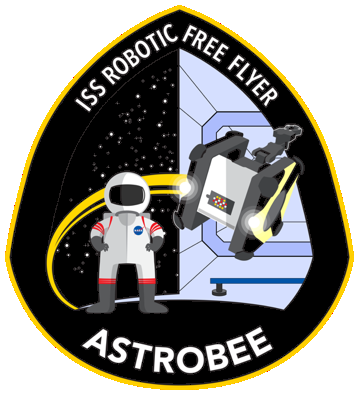 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
24 #ifndef MARKER_TRACKING_LABELLING_METHOD_H_
25 #define MARKER_TRACKING_LABELLING_METHOD_H_
34 #include <opencv2/core/core_c.h>
35 #include <ar_track_alvar/Alvar.h>
36 #include <ar_track_alvar/Util.h>
50 std::shared_ptr<cv::Mat>
bw_;
72 virtual void LabelSquares(std::shared_ptr<cv::Mat> image) = 0;
74 bool CheckBorder(std::vector<cv::Point> contour,
int width,
int height);
76 void SetThreshParams(
int param1,
int param2);
95 void LabelSquares(std::shared_ptr<cv::Mat> image);
100 #endif // MARKER_TRACKING_LABELLING_METHOD_H_
int min_edge_
Definition: labelling_method.h:88
camera::CameraParameters cam_
Definition: labelling_method.h:52
int min_area_
Definition: labelling_method.h:89
Definition: camera_params.h:58
Base class for labeling connected components from binary image.
Definition: labelling_method.h:45
int n_blobs_
Definition: labelling_method.h:87
EIGEN_MAKE_ALIGNED_OPERATOR_NEW
Definition: labelling_method.h:79
int thresh_param2_
Definition: labelling_method.h:53
Definition: arconfigio.h:27
std::vector< std::vector< alvar::PointDouble > > blob_corners
Vector of 4-length vectors where the corners of detected blobs are stored.
Definition: labelling_method.h:60
Labeling class that uses OpenCV routines to find connected components.
Definition: labelling_method.h:85
std::shared_ptr< cv::Mat > bw_
Pointer to binary image that is then labeled.
Definition: labelling_method.h:50