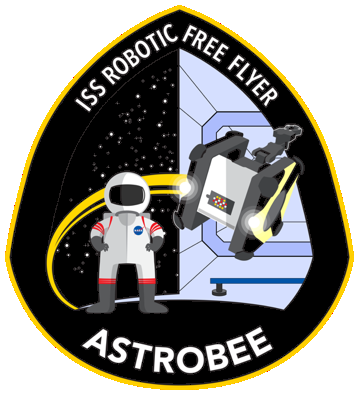 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_POLYNOMIALS_H_
20 #define MAPPER_POLYNOMIALS_H_
22 #include <pcl/point_cloud.h>
23 #include <pcl/point_types.h>
25 #include <Eigen/Dense>
31 #include "ff_msgs/ControlState.h"
32 #include "ff_msgs/Segment.h"
46 Polynomial(
const double t0,
const double tf,
const int coeff_size);
64 double *result)
const;
81 Poly3D(
const double t0_in,
const double tf_in,
82 const ff_msgs::ControlState segment);
90 void SegmentAtTime(
const double time, pcl::PointXYZ *result)
const;
110 pcl::PointXYZ *result)
116 #endif // MAPPER_POLYNOMIALS_H_
void SegmentAtTime(const double time, Eigen::Vector3d *result) const
Definition: polynomials.cc:234
Polynomial poly_z_
Definition: polynomials.h:74
Definition: polynomials.h:70
Trajectory3D(const ff_msgs::Segment segments)
Definition: polynomials.cc:254
void PolyAtTime(const double time, double *result) const
Definition: polynomials.cc:91
double tf_
Definition: polynomials.h:41
Eigen::VectorXd coeff_
Definition: polynomials.h:43
Poly3D()
Definition: polynomials.cc:213
double tf_
Definition: polynomials.h:76
void PrintTrajCoeff()
Definition: polynomials.cc:278
std::vector< Setpoint > Segment
Definition: ff_flight.h:47
std::vector< std::complex< double > > Roots3rdOrderPoly()
Definition: polynomials.cc:136
double t0_
Definition: polynomials.h:75
double tf_
Definition: polynomials.h:98
double t0_
Definition: polynomials.h:97
int order_
Definition: polynomials.h:77
int n_segments_
Definition: polynomials.h:99
void PolyConv(const Polynomial *poly2, Polynomial *poly_out)
Definition: polynomials.cc:51
Polynomial poly_y_
Definition: polynomials.h:73
Definition: polynomials.h:34
void PrintSegmentCoeff()
Definition: polynomials.cc:227
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
void PrintPolyCoeff()
Definition: polynomials.cc:43
std::vector< Poly3D > segments_poly_
Definition: polynomials.h:96
void TrajectoryAtTime(const double time, Eigen::Vector3d *result) const
Definition: polynomials.cc:288
Polynomial & operator=(const Polynomial &other)
Definition: polynomials.h:50
double t0_
Definition: polynomials.h:40
Definition: polynomials.h:38
std::vector< std::complex< double > > Roots2ndOrderPoly()
Definition: polynomials.cc:109
int order_
Definition: polynomials.h:42
void PolyDiff(Polynomial *poly_out)
Definition: polynomials.cc:82
Polynomial()
Definition: polynomials.cc:35
void PolySquare(Polynomial *poly_out)
Definition: polynomials.cc:67
Definition: polynomials.h:94
Polynomial poly_x_
Definition: polynomials.h:72