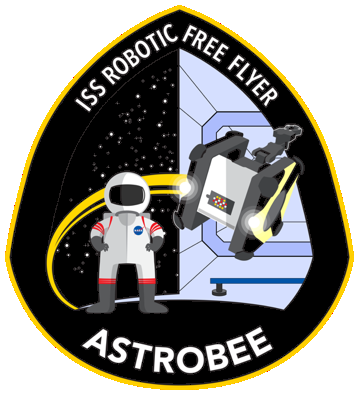 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef LOCALIZATION_ANALYSIS_UTILITIES_H_
19 #define LOCALIZATION_ANALYSIS_UTILITIES_H_
27 #include <opencv2/core/mat.hpp>
29 #include <rosbag/bag.h>
30 #include <sensor_msgs/Image.h>
38 template <
typename MsgType>
39 void SaveMsg(
const MsgType& msg,
const std::string& topic, rosbag::Bag& bag) {
41 bag.write(
"/" + topic, timestamp, msg);
53 #endif // LOCALIZATION_ANALYSIS_UTILITIES_H_
Definition: ar_tag_pose_adder.h:29
Eigen::Isometry3d Isometry3d(const cv::Mat &rodrigues_rotation_cv, const cv::Mat &translation_cv)
Definition: utilities.cc:58
bool string_ends_with(const std::string &str, const std::string &ending)
Definition: utilities.cc:117
void SaveMsg(const MsgType &msg, const std::string &topic, rosbag::Bag &bag)
Definition: utilities.h:39
geometry_msgs::PoseStamped PoseMsg(const Eigen::Isometry3d &global_T_body, const std_msgs::Header &header)
Definition: utilities.cc:125
Definition: timestamped_pose.h:27
ros::Time RosTimeFromHeader(const std_msgs::Header &header)
Definition: utilities.cc:107
double Time
Definition: time.h:23