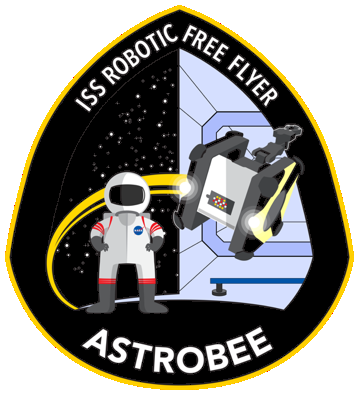 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef LOCALIZATION_SPARSE_MAPPING_TOOLS_UTILITIES_H_
19 #define LOCALIZATION_SPARSE_MAPPING_TOOLS_UTILITIES_H_
24 #include <boost/optional.hpp>
26 #include <Eigen/Geometry>
32 cv::Point2f
CvPoint2(
const Eigen::Vector2d& point);
34 void CreateSubdirectory(
const std::string& directory,
const std::string& subdirectory);
36 boost::optional<vision_common::FeatureMatches>
Matches(
44 cv::Feature2D& detector);
49 const double max_low_movement_mean_distance);
55 std::vector<std::string>
GetImageNames(
const std::string& image_directory,
const std::string& image_extension =
".jpg");
58 #endif // LOCALIZATION_SPARSE_MAPPING_TOOLS_UTILITIES_H_
Eigen::Affine3d EstimateAffine3d(const vc::FeatureMatches &matches, const camera::CameraParameters &camera_params, std::vector< cv::DMatch > &inliers)
Definition: utilities.cc:61
std::vector< FeatureMatch > FeatureMatches
Definition: feature_match.h:35
vc::FeatureImage LoadImage(const int index, const std::vector< std::string > &image_names, cv::Feature2D &detector)
Definition: utilities.cc:85
Definition: localization_parameters.h:22
Definition: camera_params.h:58
std::vector< std::string > GetImageNames(const std::string &image_directory, const std::string &image_extension)
Definition: utilities.cc:153
void CreateSubdirectory(const std::string &directory, const std::string &subdirectory)
Definition: utilities.cc:41
Definition: feature_image.h:27
boost::optional< vc::FeatureMatches > Matches(const vc::FeatureImage ¤t_image, const vc::FeatureImage &next_image, vc::LKOpticalFlowFeatureDetectorAndMatcher &detector_and_matcher)
Definition: utilities.cc:48
bool LowMovementImagePair(const vision_common::FeatureMatches &matches, const double max_low_movement_mean_distance)
Definition: utilities.cc:112
vc::LKOpticalFlowFeatureDetectorAndMatcherParams LoadParams()
Definition: utilities.cc:93
Definition: lk_optical_flow_feature_detector_and_matcher.h:26
Eigen::Affine3d Affine3d(const Eigen::Matrix< double, 7, 1 > &affine_vector)
Definition: utilities.cc:43
Definition: lk_optical_flow_feature_detector_and_matcher_params.h:24
cv::Point2f CvPoint2(const Eigen::Vector2d &point)
Definition: utilities.cc:39