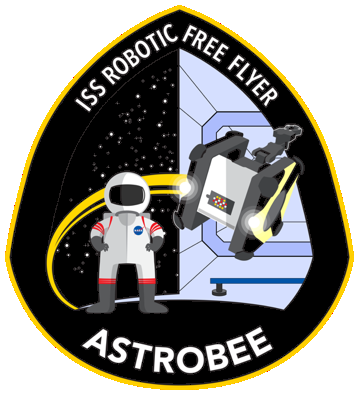 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
3 #ifndef DDS_ROS_BRIDGE_ROS_PMC_CMD_STATE_H_
4 #define DDS_ROS_BRIDGE_ROS_PMC_CMD_STATE_H_
8 #include "dds_ros_bridge/ros_sub_rapid_pub.h"
9 #include "dds_ros_bridge/util.h"
11 #include "ff_hw_msgs/PmcCommand.h"
13 #include "knDds/DdsTypedSupplier.h"
15 #include "rapidDds/RapidConstants.h"
17 #include "rapidIo/RapidIoParameters.h"
19 #include "rapidUtil/RapidHelper.h"
21 #include "dds_msgs/AstrobeeConstants.h"
22 #include "dds_msgs/PmcCmdStateSupport.h"
29 const std::string& pub_topic,
30 const ros::NodeHandle& nh,
31 const unsigned int queue_size = 10);
33 void CopyPmcGoal(
const ff_hw_msgs::PmcGoal& ros_goal, rapid::ext::astrobee::PmcGoal& dds_goal);
34 void MsgCallback(
const ff_hw_msgs::PmcCommandConstPtr& msg);
39 ff_hw_msgs::PmcCommandConstPtr pmc_msg_;
42 kn::DdsTypedSupplier<rapid::ext::astrobee::PmcCmdState>;
43 using StateSupplierPtr = std::unique_ptr<StateSupplier>;
45 StateSupplierPtr state_supplier_;
47 ros::Timer pmc_timer_;
52 #endif // DDS_ROS_BRIDGE_ROS_PMC_CMD_STATE_H_
void MsgCallback(const ff_hw_msgs::PmcCommandConstPtr &msg)
Definition: ros_pmc_cmd_state.cc:66
void PubPmcCmdState(const ros::TimerEvent &event)
Definition: ros_pmc_cmd_state.cc:71
void CopyPmcGoal(const ff_hw_msgs::PmcGoal &ros_goal, rapid::ext::astrobee::PmcGoal &dds_goal)
Definition: ros_pmc_cmd_state.cc:56
void SetPmcPublishRate(float rate)
Definition: ros_pmc_cmd_state.cc:92
Definition: ros_sub_rapid_pub.h:30
RosPmcCmdStateToRapid(const std::string &subscribe_topic, const std::string &pub_topic, const ros::NodeHandle &nh, const unsigned int queue_size=10)
Definition: ros_pmc_cmd_state.cc:24
Definition: generic_rapid_msg_ros_pub.h:36
Definition: ros_pmc_cmd_state.h:26