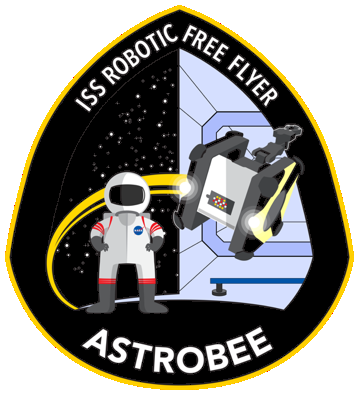 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef FF_COMMON_UTILS_H_
20 #define FF_COMMON_UTILS_H_
33 void ListFiles(std::string
const& input_dir, std::string
const& ext,
34 std::vector<std::string> * files);
39 std::string
const& before,
40 std::string
const& after);
50 template<
class T> std::vector<int>
rv_order(
const std::vector<T> &values) {
51 std::vector<int>
rv(values.size());
52 std::iota(
rv.begin(),
rv.end(), 0);
57 std::string
dirname(std::string
const& file);
58 std::string
basename(std::string
const& file);
62 void parseStr(std::string
const& str, std::vector<double> & values);
65 #endif // FF_COMMON_UTILS_H_
std::string basename(std::string const &file)
Definition: utils.cc:92
Definition: imu_bias_initializer.h:31
sorter(const std::vector< T > &v)
Definition: utils.h:47
std::string dirname(std::string const &file)
Definition: utils.cc:85
std::string ReplaceInStr(std::string const &in_str, std::string const &before, std::string const &after)
Definition: utils.cc:74
const std::vector< T > & values
Definition: utils.h:46
std::vector< int > rv_order(const std::vector< T > &values)
Definition: utils.h:50
void ListFiles(std::string const &input_dir, std::string const &ext, std::vector< std::string > *files)
Definition: utils.cc:31
bool operator()(int a, int b)
Definition: utils.h:48
std::string file_extension(std::string const &file)
Definition: utils.cc:101
void parseStr(std::string const &str, std::vector< double > &values)
Definition: utils.cc:111
void PrintProgressBar(FILE *stream, float progress)
Definition: utils.cc:57