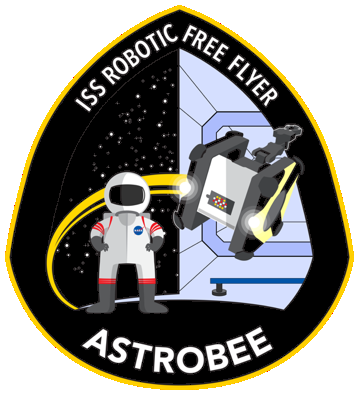 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_STRUCTS_H_
20 #define MAPPER_STRUCTS_H_
23 #include <geometry_msgs/TransformStamped.h>
24 #include <sensor_msgs/PointCloud2.h>
25 #include <pcl/point_cloud.h>
26 #include <pcl/point_types.h>
33 #include <ff_msgs/ControlGoal.h>
39 #include <condition_variable>
44 pcl::PointCloud<pcl::PointXYZ>
cloud;
64 #endif // MAPPER_STRUCTS_H_
State
Definition: structs.h:48
geometry_msgs::TransformStamped tf_body2world
Definition: structs.h:56
pcl::PointCloud< pcl::PointXYZ > cloud
Definition: structs.h:44
@ VALIDATING
Definition: structs.h:50
Definition: sampled_trajectory.h:47
geometry_msgs::TransformStamped tf_cam2world
Definition: structs.h:54
std::queue< StampedPcl > pcl_queue
Definition: structs.h:59
octoclass::OctoClass octomap
Definition: structs.h:57
@ IDLE
Definition: structs.h:49
Definition: octoclass.h:37
geometry_msgs::TransformStamped tf_cam2world
Definition: structs.h:45
geometry_msgs::TransformStamped tf_perch2world
Definition: structs.h:55
sampled_traj::SampledTrajectory3D sampled_traj
Definition: structs.h:58
Definition: mapper_nodelet.h:85