fmdtools.define.block
The block subpackage provides a representation of blocks, which are simulable models which may contain containers and other properties.
These variants of block are provided in the following modules:
fmdtools.define.block.base
: forSimulable
, which is used for all simulations (including architectures) andBlock
, which is used for blocks.fmdtools.define.block.action
: forAction
, which is used to represent actions.fmdtools.define.block.component
: forComponent
, which is used to represent components.fmdtools.define.block.function
: forFunction
, which is used to represent functions.
fmdtools.define.block.base
Description: A module to define blocks.
Simulable
: Superclass for architectures and blocks.Block
: Superclass for Functions, Components, Actions, etc.
- class fmdtools.define.block.base.Block(name='', flows={}, h={}, **kwargs)
Bases:
Simulable
Superclass for Function, Component, and Action subclasses.
Has functions for model setup, querying state, and reseting the model.
- name
Block name
- Type:
str
- flows
Dictionary of flows included in the Block (if any are added via flow_flowname)
- Type:
dict
- check_dict_creation = True
- check_flows(flows={})
Associate flows with the given Simulable.
Flows must be defined with the flow_ class variable pointing to the class to initialize (e.g., flow_flowname = FlowClass).
- Parameters:
flows (dict, optional) – If flows is provided AND it contains a flowname corresponding to the function’s flowname, it will be used instead (so that it can act as a connection to the rest of the model)
- choose_rand_fault(faults, default='first', combinations=1)
Randomly chooses a fault or combination of faults to insert in fxn.m.
- Parameters:
faults (list) – list of fault modes to choose from
default (str/list, optional) – Default fault to inject when model is run deterministically. The default is ‘first’, which chooses the first in the list. Can provide a mode as a str or a list of modes
combinations (int, optional) – Number of combinations of faults to elaborate and select from. The default is 1, which just chooses single fault modes.
- copy(*args, flows={}, **kwargs)
Copy the block with its current attributes.
- Parameters:
args (tuple) – New arguments to use to instantiate the block, (e.g., flows, p, s)
kwargs – New kwargs to use to instantiate the block.
- Returns:
cop – copy of the exising block
- Return type:
- create_arch_kwargs(**kwargs)
Create keyword arguments for contained architectures.
Enables the passing of flows from block to contained architecture level.
- default_track = ['s', 'm', 'r', 't', 'i']
- get_flows()
Return a dictionary of the Block’s flows.
- get_flowtypes()
Return the names of the flow types in the model.
- Returns:
flowtypes – Set of flow type names in the model.
- Return type:
set
- get_rand_states(auto_update_only=False)
Get dict of random states from block and associated actions/components.
- Parameters:
auto_update_only –
- Returns:
rand_states – Random states from the block and associated actions/components.
- Return type:
dict
- get_typename()
Get the name of the type (Block for Blocks).
- Returns:
typename – Block
- Return type:
str
- init_block(**kwargs)
Placeholder initialization method to set initial states etc.
- is_dynamic()
Check if Block has dynamic execution step.
- is_static()
Check if Block has static execution step.
- m
- propagate(time, faults={}, disturbances={}, run_stochastic=False)
Inject and propagates faults through the graph at one time-step.
- Parameters:
time (float) – The current time-step.
faults (dict) – Faults to inject during this propagation step. With structure {fname: [‘fault1’, ‘fault2’…]}
disturbances (dict) – Variables to change during this propagation step. With structure {‘var1’: value}
run_stochastic (bool) – Whether to run stochastic behaviors or use default values. Default is False. Can set as ‘track_pdf’ to calculate/track the probability densities of random states over time.
- roletypes = ['container', 'flow']
- s
- update_contained_modes()
Add contained faultmodes for the container at to the Function model.
- Parameters:
at (str ('ca' or 'aa')) – Role to update (for ComponentArchitecture or ActionArchitecture roles)
- class fmdtools.define.block.base.SimParam(*args, **kwargs)
Bases:
Parameter
Class defining Simulation parameters.
- Has fields:
- phasestuple
phases ((‘name’, start, end)…) that the simulation progresses through
- start_timefloat
Start time of the simulation.
- end_timefloat
End time of the simulation.
- track_timestuple
Defines what times to include in the history. Options are: - (‘all’,)–all simulated times - (‘interval’, n)–includes every nth time in the history - (‘times’, [t1, … tn])–only includes times defined in the
vector [t1 … tn]
- dtfloat
time-step used in the simulation. default is 1.0
- unitsstr
time-units. default is hours`
- end_conditionstr
Name of indicator method to use to end the simulation. If not provided (‘’), the simulation ends at the final time. Default is ‘’
- use_localbool
Whether to use locally-defined time-steps in functions (if any). Default is True.
- dt: float
- end_condition: str
- end_time: float
- find_any_phase_overlap()
Check that simparam phases don’t overlap.
- get_hist_ind(t_ind, t, shift)
Get the index of the history given the simulation time/index and shift.
Examples
>>> SimParam().get_hist_ind(2, 2.0, 1) 3 >>> SimParam(track_times=('interval', 2)).get_hist_ind(4, 4.0, 0) 2
- get_histrange(start_time=0.0, end_time=None)
Get the history range associated with the SimParam.
- get_shift(old_start_time=0.0)
Get the shift between the sim timerange in the history.
Examples
>>> SimParam().get_shift(6.0) 6 >>> SimParam(track_times=('interval', 2)).get_shift(2) 1
- get_timerange(start_time=None, end_time=None)
Generate the timerange to simulate over.
Examples
>>> SimParam(end_time=5.0).get_timerange() array([0., 1., 2., 3., 4., 5.])
- phases: tuple
- rolename = 'sp'
- start_time: float
- track_times: tuple
- units: str
- units_set = ('sec', 'min', 'hr', 'day', 'wk', 'month', 'year')
- use_local: bool
- class fmdtools.define.block.base.Simulable(sp={}, **kwargs)
Bases:
BaseObject
Base class for object which simulate (blocks and architectures).
Note that classes solely based on Simulable may not be able to be simulated.
…
- Parameters:
- default_sp = {}
- default_track = ['all']
- find_classification(scen, mdlhists)
Classify the results of the simulation (placeholder).
- find_mutables()
Return list of mutable roles.
- flows
- get_fxns()
Get fxns associated with the Simulable (self if Function, self.fxns if Model).
- Returns:
fxns – Dict with structure {fxnname: fxnobj}
- Return type:
dict
- get_scen_rate(fxnname, faultmode, time, phasemap={}, weight=1.0)
Get the scenario rate for the given single-fault scenario.
- Parameters:
fxnname (str) – Name of the function with the fault
faultmode (str) – Name of the fault mode
time (int) – Time when the scenario is to occur
phasemap (PhaseMap, optional) – Map of phases/modephases that define operations the mode will be injected during (and maps to the opportunity vector phases). The default is {}.
weight (int, optional) – Scenario weight (e.g., if more than one scenario is sampled for the fault). The default is 1.
- Returns:
rate – Rate of the scenario
- Return type:
float
- get_vars(*variables, trunc_tuple=True)
Get variable values in the simulable.
- Parameters:
*variables (list/string) – Variables to get from the model. Can be specified as a list [‘fxnname2’, ‘comp1’, ‘att2’], or a str ‘fxnname.comp1.att2’
- Returns:
variable_values – Values of variables. Passes (non-tuple) single value if only one variable.
- Return type:
tuple
- h
- immutable_roles = ['p', 'sp']
- init_hist(h={})
Initialize the history of the sim using SimParam parameters and track.
- init_time_hist()
Add time history to the model (only done at top level).
- log_hist(t_ind, t, shift)
Log the history over time.
- new(**kwargs)
Create a new Model with the same parameters as the current model.
Can initiate with with changes to mutable parameters (p, sp, track, rand etc.).
- new_params(name='', p={}, sp={}, r={}, track={}, **kwargs)
Create a copy of the defining immutable parameters for use in a new Simulable.
- Parameters:
p (dict) – Parameter args to update
sp (dict) – SimParam args to update
r (dict) – Rand args to update
track (dict) – track kwargs to update.
- Returns:
param_dict – Dict with immutable parameters/options. (e.g., ‘p’, ‘sp’, ‘track’)
- Return type:
dict
- p
- r
- return_mutables()
Return all mutable values in the block.
Used in static propagation steps to check if the block has changed.
- Returns:
states – tuple of all states in the block
- Return type:
tuple
- return_probdens()
Get the probability density associated with Block and things it contains.
- sp
- t
- track
- update_seed(seed=[])
Update seed and propogates update to contained actions/components.
(keeps seeds in sync)
- Parameters:
seed (int, optional) – Random seed. The default is [].
fmdtools.define.block.action
Description: A module to define Actions.
Action
: Class for defining Actions.
- class fmdtools.define.block.action.Action(name=None, duration=0.0, **kwargs)
Bases:
Block
Superclass for actions.
Actions are blocks which have behaviors and live in an ActionArchitecture.
- behavior(time)
Simulate action behavior (placeholder for user-defined method).
- copy(*args, **kwargs)
Copy the block with its current attributes.
- Parameters:
args (tuple) – New arguments to use to instantiate the block, (e.g., flows, p, s)
kwargs – New kwargs to use to instantiate the block.
- Returns:
cop – copy of the exising block
- Return type:
- duration
fmdtools.define.block.component
Description: A module to define Components.
- class fmdtools.define.block.component.Component(name='', flows={}, h={}, **kwargs)
Bases:
Block
Superclass for components (most attributes inherited from Block superclass).
- behavior(time)
Simulate behavior provided (placeholder).
Enables one to include components without yet having a defined behavior for them.
- get_typename()
Get the name of the type (Block for Blocks).
- Returns:
typename – Block
- Return type:
str
fmdtools.define.block.function
Description: A module to define Functions.
Function
: Class for defining model Functions.
Functions are used to represent overall system functionality and behaviors (i.e., what a system does).
Functions are defined by extending the Function class, which may then be instantiated, as shown below:
Example of a Function class and its corresponding instantiation.
To define a function class, it can be helpful to use this code template:
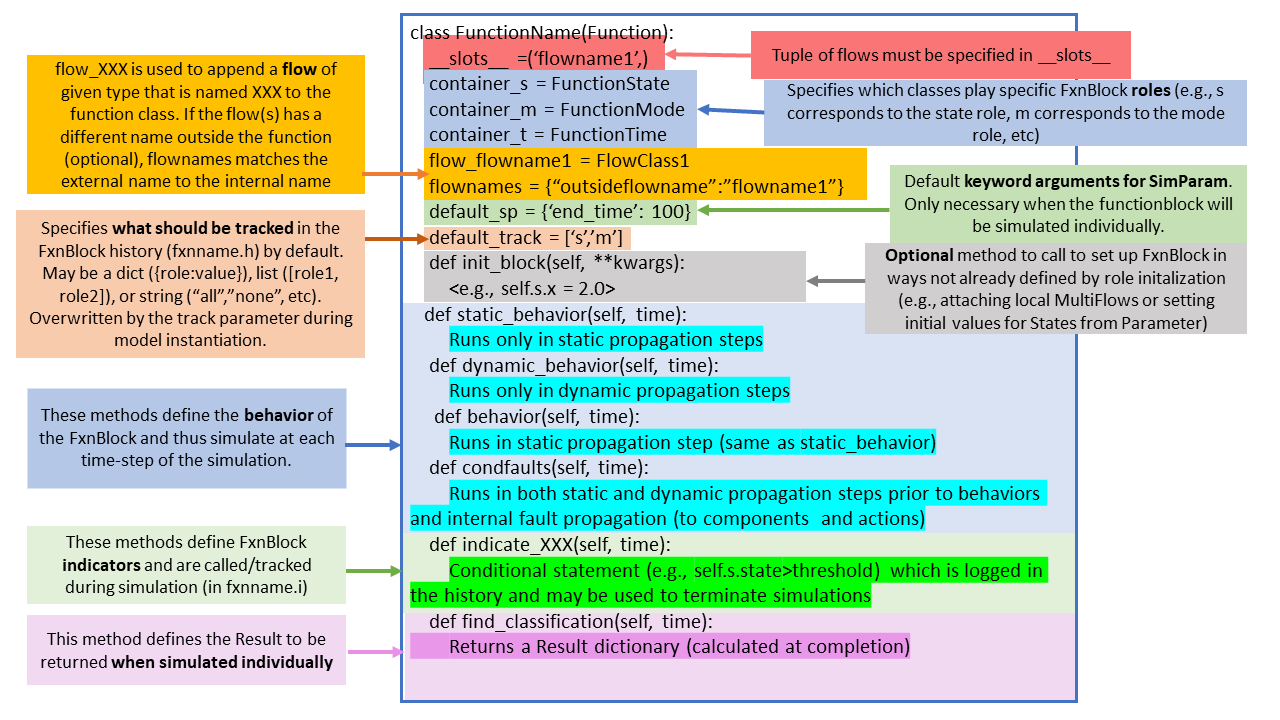
Code template for Function
used to define high-level system functions and their behavior.
- class fmdtools.define.block.function.Function(name=None, args_f={}, **kwargs)
Bases:
Block
Superclass for representing system functions.
Functions are distinguished from other blocks in their ability to contain architectures, which may be used to hold multiple components or an action sequence graph within the function.
- archArchitecture
component, action, function architectures at ca, aa, fa, etc.
Examples
>>> exf = ExampleFunction("exf") >>> exf exf ExampleFunction - ExampleState(x=1.0, y=1.0) - ExampleMode(mode=standby, faults=set())
Behavior can be called using __call__ or the user-defined behavior method:
>>> exf("dynamic", time=1.0) >>> exf exf ExampleFunction - ExampleState(x=2.0, y=1.0) - ExampleMode(mode=standby, faults=set())
Which can also be used to inject faults:
>>> exf("dynamic", time=2.0, faults=['no_charge']) >>> exf exf ExampleFunction - ExampleState(x=2.0, y=4.0) - ExampleMode(mode=no_charge, faults={'no_charge'})
- aa
- archs
- args_f
- ca
- default_track = ['ca', 'aa', 'fa', 's', 'm', 'r', 't', 'i']
- fa
- get_typename()
Get the name of the type (Block for Blocks).
- Returns:
typename – Block
- Return type:
str
- prop_arch_behaviors(proptype, faults, time, run_stochastic)
Propagate behaviors into contained architectures.
- prop_arch_faults_up()
Get faults from contained components and add to .m.
- return_faultmodes()
Get the fault modes present in the simulation (for propagate/model).
- Returns:
ms (list) – List of faults present.
modeprops (dict) – Dict of corresponding fault mode properties.
- return_probdens()
Get the probability density associated with FxnBlock and its archs.
- roletypes = ['container', 'flow', 'arch']
- update_seed(seed=[])
Update seed and propogates update to contained actions/components.
(keeps seeds in sync)
- Parameters:
seed (int, optional) – Random seed. The default is [].