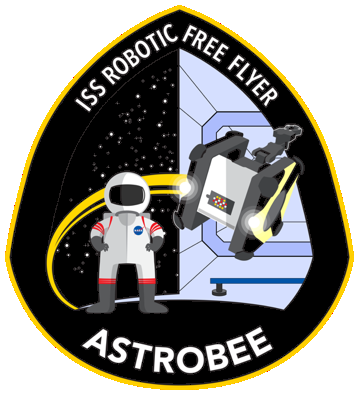 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef COMMS_BRIDGE_BRIDGE_SUBSCRIBER_H_
20 #define COMMS_BRIDGE_BRIDGE_SUBSCRIBER_H_
30 #include <topic_tools/shape_shifter.h>
50 bool addTopic(std::string
const& in_topic, std::string
const& out_topic);
129 #endif // COMMS_BRIDGE_BRIDGE_SUBSCRIBER_H_
std::shared_ptr< ros::Subscriber > SubscriberPtr
Definition: bridge_publisher.h:43
std::string definition
Definition: bridge_subscriber.h:68
BridgeSubscriber()
Definition: bridge_subscriber.cpp:34
virtual void advertiseTopic(const RelayTopicInfo &info)=0
Definition: bridge_subscriber.h:71
virtual void relayMessage(const RelayTopicInfo &topic_info, ContentInfo const &content_info)=0
void handleRelayedMessage(const ros::MessageEvent< topic_tools::ShapeShifter const > &msg_event, std::string const &topic, SubscriberPtr sub)
Definition: bridge_subscriber.cpp:45
Definition: bridge_subscriber.h:56
AdvertisementInfo ad_info
Definition: bridge_subscriber.h:81
unsigned int relay_seqnum
Definition: bridge_subscriber.h:78
bool addTopic(std::string const &in_topic, std::string const &out_topic)
Definition: bridge_subscriber.cpp:152
std::shared_ptr< ros::Subscriber > SubscriberPtr
Definition: bridge_subscriber.h:37
std::map< std::string, RelayTopicInfo > m_relay_topics_
Definition: bridge_subscriber.h:124
Definition: bridge_subscriber.h:84
virtual ~BridgeSubscriber()
Definition: bridge_subscriber.cpp:41
size_t data_size
Definition: bridge_subscriber.h:90
void setVerbosity(unsigned int verbosity)
Definition: bridge_subscriber.cpp:43
std::mutex m_mutex_
Definition: bridge_subscriber.h:122
bool advertised
Definition: bridge_subscriber.h:77
SubscriberPtr sub
Definition: bridge_subscriber.h:75
std::string data_type
Definition: bridge_subscriber.h:62
std::string md5_sum
Definition: bridge_subscriber.h:65
RelayTopicInfo()
Definition: bridge_subscriber.h:73
uint8_t * m_msgbuffer_
Definition: bridge_subscriber.h:123
std::string out_topic
Definition: bridge_subscriber.h:76
std::shared_ptr< ros::Publisher > PublisherPtr
Definition: bridge_subscriber.h:38
unsigned int m_verbose_
Definition: bridge_subscriber.h:120
unsigned int m_n_relayed_
Definition: bridge_subscriber.h:126
bool latching
Definition: bridge_subscriber.h:59
const uint8_t * data
Definition: bridge_subscriber.h:93
SubscriberPtr rosSubscribe(std::string const &topic)
Definition: bridge_subscriber.cpp:131
Definition: bridge_subscriber.h:40
std::string type_md5_sum
Definition: bridge_subscriber.h:87
virtual void subscribeTopic(std::string const &in_topic, const RelayTopicInfo &info)=0