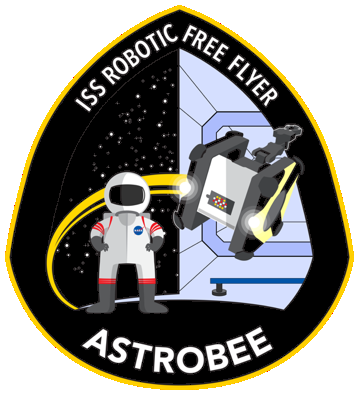 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_OCTOCLASS_H_
20 #define MAPPER_OCTOCLASS_H_
22 #include <octomap/octomap.h>
23 #include <octomap/OcTree.h>
24 #include <pcl/point_cloud.h>
25 #include <tf/transform_broadcaster.h>
26 #include <pcl/point_types.h>
27 #include <sensor_msgs/point_cloud2_iterator.h>
28 #include <visualization_msgs/MarkerArray.h>
39 octomap::OcTree
tree_ = octomap::OcTree(0.1);
45 explicit OctoClass(
const double resolution_in);
60 const double aspect_ratio);
63 const double probability_miss);
65 const double clamping_threshold_max);
72 const geometry_msgs::TransformStamped &tf_cam2world,
76 const octomap::point3d& origin,
77 const double &maxrange,
89 std::vector<octomap::point3d> *colliding_nodes);
93 visualization_msgs::MarkerArray *free,
94 sensor_msgs::PointCloud2* obstacles_cloud,
95 sensor_msgs::PointCloud2* free_cloud);
97 visualization_msgs::MarkerArray *free,
98 sensor_msgs::PointCloud2* obstacles_cloud,
99 sensor_msgs::PointCloud2* free_cloud);
109 const octomap::point3d &p2);
117 const octomap::point3d &p2);
133 std::vector<octomap::OcTreeKey> *node_keys,
134 std::vector<double> *node_sizes);
139 std::vector<double> *node_sizes);
143 const double &node_size,
144 std::vector<octomap::OcTreeKey> *neighbor_keys);
147 octomap::OcTreeNode* node);
152 double max_range_, min_range_;
153 float inflate_radius_;
154 std::vector<Eigen::Vector3d> sphere_;
155 std::vector<double> depth_volumes_;
158 double VectorNormSquared(
const double &x,
161 std_msgs::ColorRGBA HeightMapColor(
const double &height,
162 const double &alpha);
167 #endif // MAPPER_OCTOCLASS_H_
void ComputeUpdate(const octomap::KeySet &occ_inflated, const octomap::KeySet &occ_slim, const octomap::point3d &origin, const double &maxrange, octomap::KeySet *occ_slim_in_range, octomap::KeySet *free_slim, octomap::KeySet *free_inflated)
Definition: octoclass.cc:260
void BBXOccVolume(const Eigen::Vector3d &box_min, const Eigen::Vector3d &box_max, double *volume)
Definition: octoclass.cc:777
void SetMapInflation(const double inflate_radius)
Definition: octoclass.cc:90
bool CheckCollision(const octomap::point3d &p)
Definition: octoclass.cc:696
Definition: linear_algebra.h:110
double GetNodeSize(const octomap::OcTreeKey &key)
Definition: octoclass.cc:886
void SetOccupancyThreshold(const double occupancy_threshold)
Definition: octoclass.cc:129
void PclToRayOctomap(const pcl::PointCloud< pcl::PointXYZ > &cloud, const geometry_msgs::TransformStamped &tf_cam2world, const algebra_3d::FrustumPlanes &frustum)
Definition: octoclass.cc:174
void SetMemoryTime(const double memory)
Definition: octoclass.cc:38
void SetClampingThresholds(const double clamping_threshold_min, const double clamping_threshold_max)
Definition: octoclass.cc:145
void ResetMap()
Definition: octoclass.cc:123
int CheckOccupancy(const octomap::point3d &p)
Definition: octoclass.cc:641
OctoClass()
Definition: octoclass.cc:36
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
double GetMapInflation()
Definition: octoclass.cc:113
void InflatedVisMarkers(visualization_msgs::MarkerArray *obstacles, visualization_msgs::MarkerArray *free, sensor_msgs::PointCloud2 *obstacles_cloud, sensor_msgs::PointCloud2 *free_cloud)
Definition: octoclass.cc:561
Definition: octoclass.h:37
void SetMaxRange(const double max_range)
Definition: octoclass.cc:47
void GetNodeNeighbors(const octomap::OcTreeKey &node_key, const double &node_size, std::vector< octomap::OcTreeKey > *neighbor_keys)
Definition: octoclass.cc:859
std::tr1::unordered_set< IndexedOcTreeKey, IndexedOcTreeKey::KeyHash > KeySet
Definition: indexed_octree_key.h:85
void SetResolution(const double resolution_in)
Definition: octoclass.cc:57
void BBXFreeVolume(const Eigen::Vector3d &box_min, const Eigen::Vector3d &box_max, double *volume)
Definition: octoclass.cc:743
algebra_3d::FrustumPlanes cam_frustum_
Definition: octoclass.h:42
octomap::OcTree tree_inflated_
Definition: octoclass.h:40
double GetResolution() const
Definition: octoclass.h:53
void TreeVisMarkers(visualization_msgs::MarkerArray *obstacles, visualization_msgs::MarkerArray *free, sensor_msgs::PointCloud2 *obstacles_cloud, sensor_msgs::PointCloud2 *free_cloud)
Definition: octoclass.cc:479
double GetMemoryTime()
Definition: octoclass.cc:43
void SetCamFrustum(const double fov, const double aspect_ratio)
Definition: octoclass.cc:117
Definition: indexed_octree_key.h:26
void PrintQueryInfo(octomap::point3d query, octomap::OcTreeNode *node)
Definition: octoclass.cc:902
void FadeMemory(const double &rate)
Definition: octoclass.cc:299
void FindCollidingNodesInflated(const pcl::PointCloud< pcl::PointXYZ > &point_cloud, std::vector< octomap::point3d > *colliding_nodes)
Definition: octoclass.cc:427
Definition: indexed_octree_key.h:89
void BBXFreeNodes(const Eigen::Vector3d &box_min, const Eigen::Vector3d &box_max, std::vector< octomap::OcTreeKey > *node_keys, std::vector< double > *node_sizes)
Definition: octoclass.cc:812
void SetMinRange(const double min_range)
Definition: octoclass.cc:52
octomap::OcTree tree_
Definition: octoclass.h:39
void SetHitMissProbabilities(const double probability_hit, const double probability_miss)
Definition: octoclass.cc:135
double memory_time_
Definition: octoclass.h:41