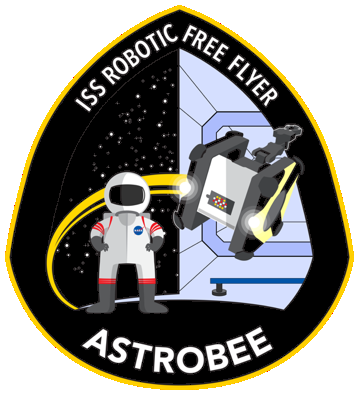 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MOBILITY_PLANNER_QP_TRAJ_OPT_ROS_SRC_TRAJECTORY_VISUAL_H_
20 #define MOBILITY_PLANNER_QP_TRAJ_OPT_ROS_SRC_TRAJECTORY_VISUAL_H_
24 #include <traj_opt_msgs/Trajectory.h>
58 Ogre::SceneNode* parent_node);
65 void setMessage(
const traj_opt_msgs::Trajectory::ConstPtr& msg);
77 void setColor(
float r,
float g,
float b,
float a);
78 void setColorV(
float r,
float g,
float b,
float a);
79 void setColorA(
float r,
float g,
float b,
float a);
91 std::vector<boost::shared_ptr<rviz::Object> > vel_arrows_;
92 std::vector<boost::shared_ptr<rviz::Object> > acc_arrows_;
94 std::vector<boost::shared_ptr<rviz::Object> > trajectory_lines_;
95 std::vector<boost::shared_ptr<rviz::Shape> > trajectory_balls_;
97 double thickness_{0.1};
98 int num_traj_points_{50};
99 int num_vel_points_{50};
106 static void setShapeFromPosePair(
const Ogre::Vector3& p0,
107 const Ogre::Vector3& p1,
double scale,
109 static void setShapeFromPosePair(
const Ogre::Vector3& p0,
110 const Ogre::Vector3& p1,
double scale,
113 boost::shared_ptr<traj_opt::Trajectory> traj_;
117 Ogre::SceneNode* frame_node_;
121 Ogre::SceneManager* scene_manager_;
127 #endif // MOBILITY_PLANNER_QP_TRAJ_OPT_ROS_SRC_TRAJECTORY_VISUAL_H_
@ SE3
Definition: trajectory_visual.h:51
@ CJ
Definition: trajectory_visual.h:51
Definition: trajectory_display.h:28
Definition: trajectory_visual.h:53
void draw()
Definition: trajectory_visual.cpp:95
void setStyle(int style)
Definition: trajectory_visual.cpp:78
TrajectoryVisual(Ogre::SceneManager *scene_manager, Ogre::SceneNode *parent_node)
Definition: trajectory_visual.cpp:51
void setCurve()
Definition: trajectory_visual.cpp:157
void resetTrajPoints(int traj_points, int tangent_points, bool use_v, bool use_a)
Definition: trajectory_visual.cpp:87
void setFramePosition(const Ogre::Vector3 &position)
Definition: trajectory_visual.cpp:304
void setColorA(float r, float g, float b, float a)
Definition: trajectory_visual.cpp:322
@ Sikang
Definition: trajectory_visual.h:51
void setColorV(float r, float g, float b, float a)
Definition: trajectory_visual.cpp:319
void setMessage(const traj_opt_msgs::Trajectory::ConstPtr &msg)
Definition: trajectory_visual.cpp:296
Style
Definition: trajectory_visual.h:51
Definition: trajectory_display.h:32
void setScale(float thickness)
Definition: trajectory_visual.cpp:325
virtual ~TrajectoryVisual()
Definition: trajectory_visual.cpp:74
Definition: msg_traj.h:27
void setColor(float r, float g, float b, float a)
Definition: trajectory_visual.cpp:314
@ Mike
Definition: trajectory_visual.h:51
void setFrameOrientation(const Ogre::Quaternion &orientation)
Definition: trajectory_visual.cpp:308
Eigen::Matrix< decimal_t, 3, 3 > Mat3
Definition: types.h:42
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49