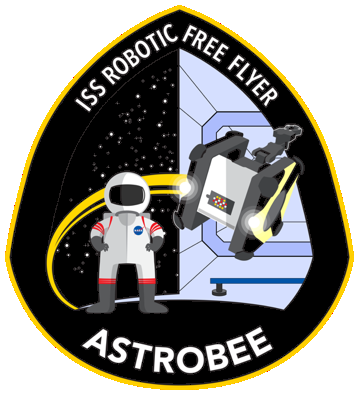 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_LINEAR_ALGEBRA_H_
20 #define MAPPER_LINEAR_ALGEBRA_H_
55 const double gain = 1.0 / (pow((x1 - x2).norm(), 2.0));
56 t_opt = gain*(x1 - x2).transpose()*(x1 - point);
61 *dist = (point - p_opt).norm();
76 std::cout <<
"Warning: plane ill-defined: "
77 <<
"zero-norm normal vector!"
89 if ((v1.norm() == 0) || (v2.norm() == 0) || ((p2-p3).norm() == 0)) {
90 std::cout <<
"Warning: plane ill-defined: "
91 <<
"three distinct points are needed!"
97 normal_ = v1.cross(v2).normalized();
106 transformedPlane->
normal_ = (transform.linear()*
normal_).normalized();
119 const double aspectRatio) {
121 double hh = tan(fov/2.0);
122 double hw = -hh*aspectRatio;
139 const uint32_t width,
140 const uint32_t height) {
142 double x0 = 0.0, y0 = 0.0;
143 double xf =
static_cast<float>(width) - 1;
144 double yf =
static_cast<float>(height) - 1;
145 double XL = -znear*(x0-cx)/fx;
146 double XR = -znear*(xf-cx)/fx;
147 double YU = -znear*(y0-cy)/fy;
148 double YD = -znear*(yf-cy)/fy;
169 transformed_frustum->
UL_ = transform*
UL_;
170 transformed_frustum->
UR_ = transform*
UR_;
171 transformed_frustum->
DL_ = transform*
DL_;
172 transformed_frustum->
DR_ = transform*
DR_;
190 visualization_msgs::Marker *line_list) {
192 line_list->header.frame_id = frame_id;
193 line_list->header.stamp = ros::Time::now();
194 line_list->ns =
"fustrum/" + frame_id;
195 line_list->action = visualization_msgs::Marker::ADD;
196 line_list->pose.orientation.w = 1.0;
197 line_list->type = visualization_msgs::Marker::LINE_LIST;
199 line_list->scale.x = 0.01;
201 line_list->lifetime = ros::Duration(1);
203 std::vector<Eigen::Vector3d> points;
205 points.push_back(
UL_);
206 points.push_back(
UR_);
207 points.push_back(
DL_);
208 points.push_back(
DR_);
210 geometry_msgs::Point node1, node2;
211 for (uint i = 0; i < points.size(); i++) {
212 for (uint j = 0; j < points.size(); j++) {
217 line_list->points.push_back(node1);
218 line_list->points.push_back(node2);
226 #endif // MAPPER_LINEAR_ALGEBRA_H_
static const Color Red()
Definition: visualization_functions.h:52
Eigen::Vector3d p0_
Definition: linear_algebra.h:33
Line3d(const Eigen::Vector3d &p1, const Eigen::Vector3d &p2)
Definition: linear_algebra.h:37
Definition: linear_algebra.h:110
Eigen::Vector3d UL_
Definition: linear_algebra.h:116
Eigen::Vector3d UR_
Definition: linear_algebra.h:116
Plane3d(const Eigen::Vector3d &origin, const Eigen::Vector3d &normal)
Definition: linear_algebra.h:71
Eigen::Vector3d DR_
Definition: linear_algebra.h:116
bool IsPointWithinFrustum(const Eigen::Vector3d &pt) const
Definition: linear_algebra.h:175
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
void VisualizeFrustum(const std::string &frame_id, visualization_msgs::Marker *line_list)
Definition: linear_algebra.h:189
FrustumPlanes(const double fx, const double fy, const double cx, const double cy, const uint32_t width, const uint32_t height)
Definition: linear_algebra.h:135
Definition: linear_algebra.h:27
Eigen::Vector3d origin_
Definition: linear_algebra.h:67
Plane3d down_plane_
Definition: linear_algebra.h:115
Definition: linear_algebra.h:65
Definition: linear_algebra.h:31
FrustumPlanes()
Definition: linear_algebra.h:160
Eigen::Vector3d origin_
Definition: linear_algebra.h:116
Plane3d up_plane_
Definition: linear_algebra.h:114
Plane3d right_plane_
Definition: linear_algebra.h:113
Eigen::Vector3d vec_
Definition: linear_algebra.h:34
Eigen::Affine3d Affine3d(const Eigen::Matrix< double, 7, 1 > &affine_vector)
Definition: utilities.cc:43
Plane3d()
Definition: linear_algebra.h:101
Plane3d(const Eigen::Vector3d &p1, const Eigen::Vector3d &p2, const Eigen::Vector3d &p3)
Definition: linear_algebra.h:83
Eigen::Vector3d normal_
Definition: linear_algebra.h:68
Eigen::Vector3d DL_
Definition: linear_algebra.h:116
void DistancePoint2Line(const Eigen::Vector3d &point, double *dist)
Definition: linear_algebra.h:44
FrustumPlanes(const double fov, const double aspectRatio)
Definition: linear_algebra.h:118
Plane3d left_plane_
Definition: linear_algebra.h:112
void transformPlane(const Eigen::Affine3d &transform, Plane3d *transformedPlane)
Definition: linear_algebra.h:103
geometry_msgs::Point eigen_to_ros_point(const Eigen::Vector3d &v)
Definition: msg_conversions.cc:37
void TransformFrustum(const Eigen::Affine3d &transform, FrustumPlanes *transformed_frustum)
Definition: linear_algebra.h:162