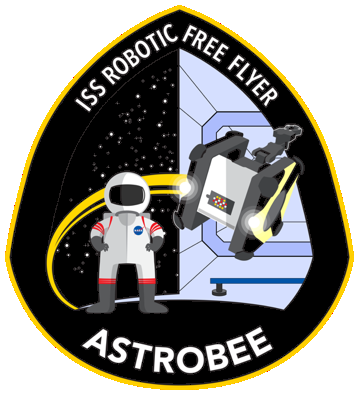 |
NASA Astrobee Robot Software
Astrobee Version:
Flight software for the Astrobee robots operating inside the International Space Station.
|
Go to the documentation of this file.
19 #ifndef COMMS_BRIDGE_GENERIC_RAPID_PUB_H_
20 #define COMMS_BRIDGE_GENERIC_RAPID_PUB_H_
27 #include "knDds/DdsTypedSupplier.h"
29 #include "rapidUtil/RapidHelper.h"
31 #include "dds_msgs/AstrobeeConstantsSupport.h"
32 #include "dds_msgs/GenericCommsAdvertisementInfoSupport.h"
33 #include "dds_msgs/GenericCommsContentSupport.h"
46 std::string
const& data_name,
47 std::string
const& topic);
51 std::string
const& data_type,
52 std::string
const& md5_sum,
53 std::string definition);
56 std::string
const& md5_sum,
58 const size_t data_size,
62 using AdvertisementInfoSupplier =
63 kn::DdsTypedSupplier<rapid::ext::astrobee::GenericCommsAdvertisementInfo>;
64 using AdvertisementInfoSupplierPtr =
65 std::unique_ptr<AdvertisementInfoSupplier>;
66 AdvertisementInfoSupplierPtr advertisement_info_supplier_;
68 using ContentSupplier =
69 kn::DdsTypedSupplier<rapid::ext::astrobee::GenericCommsContent>;
70 using ContentSupplierPtr = std::unique_ptr<ContentSupplier>;
71 ContentSupplierPtr content_supplier_;
73 unsigned int advertisement_info_seq_;
80 #endif // COMMS_BRIDGE_GENERIC_RAPID_PUB_H_
std::shared_ptr< ff::GenericRapidPub > GenericRapidPubPtr
Definition: generic_rapid_pub.h:76
~GenericRapidPub()
Definition: generic_rapid_pub.cpp:47
void SendAdvertisementInfo(std::string const &output_topic, bool latching, std::string const &data_type, std::string const &md5_sum, std::string definition)
Definition: generic_rapid_pub.cpp:66
Definition: generic_rapid_pub.h:37
Definition: generic_rapid_msg_ros_pub.h:36
void CopyString(const int max_size, std::string src, T &dest, std::string const &data_name, std::string const &topic)
Definition: generic_rapid_pub.cpp:50
void SendContent(std::string const &output_topic, std::string const &md5_sum, uint8_t const *data, const size_t data_size, const int seq_num)
Definition: generic_rapid_pub.cpp:122
GenericRapidPub(std::string const &robot_name)
Definition: generic_rapid_pub.cpp:25