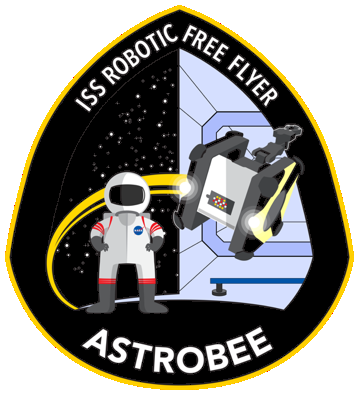 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef JSONLOADER_VALIDATION_H_
20 #define JSONLOADER_VALIDATION_H_
22 #include <json/value.h>
33 Field(std::string
const&
name, Json::ValueType type,
bool required =
true);
38 std::string
const&
name()
const noexcept;
42 Json::ValueType type_;
46 using Fields = std::vector<const Field*>;
55 bool case_sensitive =
false,
bool required =
true);
68 bool required =
true);
80 using Values = std::vector<std::string>;
83 bool required =
true);
94 static_assert(!std::is_same<std::string, T>::value,
95 "use StringField to compare strings");
97 constexpr Json::ValueType GetType() {
98 static_assert(!(std::is_integral<T>::value &&
99 std::is_floating_point<T>::value),
100 "invalid type for ValueField");
102 if (std::is_same<bool, T>::value) {
103 return Json::booleanValue;
104 }
else if (std::is_floating_point<T>::value) {
106 return Json::realValue;
107 }
else if (std::is_integral<T>::value) {
108 return Json::intValue;
114 :
Field(
name, GetType(), required), value_(value)
123 if (!obj.isMember(
name())) {
130 if (wanted != actual) {
134 if (wanted.type() == Json::uintValue &&
135 actual.type() == Json::intValue &&
136 wanted.isConvertibleTo(Json::uintValue)) {
137 return wanted.asUInt() == actual.asUInt();
156 static_assert(std::is_arithmetic<T>::value,
"Arithmetic type required");
158 constexpr Json::ValueType GetType() {
159 return (std::is_floating_point<T>::value) ?
160 Json::realValue : Json::intValue;
165 bool required =
true)
166 :
Field(
name, GetType(), required), min_(min), max_(max)
175 if (!obj.isMember(
name())) {
180 if (std::is_floating_point<T>::value) {
181 val = obj[
name()].asFloat();
183 val = obj[
name()].asInt();
186 if (val < min_ || val > max_) {
202 #endif // JSONLOADER_VALIDATION_H_
StringField(std::string const &name, std::string const &value, bool case_sensitive=false, bool required=true)
Definition: validation.cc:116
RangeField(std::string const &name, T const &min, T const &max, bool required=true)
Definition: validation.h:164
Field(std::string const &name, Json::ValueType type, bool required=true)
Definition: validation.cc:24
ObjectField(std::string const &name, Fields const &fields, bool required=true)
Definition: validation.cc:148
Definition: validation.h:52
virtual bool Validate(Json::Value const &obj) const
Definition: validation.cc:53
bool Validate(Json::Value const &obj) const override
Definition: validation.cc:153
std::string const & name() const noexcept
Definition: validation.cc:29
Definition: validation.h:65
Value
Definition: GPIO.h:42
bool Validate(Json::Value const &obj) const override
Definition: validation.h:169
bool Validate(Json::Value const &obj) const override
Definition: validation.cc:178
Definition: validation.h:155
std::vector< const Field * > Fields
Definition: validation.h:46
bool Validate(Json::Value const &obj) const override
Definition: validation.h:117
Definition: validation.h:93
std::vector< std::string > Values
Definition: validation.h:80
EnumField(std::string const &name, Values const &values, bool required=true)
Definition: validation.cc:173
ValueField(std::string const &name, T const &value, bool required=true)
Definition: validation.h:113
Definition: validation.h:78
Definition: validation.h:31
bool Validate(Json::Value const &obj) const override
Definition: validation.cc:122
bool Validate(Json::Value const &obj, Fields const &fields)
Definition: validation.cc:86