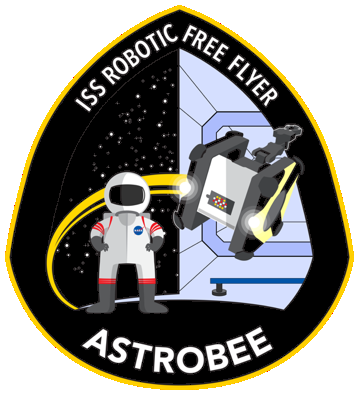 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
22 #include <Eigen/Dense>
35 void Step(
const FamInput& in, uint8_t* speed_cmd, Eigen::Matrix<float, 12, 1> & servo_pwm_cmd);
36 void UpdateCOM(
const Eigen::Vector3f & com);
39 Eigen::Matrix<float, 6, 1>& pmc1_nozzle_thrusts, Eigen::Matrix<float, 6, 1>& pmc2_nozzle_thrusts);
41 const Eigen::Matrix<float, 6, 1> & nozzle_thrusts);
42 void CalcPMServoCmd(
bool is_pmc1, uint8_t speed_gain_cmd,
const Eigen::Matrix<float, 6, 1> & nozzle_thrusts,
43 uint8_t & impeller_speed_cmd, Eigen::Matrix<float, 6, 1> & servo_pwm_cmd,
44 Eigen::Matrix<float, 6, 1> & nozzle_theta_cmd,
45 Eigen::Matrix<float, 6, 1> & normalized_pressure_density, Eigen::Matrix<float, 6, 1> & command_area_per_nozzle);
void UpdateCOM(const Eigen::Vector3f &com)
Definition: fam.cc:109
Eigen::Matrix< float, 3, 12 > thrust2torque_
Definition: fam.h:47
Eigen::Matrix< float, 12, 6 > forcetorque2thrust_
Definition: fam.h:48
static constexpr int THRUST_LOOKUP_SIZE
Definition: fam.h:53
Eigen::Matrix< float, 3, 12 > thrust2force_
Definition: fam.h:47
void CalcThrustMatrices(const Eigen::Vector3f &force, const Eigen::Vector3f &torque, Eigen::Matrix< float, 6, 1 > &pmc1_nozzle_thrusts, Eigen::Matrix< float, 6, 1 > &pmc2_nozzle_thrusts)
Definition: fam.cc:126
float ComputePlenumDeltaPressure(float impeller_speed, Eigen::Matrix< float, 6, 1 > &discharge_coeff, const Eigen::Matrix< float, 6, 1 > &nozzle_thrusts)
Definition: fam.cc:151
static const float IMPELLER_SPEEDS[]
Definition: fam.h:50
static const float THRUST_LOOKUP_BREAKPOINTS[]
Definition: fam.h:54
void CalcPMServoCmd(bool is_pmc1, uint8_t speed_gain_cmd, const Eigen::Matrix< float, 6, 1 > &nozzle_thrusts, uint8_t &impeller_speed_cmd, Eigen::Matrix< float, 6, 1 > &servo_pwm_cmd, Eigen::Matrix< float, 6, 1 > &nozzle_theta_cmd, Eigen::Matrix< float, 6, 1 > &normalized_pressure_density, Eigen::Matrix< float, 6, 1 > &command_area_per_nozzle)
Definition: fam.cc:161
void Step(const FamInput &in, uint8_t *speed_cmd, Eigen::Matrix< float, 12, 1 > &servo_pwm_cmd)
Definition: fam.cc:202
static const float THRUST_LOOKUP_CDP[]
Definition: fam.h:55