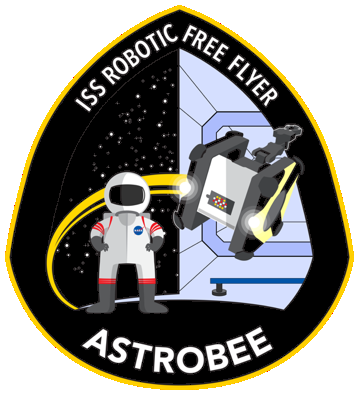 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_UTIL_FF_NODELET_H_
20 #define FF_UTIL_FF_NODELET_H_
24 #include <ros/callback_queue.h>
25 #include <nodelet/nodelet.h>
26 #include <pluginlib/class_list_macros.h>
27 #include <diagnostic_msgs/KeyValue.h>
31 #include <ff_msgs/Fault.h>
32 #include <ff_msgs/Heartbeat.h>
33 #include <ff_msgs/Trigger.h>
44 #define DEFAULT_ACTION_WAIT_TIME 30.0
45 #define DEFAULT_SERVICE_WAIT_TIME 30.0
46 #define DEFAULT_DIAGNOSTICS_RATE 1.0
49 #define FF_DEBUG(msg) NODELET_DEBUG_STREAM(msg)
50 #define FF_INFO(msg) NODELET_INFO_STREAM(msg)
51 #define FF_WARN(msg) NODELET_WARN_STREAM(msg)
52 #define FF_ERROR(msg) NODELET_ERROR_STREAM(msg)
53 #define FF_FATAL(msg) NODELET_FATAL_STREAM(msg)
72 std::string
const& message,
73 ros::Time time_fault_occurred = ros::Time::now());
99 void SendDiagnostics(
const std::vector<diagnostic_msgs::KeyValue> &keyval);
104 void Setup(ros::NodeHandle & nh, ros::NodeHandle & nh_mt, std::string plugin_name);
110 void HeartbeatCallback(ros::TimerEvent
const& ev);
113 void InitCallback(ros::TimerEvent
const& ev);
116 bool TriggerCallback(ff_msgs::Trigger::Request &req, ff_msgs::Trigger::Response &res);
119 void PublishHeartbeat();
128 bool autostart_hb_timer_;
132 unsigned int heartbeat_queue_size_;
137 ff_msgs::Heartbeat heartbeat_;
141 ros::NodeHandle nh_mt_;
142 ros::NodeHandle nh_private_;
143 ros::NodeHandle nh_private_mt_;
146 ros::Timer timer_heartbeat_;
147 ros::Timer timer_deferred_init_;
150 ros::Publisher pub_heartbeat_;
151 ros::Publisher pub_diagnostics_;
154 ros::ServiceServer srv_trigger_;
157 std::string platform_;
163 #endif // FF_UTIL_FF_NODELET_H_
FreeFlyerNodelet(bool autostart_hb_timer=true)
Definition: ff_nodelet.cc:46
std::string GetTransform(std::string const &child)
Definition: ff_nodelet.cc:434
ros::NodeHandle * GetPrivateHandle(bool multithreaded=false)
Definition: ff_nodelet.cc:419
void SendDiagnostics(const std::vector< diagnostic_msgs::KeyValue > &keyval)
Definition: ff_nodelet.cc:377
@ TRANSFORM
Definition: ff_nodelet.h:61
ros::NodeHandle * GetPlatformHandle(bool multithreaded=false)
Definition: ff_nodelet.cc:414
@ NAMESPACE
Definition: ff_nodelet.h:60
virtual ~FreeFlyerNodelet()
Definition: ff_nodelet.cc:53
std::string GetPlatform()
Definition: ff_nodelet.cc:429
std::string name
Definition: eps_simulator.cc:48
Definition: ff_nodelet.h:57
std::string GetName()
Definition: ff_nodelet.cc:424
virtual void Sleep()
Definition: ff_nodelet.h:92
Definition: config_reader.h:48
void ClearFault(FaultKeys enum_key)
Definition: ff_nodelet.cc:253
virtual void Wakeup()
Definition: ff_nodelet.h:93
void PrintFaults()
Definition: ff_nodelet.cc:284
@ RESOURCE
Definition: ff_nodelet.h:62
std::map< std::string, int > faults_
Definition: ff_nodelet.h:106
virtual void Reset()
Definition: ff_nodelet.h:91
void Setup(ros::NodeHandle &nh, ros::NodeHandle &nh_mt, std::string plugin_name)
Definition: ff_nodelet.cc:57
void StopHeartbeat()
Definition: ff_nodelet.cc:293
Definition: config_client.h:31
void ClearAllFaults()
Definition: ff_nodelet.cc:247
void AssertFault(FaultKeys enum_key, std::string const &message, ros::Time time_fault_occurred=ros::Time::now())
Definition: ff_nodelet.cc:199
virtual void Initialize(ros::NodeHandle *nh)
Definition: ff_nodelet.h:90
double Time
Definition: time.h:23
FaultKeys
Definition: ff_faults.h:28
ResolveType
Definition: ff_nodelet.h:59