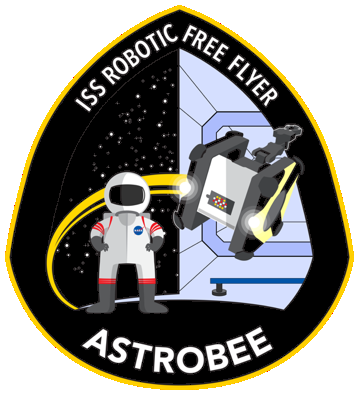 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
18 #ifndef ROS_GRAPH_VIO_ROS_GRAPH_VIO_WRAPPER_H_
19 #define ROS_GRAPH_VIO_ROS_GRAPH_VIO_WRAPPER_H_
21 #include <ff_msgs/DepthOdometry.h>
22 #include <ff_msgs/Feature2dArray.h>
23 #include <ff_msgs/FlightMode.h>
24 #include <ff_msgs/GraphVIOState.h>
32 #include <sensor_msgs/Imu.h>
46 void LoadConfigs(
const std::string& graph_config_path_prefix);
84 const std::unique_ptr<graph_vio::GraphVIO>&
graph_vio()
const;
87 std::unique_ptr<graph_vio::GraphVIO>&
graph_vio();
93 std::unique_ptr<graph_vio::GraphVIO> graph_vio_;
94 std::unique_ptr<ImuBiasInitializer> imu_bias_initializer_;
96 boost::optional<localization_common::Time> latest_msg_time_;
98 int feature_point_count_;
102 #endif // ROS_GRAPH_VIO_ROS_GRAPH_VIO_WRAPPER_H_
boost::optional< ff_msgs::GraphVIOState > GraphVIOStateMsg()
Definition: ros_graph_vio_wrapper.cc:165
void ResetVIO()
Definition: ros_graph_vio_wrapper.cc:120
Definition: imu_bias_initializer.h:31
Definition: ros_graph_vio_wrapper.h:41
void FlightModeCallback(const ff_msgs::FlightMode &flight_mode)
Definition: ros_graph_vio_wrapper.cc:108
void ResetBiasesFromFileAndResetVIO()
Definition: ros_graph_vio_wrapper.cc:159
Definition: graph_vio_params.h:34
void ResetBiasesAndVIO()
Definition: ros_graph_vio_wrapper.cc:153
void Update()
Definition: ros_graph_vio_wrapper.cc:114
void ImuCallback(const sensor_msgs::Imu &imu_msg)
Definition: ros_graph_vio_wrapper.cc:62
void FeaturePointsCallback(const ff_msgs::Feature2dArray &feature_array_msg)
Definition: ros_graph_vio_wrapper.cc:53
void DepthOdometryCallback(const ff_msgs::DepthOdometry &depth_odometry_msg)
Definition: ros_graph_vio_wrapper.cc:58
void LoadConfigs(const std::string &graph_config_path_prefix)
Definition: ros_graph_vio_wrapper.cc:40
Definition: ros_graph_vio_wrapper_params.h:22
RosGraphVIOWrapper(const std::string &graph_config_path_prefix="")
Definition: ros_graph_vio_wrapper.cc:36
const std::unique_ptr< graph_vio::GraphVIO > & graph_vio() const
Definition: ros_graph_vio_wrapper.cc:221
bool Initialized() const
Definition: ros_graph_vio_wrapper.cc:118