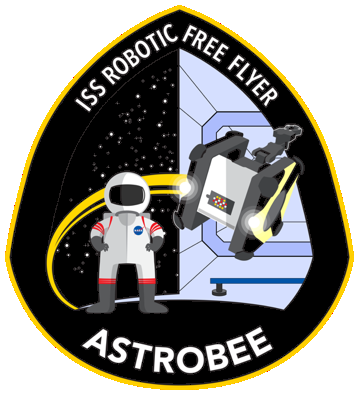 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
22 #include <Eigen/Dense>
30 static constexpr
float DT = 1.0 / 62.5;
76 float Lookup(
int table_size,
const float lookup[],
const float breakpoints[],
float value);
79 Eigen::Matrix<typename MatT::Scalar, MatT::ColsAtCompileTime, MatT::RowsAtCompileTime>
pseudoInverse(
80 const MatT& mat,
typename MatT::Scalar tolerance =
typename MatT::Scalar{std::numeric_limits<double>::epsilon()}) {
81 typedef typename MatT::Scalar Scalar;
82 auto svd = mat.jacobiSvd(Eigen::ComputeFullU | Eigen::ComputeFullV);
83 const auto& singularValues = svd.singularValues();
84 Eigen::Matrix<Scalar, MatT::ColsAtCompileTime, MatT::RowsAtCompileTime> singularValuesInv(mat.cols(), mat.rows());
85 singularValuesInv.setZero();
86 for (
unsigned int i = 0; i < singularValues.size(); ++i) {
87 if (singularValues(i) > tolerance) {
88 singularValuesInv(i, i) = Scalar {1} / singularValues(i);
90 singularValuesInv(i, i) = Scalar {0};
93 return svd.matrixV() * singularValuesInv * svd.matrixU().adjoint();
98 #endif // PMC_SHARED_H_
static const Eigen::Matrix< float, 6, 1 > discharge_coeff1
Definition: shared.h:32
static const int AREA_LOOKUP_TABLE_SIZE
Definition: shared.h:73
static constexpr float abp_nozzle_flap_length
Definition: shared.h:46
static constexpr float imp_motor_torque_k
Definition: shared.h:61
static constexpr float abp_nozzle_intake_height
Definition: shared.h:47
static const float AREA_LOOKUP_INPUT[]
Definition: shared.h:71
static constexpr float servo_motor_k
Definition: shared.h:50
Eigen::Matrix< typename MatT::Scalar, MatT::ColsAtCompileTime, MatT::RowsAtCompileTime > pseudoInverse(const MatT &mat, typename MatT::Scalar tolerance=typename MatT::Scalar{std::numeric_limits< double >::epsilon()})
Definition: shared.h:79
static const Eigen::Vector3f impeller_orientation1
Definition: shared.h:39
static const Eigen::Matrix< float, 6, 3 > nozzle_orientations2
Definition: shared.h:36
static constexpr float impeller_inertia
Definition: shared.h:69
static constexpr float abp_nozzle_gear_ratio
Definition: shared.h:48
static const Eigen::Matrix< float, 6, 3 > nozzle_orientations1
Definition: shared.h:36
static constexpr float servo_motor_friction
Definition: shared.h:54
static constexpr float DT
Definition: shared.h:30
static constexpr float imp_motor_speed_k
Definition: shared.h:59
float Lookup(int table_size, const float lookup[], const float breakpoints[], float value)
Definition: shared.cc:216
static constexpr float backlash_half_width
Definition: shared.h:64
static constexpr float abp_nozzle_min_open_angle
Definition: shared.h:45
static const float CDP_LOOKUP_OUTPUT[]
Definition: shared.h:72
static const Eigen::Vector3f impeller_orientation2
Definition: shared.h:39
static constexpr float impeller_diameter
Definition: shared.h:66
static constexpr float air_density
Definition: shared.h:67
static constexpr float servo_motor_gearbox_inertia
Definition: shared.h:56
static constexpr float abp_nozzle_max_open_angle
Definition: shared.h:43
static constexpr float imp_motor_r
Definition: shared.h:60
static constexpr float units_inches_to_meters
Definition: shared.h:31
static constexpr float servo_motor_gear_ratio
Definition: shared.h:57
static const Eigen::Matrix< float, 6, 3 > nozzle_offsets2
Definition: shared.h:34
static constexpr float zero_thrust_area[2]
Definition: shared.h:41
static const Eigen::Matrix< float, 6, 1 > discharge_coeff2
Definition: shared.h:32
static constexpr float servo_motor_r
Definition: shared.h:52
static constexpr float impeller_speed2pwm
Definition: shared.h:65
static const Eigen::Matrix< float, 6, 1 > nozzle_widths
Definition: shared.h:40
static constexpr float imp_motor_friction_coeff
Definition: shared.h:63
static const Eigen::Matrix< float, 6, 3 > nozzle_offsets1
Definition: shared.h:34