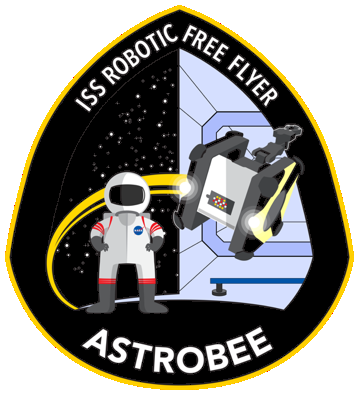 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef GRAPH_FACTORS_LOC_PROJECTION_FACTOR_H_
20 #define GRAPH_FACTORS_LOC_PROJECTION_FACTOR_H_
22 #include <gtsam/geometry/Point2.h>
23 #include <gtsam/geometry/Pose3.h>
24 #include <gtsam/geometry/SimpleCamera.h>
25 #include <gtsam/nonlinear/NonlinearFactor.h>
26 #include <boost/optional.hpp>
37 template <
class POSE = Pose3,
class LANDMARK = Po
int3,
class CALIBRATION = Cal3_S2>
43 boost::shared_ptr<CALIBRATION>
K_;
54 typedef NoiseModelFactor1<POSE>
Base;
78 Key poseKey,
const boost::shared_ptr<CALIBRATION>& K,
80 :
Base(model, poseKey),
104 Key poseKey,
const boost::shared_ptr<CALIBRATION>& K,
bool throwCheirality,
106 :
Base(model, poseKey),
118 virtual NonlinearFactor::shared_ptr
clone()
const {
119 return boost::static_pointer_cast<NonlinearFactor>(NonlinearFactor::shared_ptr(
new This(*
this)));
127 void print(
const std::string& s =
"",
const KeyFormatter& keyFormatter = DefaultKeyFormatter)
const {
128 std::cout << s <<
"LocProjectionFactor, z = ";
130 std::cout <<
" landmark_point = ";
132 if (this->body_P_sensor_) this->body_P_sensor_->print(
" sensor pose in body frame: ");
133 Base::print(
"", keyFormatter);
137 virtual bool equals(
const NonlinearFactor& p,
double tol = 1e-9)
const {
138 const This* e =
dynamic_cast<const This*
>(&p);
139 return e && Base::equals(p, tol) && traits<Point2>::Equals(this->measured_, e->
measured_, tol) &&
147 Vector
evaluateError(
const Pose3& pose, boost::optional<Matrix&> H1 = boost::none)
const {
155 return reprojectionError;
161 PinholeCamera<CALIBRATION>
camera(pose, *
K_);
164 }
catch (CheiralityException& e) {
165 if (H1) *H1 = Matrix::Zero(2, 6);
167 std::cout << e.what() <<
": Landmark moved behind camera " << DefaultKeyFormatter(this->key()) << std::endl;
170 return Vector2::Constant(0.0);
179 PinholeCamera<CALIBRATION>
camera(pose, *
K_);
182 }
catch (CheiralityException& e) {
196 inline const boost::shared_ptr<CALIBRATION>
calibration()
const {
return K_; }
209 template <
class ARCHIVE>
210 void serialize(ARCHIVE& ar,
const unsigned int ) {
211 ar& BOOST_SERIALIZATION_BASE_OBJECT_NVP(
Base);
214 ar& BOOST_SERIALIZATION_NVP(
K_);
221 EIGEN_MAKE_ALIGNED_OPERATOR_NEW
225 template <
class POSE,
class LANDMARK,
class CALIBRATION>
227 :
public Testable<LocProjectionFactor<POSE, LANDMARK, CALIBRATION>> {};
231 #endif // GRAPH_FACTORS_LOC_PROJECTION_FACTOR_H_
LANDMARK & landmark_point()
Definition: loc_projection_factor.h:193
LocProjectionFactor()
Default constructor.
Definition: loc_projection_factor.h:63
virtual ~LocProjectionFactor()
Definition: loc_projection_factor.h:115
Definition: camera_model.h:26
NoiseModelFactor1< POSE > Base
shorthand for base class type
Definition: loc_projection_factor.h:54
Definition: loc_projection_factor.h:38
bool cheiralityError(const Pose3 &pose) const
Definition: loc_projection_factor.h:173
const Point2 & measured() const
Definition: loc_projection_factor.h:189
LocProjectionFactor(const Point2 &measured, const LANDMARK &landmark_point, const SharedNoiseModel &model, Key poseKey, const boost::shared_ptr< CALIBRATION > &K, bool throwCheirality, bool verboseCheirality, boost::optional< POSE > body_P_sensor=boost::none)
Definition: loc_projection_factor.h:103
boost::optional< POSE > body_P_sensor_
The pose of the sensor in the body frame.
Definition: loc_projection_factor.h:44
boost::shared_ptr< CALIBRATION > K_
shared pointer to calibration object
Definition: loc_projection_factor.h:43
friend class boost::serialization::access
Serialization function.
Definition: loc_projection_factor.h:208
Vector evaluateError(const Pose3 &pose, boost::optional< Matrix & > H1=boost::none) const
Evaluate error h(x)-z and optionally derivatives.
Definition: loc_projection_factor.h:147
bool verboseCheirality_
Definition: loc_projection_factor.h:49
LocProjectionFactor(const Point2 &measured, const LANDMARK &landmark_point, const SharedNoiseModel &model, Key poseKey, const boost::shared_ptr< CALIBRATION > &K, boost::optional< POSE > body_P_sensor=boost::none)
Definition: loc_projection_factor.h:77
virtual bool equals(const NonlinearFactor &p, double tol=1e-9) const
equals
Definition: loc_projection_factor.h:137
boost::optional< POSE > body_P_sensor() const
Definition: loc_projection_factor.h:204
boost::shared_ptr< This > shared_ptr
shorthand for a smart pointer to a factor
Definition: loc_projection_factor.h:60
LANDMARK landmark_point_
Landmark point.
Definition: loc_projection_factor.h:42
virtual NonlinearFactor::shared_ptr clone() const
Definition: loc_projection_factor.h:118
bool throwCheirality_
Definition: loc_projection_factor.h:47
const boost::shared_ptr< CALIBRATION > calibration() const
Definition: loc_projection_factor.h:196
Definition: cumulative_factor.h:26
bool throwCheirality() const
Definition: loc_projection_factor.h:202
LocProjectionFactor< POSE, LANDMARK, CALIBRATION > This
shorthand for this class
Definition: loc_projection_factor.h:57
Point2 measured_
2D measurement
Definition: loc_projection_factor.h:41
void print(const std::string &s="", const KeyFormatter &keyFormatter=DefaultKeyFormatter) const
Definition: loc_projection_factor.h:127
bool verboseCheirality() const
Definition: loc_projection_factor.h:199
const LANDMARK & landmark_point() const
Definition: loc_projection_factor.h:191