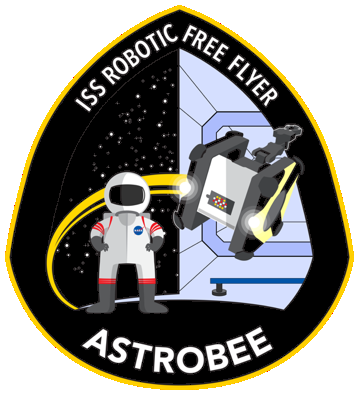 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef LOCALIZATION_MANAGER_LOCALIZATION_PIPELINE_H_
20 #define LOCALIZATION_MANAGER_LOCALIZATION_PIPELINE_H_
26 #include <ff_msgs/CameraRegistration.h>
27 #include <ff_msgs/VisualLandmarks.h>
28 #include <ff_msgs/DepthLandmarks.h>
29 #include <ff_msgs/SetBool.h>
30 #include <ff_msgs/EkfState.h>
62 : mode_(
mode), components_(0), name_(
name) {}
67 max_filter_ = max_conf;
70 timeout_filter_ = timeout;
77 topic_enable_ = topic;
78 timeout_enable_ = timeout;
86 timeout_reg_ = timeout;
92 std::string
const& topic,
double timeout, uint32_t min_features) {
94 topic_visual_ = topic;
95 timeout_visual_ = timeout;
96 min_visual_ = min_features;
102 std::string
const& topic,
double timeout, uint32_t min_features) {
104 topic_depth_ = topic;
105 timeout_depth_ = timeout;
106 min_depth_ = min_features;
112 return topic_enable_;
138 std::function<
void(
void)> cb_connected,
139 std::function<
void(
void)> cb_timeout) {
141 callback_ = cb_error;
148 service_.
Create(nh, topic_enable_);
166 sub_reg_ = nh_->subscribe(topic_reg_, 1,
168 timer_reg_ = nh_->createTimer(ros::Duration(timeout_reg_),
172 sub_visual_ = nh_->subscribe(topic_visual_, 1,
174 timer_visual_ = nh_->createTimer(ros::Duration(timeout_visual_),
178 sub_depth_ = nh_->subscribe(topic_depth_, 1,
180 timer_depth_ = nh_->createTimer(ros::Duration(timeout_depth_),
189 sub_visual_.shutdown();
190 timer_visual_.stop();
193 sub_depth_.shutdown();
203 ff_msgs::SetBool msg;
204 msg.request.enable = enable;
205 if (!service_.
Call(msg))
218 timer_filter_ = nh_->createTimer(ros::Duration(timeout_filter_),
223 sub_filter_.shutdown();
224 timer_filter_.stop();
244 if (msg->landmarks.size() < min_visual_)
246 timer_visual_.stop();
247 timer_visual_.start();
257 if (msg->landmarks.size() < min_depth_)
260 timer_depth_.start();
270 if (msg->estimating_bias)
272 else if (msg->confidence > max_filter_)
274 timer_filter_.stop();
275 timer_filter_.start();
284 uint8_t mode_, components_;
285 std::string name_, topic_enable_, topic_reg_, topic_visual_, topic_depth_;
286 double timeout_reg_, timeout_visual_, timeout_depth_, timeout_enable_, timeout_filter_;
287 ros::Subscriber sub_visual_, sub_depth_, sub_reg_, sub_filter_;
288 uint32_t min_visual_, min_depth_, max_filter_;
290 ros::NodeHandle *nh_;
291 ros::Timer timer_visual_, timer_filter_, timer_depth_, timer_reg_;
301 #endif // LOCALIZATION_MANAGER_LOCALIZATION_PIPELINE_H_
uint8_t mode
Definition: signal_lights.h:74
Pipeline & NeedsVisualFeatures(std::string const &topic, double timeout, uint32_t min_features)
Definition: localization_pipeline.h:91
@ ERROR_FILTER_TIMEOUT
Definition: localization_pipeline.h:43
Components
Definition: localization_pipeline.h:50
bool Toggle(bool enable)
Definition: localization_pipeline.h:162
Definition: localization_pipeline.h:37
void FilterTimeoutCallback(ros::TimerEvent const &event)
Definition: localization_pipeline.h:279
void SetConnectedCallback(ConnectedCallbackType cb_connected)
Definition: ff_service.h:56
Pipeline & NeedsDepthFeatures(std::string const &topic, double timeout, uint32_t min_features)
Definition: localization_pipeline.h:101
@ ERROR_REGISTRATION_TIMEOUT
Definition: localization_pipeline.h:40
std::map< std::string, Pipeline > PipelineMap
Definition: localization_pipeline.h:297
uint8_t GetMode()
Definition: localization_pipeline.h:121
@ COMPONENT_ENABLE
Definition: localization_pipeline.h:53
void RegistrationTimeoutCallback(ros::TimerEvent const &event)
Definition: localization_pipeline.h:238
Pipeline & NeedsFilter(uint32_t max_conf, bool optical_flow, double timeout)
Definition: localization_pipeline.h:65
@ COMPONENT_REGISTRATIONS
Definition: localization_pipeline.h:55
@ COMPONENT_DEPTH_FEATURES
Definition: localization_pipeline.h:57
bool Use(bool enable)
Definition: localization_pipeline.h:212
bool IsConnected()
Definition: ff_service.h:86
Definition: localization_pipeline.h:49
@ COMPONENT_VISUAL_FEATURES
Definition: localization_pipeline.h:56
@ ERROR_VISUAL_TIMEOUT
Definition: localization_pipeline.h:41
std::string name
Definition: eps_simulator.cc:48
Pipeline(uint8_t mode, std::string const &name)
Definition: localization_pipeline.h:61
std::function< void(PipelineError)> PipelineCallbackType
Definition: localization_pipeline.h:47
@ COMPONENT_FILTER
Definition: localization_pipeline.h:51
@ COMPONENT_SET_INPUT
Definition: localization_pipeline.h:54
PipelineError
Definition: localization_pipeline.h:39
Pipeline & NeedsEnable(std::string const &topic, double timeout)
Definition: localization_pipeline.h:75
bool IsConnected()
Definition: localization_pipeline.h:155
Pipeline & NeedsRegistrations(std::string const &topic, double timeout)
Definition: localization_pipeline.h:83
void SetConnectedTimeout(double to_connected)
Definition: ff_service.h:59
bool Initialize(ros::NodeHandle *nh, PipelineCallbackType cb_error, std::function< void(void)> cb_connected, std::function< void(void)> cb_timeout)
Definition: localization_pipeline.h:136
std::string const & GetTopic()
Definition: localization_pipeline.h:111
@ ERROR_DEPTH_TIMEOUT
Definition: localization_pipeline.h:42
@ ERROR_FILTER_BIAS
Definition: localization_pipeline.h:44
void RegistrationCallback(ff_msgs::CameraRegistration::ConstPtr const &msg)
Definition: localization_pipeline.h:232
bool RequiresFilter()
Definition: localization_pipeline.h:131
void DepthTimeoutCallback(ros::TimerEvent const &event)
Definition: localization_pipeline.h:264
void FilterCallback(ff_msgs::EkfState::ConstPtr const &msg)
Definition: localization_pipeline.h:269
void VisualTimeoutCallback(ros::TimerEvent const &event)
Definition: localization_pipeline.h:251
void DepthCallback(ff_msgs::DepthLandmarks::ConstPtr const &msg)
Definition: localization_pipeline.h:256
void VisualCallback(ff_msgs::VisualLandmarks::ConstPtr const &msg)
Definition: localization_pipeline.h:243
@ COMPONENT_OPTICAL_FLOW
Definition: localization_pipeline.h:52
bool RequiresOpticalFlow()
Definition: localization_pipeline.h:126
bool Call(ServiceSpec &service)
Definition: ff_service.h:94
std::string const & GetName()
Definition: localization_pipeline.h:116
#define TOPIC_GNC_EKF
Definition: ff_names.h:135
bool Create(ros::NodeHandle *nh, std::string const &topic)
Definition: ff_service.h:72
void SetTimeoutCallback(TimeoutCallbackType cb_timeout)
Definition: ff_service.h:55