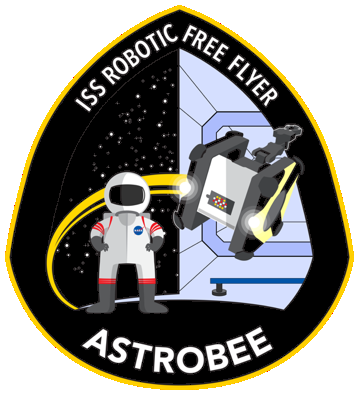 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef CHOREOGRAPHER_VALIDATOR_H_
20 #define CHOREOGRAPHER_VALIDATOR_H_
26 #include <visualization_msgs/MarkerArray.h>
34 #include <ff_msgs/Zone.h>
35 #include <ff_msgs/SetZones.h>
36 #include <ff_msgs/GetZones.h>
37 #include <ff_msgs/GetOccupancyMap.h>
38 #include <ff_msgs/GetFloat.h>
41 #include <jps3d/planner/jps_3d_util.h>
74 ff_msgs::FlightMode
const& flight_mode,
bool face_forward);
81 void ProcessZone(std::vector<signed char>& map,
int type,
char cell_value,
bool surface);
88 ff_msgs::GetZones::Response& res);
92 ff_msgs::GetOccupancyMap::Response& res);
96 ff_msgs::SetZones::Response& res);
99 std::string zone_file_;
101 ff_msgs::SetZones::Request zones_;
102 ros::Publisher pub_zones_;
103 ros::ServiceServer get_zones_srv_;
104 ros::ServiceServer get_zones_map_srv_;
105 ros::ServiceServer set_zones_srv_;
106 ros::ServiceClient get_resolution_;
107 ros::ServiceClient get_map_inflation_;
109 double map_res_ = 0.08;
110 std::shared_ptr<JPS::VoxelMapUtil> jps_map_util_;
115 char val_unknown_ = -1;
120 #endif // CHOREOGRAPHER_VALIDATOR_H_
@ VIOLATES_HARD_LIMIT_ACCEL
Definition: validator.h:64
Response
Definition: validator.h:54
Definition: validator.h:51
bool GetZonesMap()
Definition: validator.cc:81
@ SUCCESS
Definition: validator.h:55
@ VIOLATES_STATIONARY_ENDPOINT
Definition: validator.h:60
@ VIOLATES_FIRST_IN_PAST
Definition: validator.h:61
@ VIOLATES_HARD_LIMIT_ALPHA
Definition: validator.h:66
Definition: config_server.h:45
std::vector< Setpoint > Segment
Definition: ff_flight.h:47
void ProcessZone(std::vector< signed char > &map, int type, char cell_value, bool surface)
Definition: validator.cc:27
bool Init(ros::NodeHandle *nh, ff_util::ConfigServer &cfg)
Definition: validator.cc:216
void PublishMarkers()
Definition: validator.cc:256
@ VIOLATES_MINIMUM_FREQUENCY
Definition: validator.h:59
Response CheckSegment(ff_util::Segment const &msg, ff_msgs::FlightMode const &flight_mode, bool face_forward)
Definition: validator.cc:161
@ VIOLATES_MINIMUM_SETPOINTS
Definition: validator.h:62
@ VIOLATES_HARD_LIMIT_VEL
Definition: validator.h:63
bool SetZonesCallback(ff_msgs::SetZones::Request &req, ff_msgs::SetZones::Response &res)
Definition: validator.cc:340
bool GetZonesMapCallback(ff_msgs::GetOccupancyMap::Request &req, ff_msgs::GetOccupancyMap::Response &res)
Definition: validator.cc:318
@ VIOLATES_KEEP_IN
Definition: validator.h:58
Definition: validator.h:49
@ VIOLATES_HARD_LIMIT_OMEGA
Definition: validator.h:65
bool GetZonesCallback(ff_msgs::GetZones::Request &req, ff_msgs::GetZones::Response &res)
Definition: validator.cc:310
@ VIOLATES_KEEP_OUT
Definition: validator.h:57
@ VIOLATES_RESAMPLING
Definition: validator.h:56