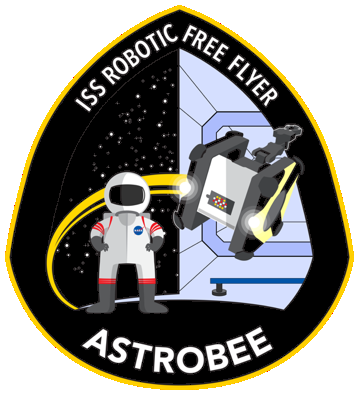 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
22 #include <ff_hw_msgs/ConfigureLED.h>
24 #include <jsoncpp/json/value.h>
30 #endif // LIGHT_FLOW_H_
91 Light(
float r,
float g,
float b);
93 explicit Light(
const std::vector<double> &d);
102 void setLight(
int index,
const std::vector<double> &d);
111 const std::vector<double> &filter);
113 const std::vector<double> &filter);
115 const std::vector<double> &filter);
117 const std::vector<double> &filter);
124 explicit Modules(
int numberOfLights);
130 void setAllBlack(std::vector<ff_hw_msgs::ConfigureLED> &ledConfigs);
134 const ros::Publisher &publishLEDGroup,
Json::Value compileListAnimation(const Json::Value &obj)
Definition: light_flow.cc:321
void printLights()
Definition: light_flow.cc:639
Definition: light_flow.h:120
std::vector< Light > lights
Definition: light_flow.h:98
Json::Value compileBasicAnimation(const Json::Value &obj)
Definition: light_flow.cc:311
std::vector< double > getPixelColorFrameInfo(const Json::Value &frameInfo, double pos, const std::vector< double > &filter)
Definition: light_flow.cc:666
Json::Value getPixelColorBasicFrame(const Json::Value &frame, double pos)
Definition: light_flow.cc:519
Json::Value getPixelColorListFrame(const Json::Value &frame, double pos)
Definition: light_flow.cc:557
ff_hw_msgs::ConfigureLED createLED(uint red, uint green, uint blue, uint position)
Definition: light_flow.cc:772
Json::Value compileDomain(const Json::Value &obj)
Definition: light_flow.cc:300
Definition: light_flow.h:87
Json::Value getPixelColorFrame(const Json::Value &frame, double pos)
Definition: light_flow.cc:571
float green
Definition: light_flow.h:89
int translateIndex(int index)
Definition: light_flow.cc:606
Json::Value macroExpand(const Json::Value ¯oLookup, Json::Value obj)
Definition: light_flow.cc:84
Json::Value compileRepeatSequence(const Json::Value &obj)
Definition: light_flow.cc:235
Json::Value compileBasicFrame(const Json::Value &obj)
Definition: light_flow.cc:125
Json::Value compileAnimation(const Json::Value &obj)
Definition: light_flow.cc:331
void applyCall(Frame &frame, double t, const Json::Value &call)
Definition: light_flow.cc:730
Json::Value getRepeatSequenceFrameInfo(const Json::Value &seq, double t, double motionOffset)
Definition: light_flow.cc:465
void applyListAnimation(Frame &frame, Json::Value animation, double t, const std::vector< double > &filter)
Definition: light_flow.cc:713
double getUnixTimestamp()
Definition: light_flow.cc:44
Definition: light_flow.h:96
Json::Value compileBasicSequence(const Json::Value &obj)
Definition: light_flow.cc:199
void compileLightFlow(Json::Value &input)
Definition: light_flow.cc:759
Frame(int numberOfLights, bool isLeft)
Definition: light_flow.cc:595
Json::Value compileInterval(const Json::Value &obj)
Definition: light_flow.cc:291
constexpr char hex[]
Definition: access_control.cc:23
Value
Definition: GPIO.h:42
Json::Value compileListFrame(const Json::Value &obj)
Definition: light_flow.cc:170
void setLight(int index, float red, float green, float blue)
Definition: light_flow.cc:598
void renderFrame(const Json::Value &statesOfExecution, Modules &modules, double timeElapsed)
Definition: light_flow.cc:739
Json::Value getSequenceFrameInfo(const Json::Value &seq, double t, double motionOffset)
Definition: light_flow.cc:490
Json::Value compileSequence(const Json::Value &obj)
Definition: light_flow.cc:266
Json::Value getProcedureLookup(const Json::Value &procedures)
Definition: light_flow.cc:383
Frame left
Definition: light_flow.h:122
void setAllBlack(std::vector< ff_hw_msgs::ConfigureLED > &ledConfigs)
Definition: light_flow.cc:764
Frame right
Definition: light_flow.h:123
bool set
Definition: light_flow.h:90
Json::Value compileRepeatFrame(const Json::Value &obj)
Definition: light_flow.cc:145
Json::Value compileReturn(const Json::Value &obj)
Definition: light_flow.cc:349
void applyBasicAnimation(Frame &frame, const Json::Value &animation, double t, const std::vector< double > &filter)
Definition: light_flow.cc:689
int hexToInt(std::string hex)
Definition: light_flow.cc:46
Json::Value compileFrame(const Json::Value &obj)
Definition: light_flow.cc:180
Json::Value compileCall(const Json::Value &obj, const Json::Value &procedureLookup)
Definition: light_flow.cc:392
Json::Value compileListSequence(const Json::Value &obj)
Definition: light_flow.cc:256
Light()
Definition: light_flow.cc:586
std::vector< double > jsonArrayToDoubleVector(const Json::Value &array)
Definition: light_flow.cc:657
Json::Value macroExpandRecursive(const Json::Value ¯oLookup, Json::Value obj)
Definition: light_flow.cc:55
Json::Value getPixelColorRepeatFrame(const Json::Value &frame, double pos)
Definition: light_flow.cc:542
Definition: light_flow.h:32
Json::Value getBasicSequenceFrameInfo(const Json::Value &seq, double t, const Json::Value &motionOffset)
Definition: light_flow.cc:425
void applyAnimation(Frame &frame, Json::Value animation, double t, const std::vector< double > &filter)
Definition: light_flow.cc:719
float blue
Definition: light_flow.h:89
uint8_t green
Definition: signal_lights.h:62
Json::Value compileCallList(const Json::Value &obj, const Json::Value &procedureLookup)
Definition: light_flow.cc:405
Json::Value compileDefine(const Json::Value &obj)
Definition: light_flow.cc:341
bool isLeft
Definition: light_flow.h:99
Json::Value compileColor(const Json::Value &rgbHexAsJson)
Definition: light_flow.cc:106
float red
Definition: light_flow.h:89
void vectorElementProduct(std::vector< double > &u, const std::vector< double > &v)
Definition: light_flow.cc:651
void publishLightFlow(const Json::Value &statesOfExecution, const ros::Publisher &publishLEDGroup, bool isStreaming)
Definition: light_flow.cc:782
Json::Value compileExecState(const Json::Value &obj, const Json::Value &procedureLookup)
Definition: light_flow.cc:413
Json::Value getMacroLookup(const Json::Value &proc)
Definition: light_flow.cc:48
Modules(int numberOfLights)
Definition: light_flow.cc:736
Json::Value getProcedureAnimation(const Json::Value &procedure)
Definition: light_flow.cc:373
Json::Value getMoveSequenceFrameInfo(const Json::Value &seq, double t, double motionOffset)
Definition: light_flow.cc:459
Json::Value compileProcedure(const Json::Value &obj)
Definition: light_flow.cc:358
void vectorAdd(Json::Value &u, const Json::Value &v)
Definition: light_flow.cc:506
uint8_t blue
Definition: signal_lights.h:63
Json::Value compileMoveSequence(const Json::Value &obj)
Definition: light_flow.cc:227
uint8_t red
Definition: signal_lights.h:61
Json::Value vectorMix(const Json::Value &u, double ku, const Json::Value &v, double kv)
Definition: light_flow.cc:511
double floatFromHex(const std::string &hex)
Definition: light_flow.cc:95
Json::Value getListSequenceFrameInfo(const Json::Value &seq, double t, double motionOffset)
Definition: light_flow.cc:481