44 cv::Mat* keypoints_description) = 0;
45 virtual void TooFew(
void) = 0;
47 void GetDetectorParams(
int& min_features,
int& max_features,
int& max_retries,
double& min_thresh,
48 double& default_thresh,
double& max_thresh,
double& too_many_ratio,
double& too_few_ratio);
61 std::string detector_name_;
69 FeatureDetector(std::string
const& detector_name =
"SURF",
int min_features = 0,
int max_features = 0,
70 int retries = 0,
double min_thresh = 0,
double default_thresh = 0,
double max_thresh = 0,
71 double too_many_ratio = 0,
double too_few_ratio = 0);
74 void Reset(std::string
const& detector_name,
int min_features = 0,
int max_features = 0,
int retries = 0,
75 double min_thresh = 0,
double default_thresh = 0,
double max_thresh = 0,
double too_many_ratio = 0,
76 double too_few_ratio = 0);
78 void Detect(
const cv::Mat& image, std::vector<cv::KeyPoint>* keypoints,
79 cv::Mat* keypoints_description);
83 void GetDetectorParams(
int& min_features,
int& max_features,
int& max_retries,
double& min_thresh,
84 double& default_thresh,
double& max_thresh,
double& too_many_ratio,
double& too_few_ratio);
87 return (A.detector_name_ == B.detector_name_);
100 void FindMatches(
const cv::Mat& img1_descriptor_map,
const cv::Mat& img2_descriptor_map,
101 std::vector<cv::DMatch>* matches, boost::optional<int> hamming_distance = boost::none,
102 boost::optional<double> goodness_ratio = boost::none);
105 #endif // INTEREST_POINT_MATCHING_H_
int max_features_
Definition: matching.h:53
void FindMatches(const cv::Mat &img1_descriptor_map, const cv::Mat &img2_descriptor_map, std::vector< cv::DMatch > *matches, boost::optional< int > hamming_distance=boost::none, boost::optional< double > goodness_ratio=boost::none)
Definition: matching.cc:358
double min_thresh_
Definition: matching.h:54
double default_thresh_
Definition: matching.h:54
int min_features_
Definition: matching.h:53
void Reset(std::string const &detector_name, int min_features=0, int max_features=0, int retries=0, double min_thresh=0, double default_thresh=0, double max_thresh=0, double too_many_ratio=0, double too_few_ratio=0)
Definition: matching.cc:288
int last_keypoint_count(void)
Definition: matching.h:50
Definition: matching.h:58
int last_keypoint_count_
Definition: matching.h:55
friend bool operator==(FeatureDetector const &A, FeatureDetector const &B)
Definition: matching.h:86
int max_retries_
Definition: matching.h:53
double too_many_ratio_
Definition: matching.h:54
void Detect(const cv::Mat &image, std::vector< cv::KeyPoint > *keypoints, cv::Mat *keypoints_description)
Definition: matching.cc:343
double max_thresh_
Definition: matching.h:54
virtual void TooMany(void)=0
void GetDetectorParams(int &min_features, int &max_features, int &max_retries, double &min_thresh, double &default_thresh, double &max_thresh, double &too_many_ratio, double &too_few_ratio)
Definition: matching.cc:96
virtual void ComputeImpl(const cv::Mat &image, std::vector< cv::KeyPoint > *keypoints, cv::Mat *keypoints_description)=0
~FeatureDetector(void)
Definition: matching.cc:281
double dynamic_thresh_
Definition: matching.h:54
virtual ~DynamicDetector(void)
Definition: matching.h:36
virtual void DetectImpl(const cv::Mat &image, std::vector< cv::KeyPoint > *keypoints)=0
void GetDetectorParams(int &min_features, int &max_features, int &max_retries, double &min_thresh, double &default_thresh, double &max_thresh, double &too_many_ratio, double &too_few_ratio)
Definition: matching.cc:272
DynamicDetector(int min_features, int max_features, int retries, double min_thresh, double default_thresh, double max_thresh, double too_many_ratio, double too_few_ratio)
Definition: matching.cc:83
double too_few_ratio_
Definition: matching.h:54
std::string GetDetectorName() const
Definition: matching.h:81
Definition: matching.h:31
virtual void TooFew(void)=0
void Detect(const cv::Mat &image, std::vector< cv::KeyPoint > *keypoints, cv::Mat *keypoints_description)
Definition: matching.cc:109
DynamicDetector & dynamic_detector()
Definition: matching.h:90