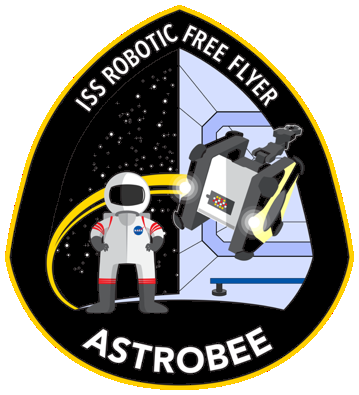 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef LOCALIZATION_MEASUREMENTS_MEASUREMENT_CONVERSIONS_H_
20 #define LOCALIZATION_MEASUREMENTS_MEASUREMENT_CONVERSIONS_H_
22 #include <ff_msgs/DepthOdometry.h>
23 #include <ff_msgs/DepthLandmarks.h>
24 #include <ff_msgs/Feature2dArray.h>
25 #include <ff_msgs/VisualLandmarks.h>
43 #include <pcl/point_cloud.h>
44 #include <pcl/point_types.h>
45 #include <pcl_conversions/pcl_conversions.h>
47 #include <sensor_msgs/Image.h>
48 #include <sensor_msgs/PointCloud2.h>
58 const TimestampedHandrailPose& world_T_handrail);
60 Plane
MakeHandrailPlane(
const gtsam::Pose3& world_T_handrail,
const double distance_to_wall);
66 const MatchedProjectionsMeasurement& matched_projections_measurement,
67 const gtsam::Pose3& new_frame_T_measurement_origin);
73 boost::optional<ImageMeasurement>
MakeImageMeasurement(
const sensor_msgs::ImageConstPtr& image_msg,
74 const std::string& encoding);
79 const sensor_msgs::PointCloud2ConstPtr& depth_cloud_msg,
const sensor_msgs::ImageConstPtr& image_msg,
83 template <
typename Po
intType>
86 sensor_msgs::PointCloud2 cloud_msg;
89 cloud_msg.header.frame_id = frame;
102 #endif // LOCALIZATION_MEASUREMENTS_MEASUREMENT_CONVERSIONS_H_
FanSpeedMode ConvertFanSpeedMode(const uint8_t speed)
Definition: measurement_conversions.cc:128
DepthCorrespondences MakeDepthCorrespondences(const std::vector< ff_msgs::DepthCorrespondence > &msgs)
HandrailPointsMeasurement MakeHandrailPointsMeasurement(const ff_msgs::DepthLandmarks &depth_landmarks, const TimestampedHandrailPose &world_T_handrail)
Definition: measurement_conversions.cc:72
sensor_msgs::PointCloud2 MakePointCloudMsg(const pcl::PointCloud< PointType > &cloud, const localization_common::Time timestamp, const std::string frame)
Definition: measurement_conversions.h:84
DepthOdometryMeasurement MakeDepthOdometryMeasurement(const ff_msgs::DepthOdometry &depth_odometry_msg)
Definition: measurement_conversions.cc:255
std::pair< gtsam::Point3, gtsam::Point3 > MakeHandrailEndpoints(const gtsam::Pose3 &world_T_handrail, const double length)
Definition: measurement_conversions.cc:60
FeaturePointsMeasurement MakeFeaturePointsMeasurement(const ff_msgs::Feature2dArray &optical_flow_tracks)
Definition: measurement_conversions.cc:111
void toROSMsg(const pcl::PointCloud< T > &pcl_cloud, sensor_msgs::PointCloud2 &cloud)
Definition: pcl_conversions.h:234
MatchedProjectionsMeasurement FrameChangeMatchedProjectionsMeasurement(const MatchedProjectionsMeasurement &matched_projections_measurement, const gtsam::Pose3 &new_frame_T_measurement_origin)
Definition: measurement_conversions.cc:100
Definition: depth_correspondences.h:25
Definition: pose_with_covariance.h:28
MatchedProjectionsMeasurement MakeMatchedProjectionsMeasurement(const ff_msgs::VisualLandmarks &visual_landmarks)
Definition: measurement_conversions.cc:33
boost::optional< ImageMeasurement > MakeImageMeasurement(const sensor_msgs::ImageConstPtr &image_msg, const std::string &encoding)
Definition: measurement_conversions.cc:143
Plane MakeHandrailPlane(const gtsam::Pose3 &world_T_handrail, const double distance_to_wall)
Definition: measurement_conversions.cc:50
Eigen::Affine3d Affine3d(const Eigen::Matrix< double, 7, 1 > &affine_vector)
Definition: utilities.cc:43
FanSpeedMode
Definition: fan_speed_mode.h:23
PointCloudMeasurement MakePointCloudMeasurement(const sensor_msgs::PointCloud2ConstPtr &point_cloud_msg)
Definition: measurement_conversions.cc:156
localization_common::PoseWithCovariance MakePoseWithCovariance(const geometry_msgs::PoseWithCovariance &msg)
Definition: measurement_conversions.cc:208
void TimeToHeader(const Time timestamp, std_msgs::Header &header)
Definition: utilities.cc:113
Odometry MakeOdometry(const ff_msgs::Odometry &msg)
Definition: measurement_conversions.cc:219
double Time
Definition: time.h:23
boost::optional< DepthImageMeasurement > MakeDepthImageMeasurement(const sensor_msgs::PointCloud2ConstPtr &depth_cloud_msg, const sensor_msgs::ImageConstPtr &image_msg, const Eigen::Affine3d image_A_depth_cam=Eigen::Affine3d::Identity())
Definition: measurement_conversions.cc:163