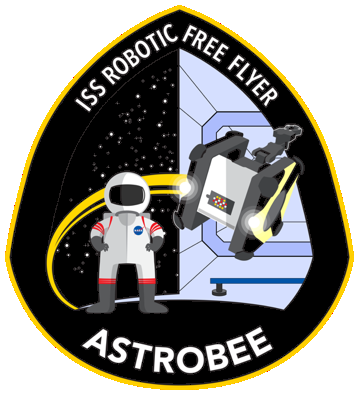 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_PRO_GUROBI_TRAJECTORY_H_
20 #define TRAJ_OPT_PRO_GUROBI_TRAJECTORY_H_
40 boost::shared_ptr<BasisBundlePro>
basis);
48 void getCost(GRBQuadExpr &cost);
67 boost::shared_ptr<BasisBundlePro>
basis;
86 boost::shared_ptr<BasisBundlePro>
basis_,
87 boost::shared_ptr<BasisTransformer> basisT_,
88 boost::shared_ptr<BasisTransformer> basisD_);
95 void getCost(GRBQuadExpr &cost);
103 std::vector<boost::shared_ptr<TrajSection1D>>
secs;
106 void getControl(uint point_id, uint
dim, GRBLinExpr &expr,
bool derr);
112 boost::shared_ptr<BasisTransformer>
basisT;
113 boost::shared_ptr<BasisTransformer>
basisD;
114 boost::shared_ptr<BasisBundlePro>
basis_;
149 const std::vector<decimal_t> &ds);
170 const MatD &Aenv,
const VecD &benv);
187 std::vector<decimal_t> dts;
188 std::vector<MatD> A_;
189 std::vector<VecD> b_;
190 std::vector<Waypoint> waypnts_;
192 std::vector<decimal_t> old_dts;
196 boost::shared_ptr<BasisTransformer> basisT;
197 boost::shared_ptr<BasisTransformer> basisD;
198 std::vector<GRBVar> slack_var;
200 std::vector<Poly> time_composite;
201 bool use_time_composite{
false};
207 #endif // TRAJ_OPT_PRO_GUROBI_TRAJECTORY_H_
uint8_t mode
Definition: signal_lights.h:74
uint k_r
Definition: gurobi_trajectory.h:64
std::vector< boost::shared_ptr< TrajSection1D > > secs
Definition: gurobi_trajectory.h:103
bool generated
Definition: gurobi_trajectory.h:75
void setConParam(ConstraintMode mode, int num)
Definition: gurobi_trajectory.h:172
void getContr(decimal_t x, uint derr, uint dim, GRBLinExpr &expr)
@ CHEBY
Definition: gurobi_trajectory.h:119
decimal_t evaluate(decimal_t t, uint derr) const
PolyType
Definition: traj_data.h:27
Eigen::Matrix< decimal_t, Eigen::Dynamic, Eigen::Dynamic > MatD
Definition: types.h:51
void addMaximumBound(decimal_t bound, uint derr)
Definition: trajectory.h:35
bool adjustTimes(decimal_t epsilon)
void getCost(GRBQuadExpr &cost)
boost::shared_ptr< BasisBundlePro > basis_
Definition: gurobi_trajectory.h:114
int dim
Definition: gurobi_trajectory.h:115
std::vector< GRBVar > const_var
Definition: gurobi_trajectory.h:109
void addConvexificationConstraints(const MatD &Aobs, const VecD &bobs, const MatD &Aenv, const VecD &benv)
void addPathCost(const MatD &A, const VecD &b, double epsilon)
Definition: traj_data.h:61
decimal_t dt_
Definition: gurobi_trajectory.h:117
void addLineCost(const Vec3 &p0, const Vec3 &p1, double upsilon)
boost::shared_ptr< BasisTransformer > basisT
Definition: gurobi_trajectory.h:112
std::vector< decimal_t > coeffs
Definition: gurobi_trajectory.h:72
bool recoverVars(decimal_t ratio=1.0)
decimal_t getCostV(const MatD &points, decimal_t weight)
void addWayPointConstrains(const std::vector< Waypoint > &waypnts, bool use_v0)
void resetConstrVision(const MatD &points, decimal_t weight)
ConstraintMode
Definition: gurobi_trajectory.h:119
double decimal_t
Definition: types.h:35
Definition: gurobi_trajectory.h:120
Eigen::Matrix< decimal_t, 3, 1 > Vec3
Definition: types.h:40
@ CONTROL
Definition: gurobi_trajectory.h:119
TrajSection4D(GRBModel *model, uint n_p_, uint k_r_, decimal_t dt_, boost::shared_ptr< BasisBundlePro > basis_, boost::shared_ptr< BasisTransformer > basisT_, boost::shared_ptr< BasisTransformer > basisD_)
void adjustTimeToMaxs(decimal_t max_vel, decimal_t max_acc, decimal_t max_jrk, bool use_v0, decimal_t v0)
bool evaluate(decimal_t t, uint derr, VecD &out) const
VecD check_max_violation(decimal_t max_vel, decimal_t max_acc, decimal_t max_jrk)
std::vector< GRBVar > coeffs_var
Definition: gurobi_trajectory.h:70
void resetConstr(bool lp=false)
std::vector< Mat4, Eigen::aligned_allocator< Mat4 > > Mat4Vec
Definition: types.h:47
void addVolumeContraints(const Mat4Vec &constr)
virtual TrajData serialize()
decimal_t dt
Definition: gurobi_trajectory.h:65
Definition: msg_traj.h:27
void setDt(decimal_t t)
Definition: gurobi_trajectory.h:55
bool check_max(decimal_t max_vel, decimal_t max_acc, decimal_t max_jrk)
std::vector< boost::shared_ptr< TrajSection4D > > individual_sections
Definition: gurobi_trajectory.h:168
GRBModel * model_
Definition: gurobi_trajectory.h:111
int n_p
Definition: gurobi_trajectory.h:116
void getCost(GRBQuadExpr &cost)
@ SAMPLE
Definition: gurobi_trajectory.h:119
void recoverVars(decimal_t ratio=1.0)
void getContr(decimal_t x, uint derr, GRBLinExpr &expr)
void recoverVarsF(decimal_t ratio=1.0)
@ TRAJ_PSI
Definition: gurobi_trajectory.h:35
void addVisionCost(const MatD &norm_pnts, decimal_t weight_loss, VecD &grad)
void recoverVarsF(decimal_t ratio=1.0)
TrajSection1D(GRBModel *model_, uint n_p_, uint k_r_, decimal_t dt_, boost::shared_ptr< BasisBundlePro > basis)
Definition: gurobi_trajectory.h:37
bool recoverVarsF(decimal_t ratio=1.0)
void getJacobian(const MatD &norm_pnts, decimal_t weight_loss, VecD &J)
void getControl(uint point_id, uint dim, GRBLinExpr &expr, bool derr)
uint n_p
Definition: gurobi_trajectory.h:63
void evaluate(decimal_t t, uint derr, VecD &out) const
decimal_t getTotalTime() const
@ TRAJ_Y
Definition: gurobi_trajectory.h:35
void getLinCost(GRBLinExpr &expr, uint i)
Definition: gurobi_trajectory.h:83
@ TRAJ_X
Definition: gurobi_trajectory.h:35
boost::math::tools::polynomial< decimal_t > Poly
Definition: polynomial_basis.h:61
LegendreTrajectory(GRBModel *model_, const std::vector< decimal_t > dts_, int degree, PolyType polytype, int order=3)
boost::shared_ptr< BasisBundlePro > basis
Definition: gurobi_trajectory.h:67
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49
boost::shared_ptr< BasisTransformer > basisD
Definition: gurobi_trajectory.h:113
void addAxbConstraints(const std::vector< MatD > &A, const std::vector< VecD > &b, const std::vector< decimal_t > &ds)
@ TRAJ_Z
Definition: gurobi_trajectory.h:35
void recoverVars(decimal_t ratio=1.0)
@ ELLIPSE
Definition: gurobi_trajectory.h:119
GRBModel * model
Definition: gurobi_trajectory.h:78