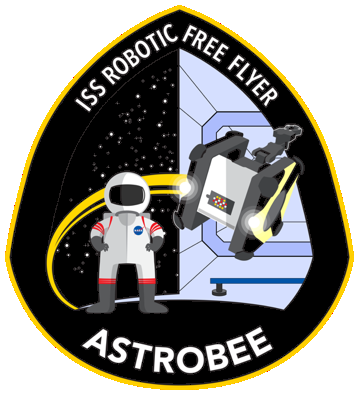 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_UTIL_FF_FLIGHT_H_
20 #define FF_UTIL_FF_FLIGHT_H_
26 #include <geometry_msgs/InertiaStamped.h>
29 #include <ff_msgs/EkfState.h>
30 #include <ff_msgs/ControlState.h>
31 #include <ff_msgs/FlightMode.h>
34 #include <Eigen/Dense>
35 #include <Eigen/Geometry>
136 ff_msgs::FlightMode &data, std::string
const&
name =
"");
149 ff_msgs::FlightMode
const& flight_mode,
bool faceforward);
164 geometry_msgs::Pose
const& b,
double tolerance_pos,
double tolerance_att);
168 geometry_msgs::Pose
const& a, geometry_msgs::Pose
const& b);
173 #endif // FF_UTIL_FF_FLIGHT_H_
@ SUCCESS
Definition: ff_flight.h:111
static bool GetInertiaConfig(geometry_msgs::InertiaStamped &data)
Definition: ff_flight.cc:123
State operator-(State const &right)
Definition: ff_flight.cc:73
@ ERROR_STATIONARY_ENDPOINT
Definition: ff_flight.h:113
@ CHECK_NONE
Definition: ff_flight.h:95
Eigen::Vector3d w
Definition: ff_flight.h:83
static double VectorMagnitude(Eigen::Vector3d const &iv)
Definition: ff_flight.cc:114
static bool WithinTolerance(geometry_msgs::Pose const &a, geometry_msgs::Pose const &b, double tolerance_pos, double tolerance_att)
Definition: ff_flight.cc:504
virtual ~State()
Definition: ff_flight.cc:61
ff_msgs::EkfState Estimate
Definition: ff_flight.h:46
@ ERROR_LIMITS_ACCEL
Definition: ff_flight.h:117
static bool GetFlightMode(ff_msgs::FlightMode &data, std::string const &name="")
Definition: ff_flight.cc:151
@ CHECK_MINIMUM_NUM_SETPOINTS
Definition: ff_flight.h:99
Setpoint ToSetpoint()
Definition: ff_flight.cc:86
@ ERROR_LIMITS_OMEGA
Definition: ff_flight.h:118
std::vector< Setpoint > Segment
Definition: ff_flight.h:47
uint8_t mask
Definition: signal_lights.h:72
@ ERROR_TIME_RUNS_BACKWARDS
Definition: ff_flight.h:115
Eigen::Vector3d p
Definition: ff_flight.h:82
static constexpr double MIN_CONTROL_RATE
Definition: ff_flight.h:129
static double ValidateUpperLimit(const double limit, double val)
Definition: ff_flight.cc:237
Eigen::Vector3d v
Definition: ff_flight.h:84
std::string name
Definition: eps_simulator.cc:48
static bool GetInitialFlightMode(ff_msgs::FlightMode &data)
Definition: ff_flight.cc:226
State()
Definition: ff_flight.cc:41
Eigen::Vector3d Vector3d(const PointType &point)
Definition: utilities.h:328
static bool Equal(Setpoint const &left, Setpoint const &right, size_t degree=2)
Definition: ff_flight.cc:466
Definition: ff_flight.h:123
@ CHECK_STATIONARY_ENDPOINT
Definition: ff_flight.h:97
double t
Definition: ff_flight.h:80
@ CHECK_MINIMUM_FREQUENCY
Definition: ff_flight.h:96
Eigen::Vector3d b
Definition: ff_flight.h:85
@ ERROR_LIMITS_VEL
Definition: ff_flight.h:116
@ CHECK_ALL
Definition: ff_flight.h:107
@ CHECK_ALL_BASIC
Definition: ff_flight.h:104
@ CHECK_LIMITS_ALPHA
Definition: ff_flight.h:103
@ ERROR_MINIMUM_FREQUENCY
Definition: ff_flight.h:112
@ ERROR_MINIMUM_NUM_SETPOINTS
Definition: ff_flight.h:114
std::map< std::string, ff_msgs::FlightMode > FlightModeMap
Definition: ff_flight.h:126
Eigen::Vector3d a
Definition: ff_flight.h:86
@ CHECK_ALL_LIMITS
Definition: ff_flight.h:105
@ CHECK_FIRST_TIMESTAMP_IN_PAST
Definition: ff_flight.h:98
static std::string GetDescription(SegmentResult result)
Definition: ff_flight.cc:441
static double ValidateLowerLimit(const double limit, double val)
Definition: ff_flight.cc:246
void operator=(State const &right)
Definition: ff_flight.cc:63
static SegmentResult Check(SegmentCheckMask mask, Segment const &segment, ff_msgs::FlightMode const &flight_mode, bool faceforward)
Definition: ff_flight.cc:255
@ CHECK_LIMITS_OMEGA
Definition: ff_flight.h:102
SegmentResult
Definition: ff_flight.h:110
@ CHECK_LIMITS_VEL
Definition: ff_flight.h:100
Definition: config_client.h:31
SegmentCheckMask
Definition: ff_flight.h:94
@ CHECK_ALL_CONSISTENCY
Definition: ff_flight.h:106
Definition: ff_flight.h:49
@ ERROR_LIMITS_ALPHA
Definition: ff_flight.h:119
static SegmentResult Resample(Segment const &in, Segment &out, double rate=-1.0)
Definition: ff_flight.cc:320
ff_msgs::ControlState Setpoint
Definition: ff_flight.h:45
double QuaternionMagnitude()
Definition: ff_flight.cc:99
Eigen::Quaterniond q
Definition: ff_flight.h:81
@ CHECK_LIMITS_ACCEL
Definition: ff_flight.h:101