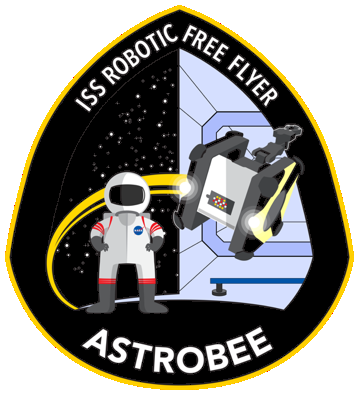 |
NASA Astrobee Robot Software
0.19.1
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef MAPPER_INDEXED_OCTREE_KEY_H_
20 #define MAPPER_INDEXED_OCTREE_KEY_H_
22 #include <octomap/octomap.h>
23 #include <octomap/OcTree.h>
24 #include <unordered_set>
49 return ((
k_[0] == other[0]) && (
k_[1] == other[1]) && (
k_[2] == other[2]));
53 return( (
k_[0] != other[0]) || (
k_[1] != other[1]) || (
k_[2] != other[2]) );
78 return static_cast<size_t>(key.
k_[0])
79 + 1447*
static_cast<size_t>(key.
k_[1])
80 + 345637*
static_cast<size_t>(key.
k_[2]);
85 typedef std::tr1::unordered_set<IndexedOcTreeKey, IndexedOcTreeKey::KeyHash>
KeySet;
94 void Insert(
const octomap::OcTreeKey &key, uint &index) {
98 bool Key2Index(
const octomap::OcTreeKey &key, uint *index_out) {
99 std::tr1::unordered_set<IndexedOcTreeKey, IndexedOcTreeKey::KeyHash>::const_iterator key2index;
101 if (key2index ==
set_.end()) {
104 *index_out = key2index->index_;
114 #endif // MAPPER_INDEXED_OCTREE_KEY_H_
Definition: indexed_octree_key.h:31
uint16_t key_type
Definition: indexed_octree_key.h:28
IndexedOcTreeKey & operator=(const IndexedOcTreeKey &other)
Definition: indexed_octree_key.h:56
key_type k_[3]
Definition: indexed_octree_key.h:69
const key_type & operator[](unsigned int i) const
Definition: indexed_octree_key.h:61
IndexedOcTreeKey()
Definition: indexed_octree_key.h:33
size_t Size()
Definition: indexed_octree_key.h:109
bool operator!=(const IndexedOcTreeKey &other) const
Definition: indexed_octree_key.h:52
uint index_
Definition: indexed_octree_key.h:70
size_t operator()(const IndexedOcTreeKey &key) const
Definition: indexed_octree_key.h:74
std::tr1::unordered_set< IndexedOcTreeKey, IndexedOcTreeKey::KeyHash > KeySet
Definition: indexed_octree_key.h:85
bool operator==(const IndexedOcTreeKey &other) const
Definition: indexed_octree_key.h:48
Definition: indexed_octree_key.h:26
IndexedOcTreeKey(const octomap::OcTreeKey &other, uint index_in)
Definition: indexed_octree_key.h:41
Definition: indexed_octree_key.h:89
void Insert(const octomap::OcTreeKey &key, uint &index)
Definition: indexed_octree_key.h:94
Provides a hash function on Keys.
Definition: indexed_octree_key.h:73
IndexedOcTreeKey(key_type a, key_type b, key_type c, uint index_in)
Definition: indexed_octree_key.h:34
KeySet set_
Definition: indexed_octree_key.h:91
bool Key2Index(const octomap::OcTreeKey &key, uint *index_out)
Definition: indexed_octree_key.h:98