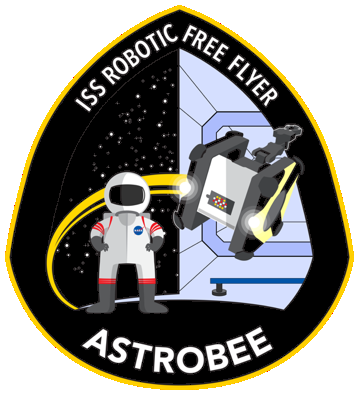 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef SPEED_CAM_SPEED_CAM_H_
20 #define SPEED_CAM_SPEED_CAM_H_
26 #include <mavlink/v1.0/common/mavlink.h>
34 #define MILLIMSS_TO_MSS (0.0010)
35 #define MILLIRADS_TO_RADS (0.0010)
99 size_t image_packets_;
100 int32_t image_payload_;
101 int32_t image_width_;
102 int32_t image_height_;
103 std::vector<uint8_t> image_buffer_;
104 uint32_t sw_version_;
109 #endif // SPEED_CAM_SPEED_CAM_H_
@ STATUS_NORMAL
Definition: speed_cam.h:60
std::function< void(int32_t)> SpeedCamStateCallback
Definition: speed_cam.h:49
SpeedCamResult
Definition: speed_cam.h:52
std::function< void(std::vector< uint8_t > const &, int32_t, int32_t)> SpeedCamCameraImageCallback
Definition: speed_cam.h:44
@ RESULT_PORT_NOT_OPEN
Definition: speed_cam.h:54
std::function< void(mavlink_vision_speed_estimate_t const &)> SpeedCamSpeedCallback
Definition: speed_cam.h:46
SpeedCamStatus
Definition: speed_cam.h:59
void ReadCallback(const uint8_t *buffer, size_t len)
Definition: speed_cam.cc:71
Definition: speed_cam.h:40
SpeedCam(SpeedCamImuCallback cb_imu, SpeedCamCameraImageCallback cb_camera_image, SpeedCamOpticalFlowCallback cb_optical_flow, SpeedCamSpeedCallback cb_speed, SpeedCamStatusCallback cb_status, SpeedCamVersionCallback cb_version, SpeedCamStateCallback)
Definition: speed_cam.cc:24
std::function< void(mavlink_raw_imu_t const &)> SpeedCamImuCallback
Definition: speed_cam.h:43
void TimeoutCallback(void)
Definition: speed_cam.cc:173
SpeedCamResult Initialize(std::string const &port, uint32_t baud)
Definition: speed_cam.cc:50
void SetState(int32_t const &state)
Definition: speed_cam.cc:59
std::function< void(mavlink_optical_flow_t const &)> SpeedCamOpticalFlowCallback
Definition: speed_cam.h:45
std::function< void(mavlink_heartbeat_t const &)> SpeedCamStatusCallback
Definition: speed_cam.h:47
@ STATUS_ANGULAR_SPEED_LIMIT_EXCEEDED
Definition: speed_cam.h:62
@ STATUS_LINEAR_SPEED_LIMIT_EXCEEDED
Definition: speed_cam.h:61
uint8_t state
Definition: signal_lights.h:90
@ RESULT_PORT_WRITE_FAILURE
Definition: speed_cam.h:55
std::function< void(uint32_t)> SpeedCamVersionCallback
Definition: speed_cam.h:48
Definition: speed_cam.h:66
@ RESULT_SUCCESS
Definition: speed_cam.h:53