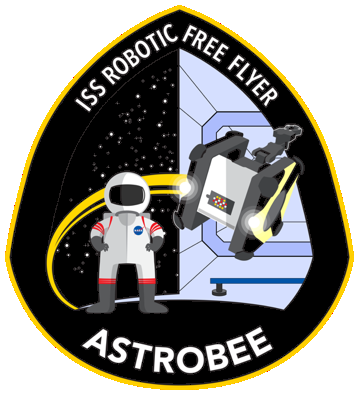 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef CAMERA_CAMERA_PARAMS_H_
20 #define CAMERA_CAMERA_PARAMS_H_
38 "camera. Use 0.923169 for ISS imagery, and 0.925417 for images "
39 "from the tango device.";
64 explicit CameraParameters(std::string
const& filename, std::string
const& base_dir =
"");
67 Eigen::Vector2d
const& focal_length,
68 Eigen::Vector2d
const& optical_center,
69 Eigen::VectorXd
const& distortion = Eigen::VectorXd());
77 template <
int SRC,
int DEST>
78 void Convert(Eigen::Vector2d
const& input, Eigen::Vector2d *output)
const {
79 throw(
"Please use the explicitly specified conversions by using the correct enum.");
85 throw(
"Please use the explicitly specified frame by using the correct enum.");
123 return (A.crop_offset_ == B.crop_offset_ &&
124 A.distorted_image_size_ == B.distorted_image_size_ &&
125 A.undistorted_image_size_ == B.undistorted_image_size_ &&
126 A.distorted_half_size_ == B.distorted_half_size_ &&
127 A.focal_length_ == B.focal_length_ &&
128 A.optical_offset_ == B.optical_offset_ &&
129 A.distortion_coeffs_ == B.distortion_coeffs_ &&
130 A.distortion_precalc1_ == B.distortion_precalc1_ &&
131 A.distortion_precalc2_ == B.distortion_precalc2_ &&
132 A.distortion_precalc3_ == B.distortion_precalc3_);
137 void DistortCentered(Eigen::Vector2d
const& undistorted_c,
138 Eigen::Vector2d* distorted_c)
const;
140 void UndistortCentered(Eigen::Vector2d
const& distorted_c,
141 Eigen::Vector2d* undistorted_c)
const;
146 distorted_image_size_,
147 undistorted_image_size_;
152 Eigen::Vector2d distorted_half_size_, undistorted_half_size_;
162 Eigen::VectorXd distortion_coeffs_;
163 double distortion_precalc1_, distortion_precalc2_, distortion_precalc3_;
166 #define DECLARE_CONVERSION(TYPEA, TYPEB) \
168 void CameraParameters::Convert<TYPEA, TYPEB>(Eigen::Vector2d const& input, Eigen::Vector2d *output) const
179 #undef DECLARE_CONVERSION
181 #define DECLARE_INTRINSIC(TYPE) \
183 Eigen::Matrix3d CameraParameters::GetIntrinsicMatrix<TYPE>() const
189 #undef DECLARE_INTRINSIC
192 #endif // CAMERA_CAMERA_PARAMS_H_
const Eigen::Vector2d & GetOpticalOffset() const
Definition: camera_params.cc:197
void Convert(Eigen::Vector2d const &input, Eigen::Vector2d *output) const
Definition: camera_params.h:78
void GenerateRemapMaps(cv::Mat *remap_map, double scale=1.0)
Definition: camera_params.cc:375
CameraParameters()=delete
@ UNDISTORTED
Definition: camera_params.h:54
friend bool operator==(CameraParameters const &A, CameraParameters const &B)
Definition: camera_params.h:122
void SetOpticalOffset(Eigen::Vector2d const &offset)
Definition: camera_params.cc:193
Definition: camera_model.h:26
DECLARE_CONVERSION(RAW, DISTORTED)
void SetDistortedSize(Eigen::Vector2i const &image_size)
Definition: camera_params.cc:159
Definition: camera_params.h:58
const Eigen::Vector2d & GetFocalVector() const
Definition: camera_params.cc:209
const Eigen::VectorXd & GetDistortion() const
Definition: camera_params.cc:245
const Eigen::Vector2i & GetDistortedSize() const
Definition: camera_params.cc:164
const Eigen::Vector2i & GetCropOffset() const
Definition: camera_params.cc:189
Eigen::Matrix3d GetIntrinsicMatrix() const
Definition: camera_params.h:84
std::string name
Definition: eps_simulator.cc:48
void SetUndistortedSize(Eigen::Vector2i const &image_size)
Definition: camera_params.cc:172
@ DISTORTED
Definition: camera_params.h:52
void SetFocalLength(Eigen::Vector2d const &)
Definition: camera_params.cc:201
const Eigen::Vector2d & GetDistortedHalfSize() const
Definition: camera_params.cc:168
double GetFocalLength() const
Definition: camera_params.cc:205
Definition: config_reader.h:48
const Eigen::Vector2d & GetUndistortedHalfSize() const
Definition: camera_params.cc:181
const char distortion_msg[]
Definition: camera_params.h:37
const Eigen::Vector2i & GetUndistortedSize() const
Definition: camera_params.cc:177
EIGEN_MAKE_ALIGNED_OPERATOR_NEW
Definition: camera_params.h:60
@ UNDISTORTED_C
Definition: camera_params.h:55
Definition: camera_params.h:31
@ DISTORTED_C
Definition: camera_params.h:53
@ RAW
Definition: camera_params.h:51
void SetCropOffset(Eigen::Vector2i const &crop)
Definition: camera_params.cc:185
void SetDistortion(Eigen::VectorXd const &distortion)
Definition: camera_params.cc:213