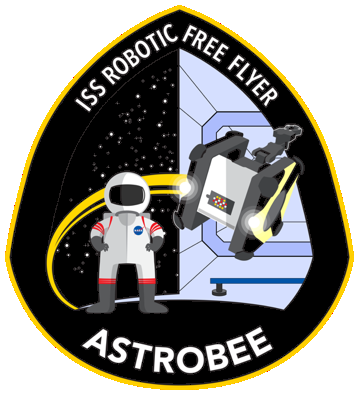 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef TRAJ_OPT_BASIC_TRAJECTORY_H_
20 #define TRAJ_OPT_BASIC_TRAJECTORY_H_
27 #include <boost/smart_ptr/shared_ptr.hpp>
58 #endif // TRAJ_OPT_BASIC_TRAJECTORY_H_
virtual decimal_t getCost()=0
int dim_
Definition: trajectory.h:55
Eigen::Matrix< decimal_t, Eigen::Dynamic, Eigen::Dynamic > MatD
Definition: types.h:51
Definition: trajectory.h:35
bool getCommand(decimal_t t, uint num_derivatives, MatD &data)
Definition: trajectory.cpp:31
Definition: traj_data.h:61
void setExecuteTime(decimal_t t)
Definition: trajectory.h:49
double decimal_t
Definition: types.h:35
decimal_t exec_t
Definition: trajectory.h:54
Definition: msg_traj.h:27
void setDim(uint ndim)
Definition: trajectory.h:48
virtual bool evaluate(decimal_t t, uint derr, VecD &out) const =0
virtual TrajData serialize()=0
virtual decimal_t getTotalTime() const =0
decimal_t getExecuteTime() const
Definition: trajectory.cpp:24
Eigen::Matrix< decimal_t, Eigen::Dynamic, 1 > VecD
Definition: types.h:49
virtual ~Trajectory()
Definition: trajectory.h:37