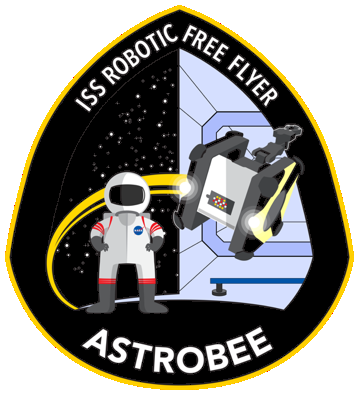 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
55 #ifndef SPARSE_MAPPING_VOCAB_TREE_H_
56 #define SPARSE_MAPPING_VOCAB_TREE_H_
58 #include <google/protobuf/io/zero_copy_stream_impl.h>
83 void SaveProtobuf(google::protobuf::io::ZeroCopyOutputStream* output)
const;
84 void LoadProtobuf(google::protobuf::io::ZeroCopyInputStream* input,
int db_type);
88 std::string
const& descriptor);
90 void BuildDB(std::string
const& map_file,
91 std::string
const& descriptor,
92 int depth,
int branching_factor,
int restarts);
97 void QueryDB(std::string
const& descriptor,
VocabDB* vocab_db,
int num_similar, cv::Mat
const& descriptors,
98 std::vector<int>* indices, std::vector<double>* scores);
101 std::string
const& descriptor,
102 int depth,
int branching_factor,
int restarts);
105 #endif // SPARSE_MAPPING_VOCAB_TREE_H_
~VocabDB()
Definition: vocab_tree.cc:290
void BuildDB(std::string const &map_file, std::string const &descriptor, int depth, int branching_factor, int restarts)
Definition: vocab_tree.cc:312
void BuildDBforDBoW2(sparse_mapping::SparseMap *map, std::string const &descriptor, int depth, int branching_factor, int restarts)
Definition: vocab_tree.cc:412
void LoadProtobuf(google::protobuf::io::ZeroCopyInputStream *input, int db_type)
Definition: vocab_tree.cc:302
void DBSanityChecks(std::string const &db_type, std::string const &descriptor)
Definition: localization_parameters.h:22
void ResetDB(VocabDB *db)
Definition: vocab_tree.cc:335
VocabDB()
Definition: vocab_tree.cc:287
void SaveProtobuf(google::protobuf::io::ZeroCopyOutputStream *output) const
Definition: vocab_tree.cc:294
BinaryDB * binary_db
Definition: vocab_tree.h:78
Definition: vocab_tree.h:74
void QueryDB(std::string const &descriptor, VocabDB *vocab_db, int num_similar, cv::Mat const &descriptors, std::vector< int > *indices, std::vector< double > *scores)
Definition: vocab_tree.cc:380
int m_num_nodes
Definition: vocab_tree.h:80
Definition: vocab_tree.cc:105
Definition: camera_params.h:31
Definition: sparse_map.h:63